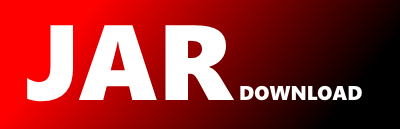
com.alachisoft.ncache.client.datastructures.DistributedHashSet Maven / Gradle / Ivy
package com.alachisoft.ncache.client.datastructures;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import java.util.Collection;
import java.util.Set;
/**
* This interface contains methods and parameters for distributed HashSet.
* @param The type of the elements in the HashSet.
*/
public interface DistributedHashSet extends Set, DistributedDataStructure, Notifiable {
/**
* Insert elements of the provided collection in DistributedHashSet.
* @param collection Elements to be inserted in the DistributedHashSet.
*/
void addRange(Collection collection) ;
/**
* Copies the HashSet elements to the specified array, starting at the specified index.
* @param array The destination array of the elements copied from Hashset.
* @param arrayIndex he zero-based index in array at which copying begins.
*/
void copyTo(T[] array, int arrayIndex);
/**
* Removes and returns a random element from the set.
* @return Random element from set.
*/
T removeRandom() ;
/**
* Return a random element from the set.
* @return Random element from set.
*/
T getRandom();
/**
* Returns count distinct random elements from the set.
* @param count Number of required elements.
* @return Count distinct elements from set.
*/
T[] getRandom(int count) ;
/**
* Remove the specified items from the set.
* @param items Items to remove from the set.
* @return The number of members that were removed from the set.
*/
int remove(Collection items) ;
/**
* Returns the union of current set with the specified set.
* @param other Name of set to compare with.
* @return Union of current set with the specified set.
* @throws CacheException
*/
Collection union(String other) throws CacheException;
/**
* Returns the intersection of current set with the specified set.
* @param other Name of set to compare with.
* @return Intersection of current set with the specified set.
* @throws CacheException
*/
Collection intersect(String other) throws CacheException;
/**
* Returns the difference of current set with the specified set.
* @param other Name of set to compare with.
* @return Difference of current set with the specified set.
* @throws CacheException
*/
Collection difference(String other) throws CacheException;
/**
* Take union of current set with the specified set and store the result in a new destination set.
* @param destination Name of destination set.
* @param other Name of set to compare with.
* @return Interface of destination set handler.
* @throws CacheException
*/
DistributedHashSet storeUnion(String destination, String other) throws CacheException;
/**
* Take difference of current set with the specified set and store the result in a new destination set.
* @param destination Name of destination set.
* @param other Name of set to compare with.
* @return Interface of destination set handler.
* @throws CacheException
*/
DistributedHashSet storeDifference(String destination, String other) throws CacheException;
/**
* Take intersection of current set with the specified set and store the result in a new destination set.
* @param destination Name of destination set.
* @param other Name of set to compare with.
* @return Interface of destination set handler.
* @throws CacheException
*/
DistributedHashSet storeIntersection(String destination, String other) throws CacheException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy