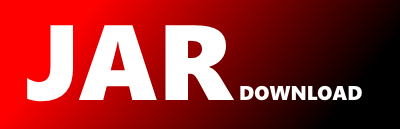
com.alachisoft.ncache.client.datastructures.DataStructureAttributes Maven / Gradle / Ivy
package com.alachisoft.ncache.client.datastructures;
import com.alachisoft.ncache.runtime.caching.expiration.Expiration;
import com.alachisoft.ncache.runtime.caching.datasource.ResyncOptions;
import com.alachisoft.ncache.runtime.CacheItemPriority;
import com.alachisoft.ncache.runtime.caching.NamedTagsDictionary;
import com.alachisoft.ncache.runtime.caching.Tag;
import com.alachisoft.ncache.runtime.dependencies.CacheDependency;
import java.util.List;
/**
* DataStructureAttributes contains the information about the DataStructures.
*/
public class DataStructureAttributes {
private Expiration _expiration = new Expiration();
private ResyncOptions _resyncOptions;
/**
* The file or cache key dependencies for the DataType.
* When any dependency changes, the object becomes invalid and is removed from
* the cache. If there are no dependencies, this property contains a null
* reference (Nothing in Visual Basic).
*/
private CacheDependency _dependency;
/**
* The Group to which the DataType is associated. It can be queryed on the basis of Groups.
*/
private String _group;
/**
* NamedTags information associated with the DataType, it can be queried on the basis of NamedTags provided.
*/
private NamedTagsDictionary _namedTags;
/**
* Tag information associated with the DataType, it can be queried on the basis of Tags provided.
*/
private List _tags;
/**
* Relative priority for DataType which is kept under consideration whenever cache starts to free up space and evicts items.
*/
private CacheItemPriority privatePriority;
/**
* Initializes DataTypeAttributes instance with default cache item priority.
*/
public DataStructureAttributes() {
setPriority(CacheItemPriority.Default);
}
/**
* Gets the expiration mechanism for DataStructure.
* @return {@link Expiration} instance that contains info about DataStructure expiration mechanism.
*/
public final Expiration getExpiration() {
return _expiration;
}
/**
* Sets the expiration mechanism for DataStructure.
* @param value Expiration object that specifies the expiration mechanism for DataStructure.
*/
public final void setExpiration(Expiration value) {
_expiration = value;
}
/**
* Gets the Cache Dependency instance that contains all dependencies associated with DataStructure.
* @return The Cache Dependency instance associated with DataStructure.
*/
public final CacheDependency getDependency() {
return _dependency;
}
/**
* Sets the Cache Dependency instance that contains all dependencies associated with DataStructure.
* @param value The Cache Dependency instance to be associated with DataStructure.
*/
public final void setDependency(CacheDependency value) {
_dependency = value;
}
/**
* Gets the associated with the DataStructure. It can be queryed on the basis of Groups.
* @return The group associated with DataStructure.
*/
public final String getGroup() {
return _group;
}
/**
* Sets the group associated with the DataStructure. It can be queryed on the basis of Groups.
* @param value The group to be associated with DataStructure.
*/
public final void setGroup(String value) {
_group = value;
}
/**
* Gets the ResyncOptions specific to the DataStructure.
* @return ResyncOptions specific to the DataStructure.
*/
public final ResyncOptions getResyncOptions() {
return _resyncOptions;
}
/**
* Sets the ResyncOptions specific to the DataStructure.
* @param value ResyncOptions specific to the DataStructure.
*/
public final void setResyncOptions(ResyncOptions value) {
_resyncOptions = value;
}
/**
* Gets the NamedTags information associated with the DataStructure, it can be queried on the basis of NamedTags provided.
* @return NamedTags associated with DataStructure.
*/
public final NamedTagsDictionary getNamedTags() {
return _namedTags;
}
/**
* Sets the NamedTags information associated with the DataStructure, it can be queried on the basis of NamedTags provided.
* @param value NamedTags to be associated with DataStructure.
*/
public final void setNamedTags(NamedTagsDictionary value) {
_namedTags = value;
}
/**
* Gets the tags information associated with the DataStructure, it can be queried on the basis of Tags provided.
* @return List of Tags associated with DataStructure.
*/
public final List getTags() {
return _tags;
}
/**
* Sets the tags information associated with the DataStructure, it can be queried on the basis of Tags provided.
* @param value List of Tags to be associated with DataStructure.
*/
public final void setTags(List value) {
_tags = value;
}
/**
* Gets the relative priority for DataStructure which is kept under consideration whenever cache starts to free up space and evicts items.
* @return CacheItemPriority associated with DataStructure.
*/
public final CacheItemPriority getPriority() {
return privatePriority;
}
/**
* Sets the relative priority for DataStructure which is kept under consideration whenever cache starts to free up space and evicts items.
* @param value CacheItemPriority to be associated with DataStructure.
*/
public final void setPriority(CacheItemPriority value) {
privatePriority = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy