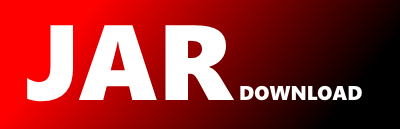
com.alachisoft.ncache.client.datastructures.DataStructureManager Maven / Gradle / Ivy
package com.alachisoft.ncache.client.datastructures;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import com.alachisoft.ncache.runtime.caching.ReadThruOptions;
import com.alachisoft.ncache.runtime.caching.WriteThruOptions;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import java.io.IOException;
/**
* This interface contains create and get operations for the {@link Counter} and remove operation for all distributed data structures.
*/
public interface DataStructureManager extends CollectionManager {
/**
* Creates the counter against the provided name.
* @param key is unqiue identifier for counter object.Initial value of counter is zero.
* @return Interface for using counters.
* @throws CacheException
* @throws IllegalArgumentException
*/
Counter createCounter(String key)throws CacheException,IllegalArgumentException;
/**
* Creates the counter against the provided name.
* @param key is unqiue identifier for counter object.
* @param initialValue is starting index of counter object.
* @return Interface for using counters.
* @throws CacheException
* @throws IllegalArgumentException
*/
Counter createCounter(String key, long initialValue)throws CacheException,IllegalArgumentException;
/**
* Creates the counter against the provided name and configures it according to the provided user configuration as attributes.
* @param name is unqiue identifier for counter object.
* @param attributes {@link DataStructureAttributes} for providing user configuration for this collection.
* @param initialValue is starting index of counter object
* @param options {@link WriteThruOptions} regarding updating data source. This can be WriteThru, WriteBehind or None.
* @return Interface for using counters.
* @throws CacheException
* @throws IllegalArgumentException
*/
Counter createCounter(String name, DataStructureAttributes attributes, long initialValue, WriteThruOptions options)throws CacheException,IllegalArgumentException;
/**
* Remove the specified data structure.
* @param key Name of the data type.
* @throws CacheException
* @throws IOException
*/
void remove(String key) throws CacheException, IOException;
/**
* Remove the specified data structure.
* @param key Name of the data type.
* @param writeThruOptions {@link WriteThruOptions} regarding updating data source. This can be WriteThru, WriteBehind or None.
* @throws CacheException
* @throws IOException
*/
void remove(String key, WriteThruOptions writeThruOptions) throws CacheException, IOException;
/**
* Gets Counter interface against the provided name.
* @param key Name of counter.
* @return Interface for using counters.
* @throws CacheException
* @throws IllegalArgumentException
*/
Counter getCounter(String key)throws CacheException,IllegalArgumentException;
/**
* Gets Counter interface against the provided name with backing source Read Thru option.
* @param key Name of counter.
* @param options {@link ReadThruOptions} to read from data source. These can be either ReadThru, ReadThruForced or None.
* @return Interface for using counters.
* @throws CacheException
* @throws IllegalArgumentException
*/
Counter getCounter(String key, ReadThruOptions options)throws CacheException,IllegalArgumentException;
/**
* @hidden
* @param cache
* @throws CacheException
* @throws IllegalArgumentException
*/
void setCache(CacheImpl cache)throws CacheException,IllegalArgumentException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy