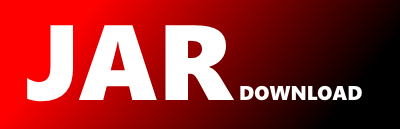
com.alachisoft.ncache.client.datastructures.DistributedList Maven / Gradle / Ivy
package com.alachisoft.ncache.client.datastructures;
import java.util.Collection;
import java.util.List;
/**
* This interface contains methods and parameters for distributed List.
* @param The type of elements in the list.
*/
public interface DistributedList extends List, DistributedDataStructure, Notifiable {
/**
* Trim an existing list so that it will contain only the specified range of elements.
* @param start Starting index.
* @param end Ending index.
*/
void trim(int start, int end);
/**
* Copies the List elements to the specified array, starting at the specified index.
* @param array The destination array of the elements copied from List.
* @param arrayIndex he zero-based index in array at which copying begins.
*/
void copyTo(T[] array, int arrayIndex);
/**
* Returns a list that will contain only the specified range of elements.
* @param start Starting index.
* @param count Number of items.
*/
Collection getRange(int start, int count) ;
/**
* Adds the elements of the specified collection to the end of the List.
* @param items The collection whose elements should be added to the end of the List.
*/
void addRange(Collection extends T> items) ;
/**
* Removes a range of elements from the List.
* @param index The zero-based starting index of the range of elements to remove.
* @param count The number of elements to remove.
*/
void removeRange(int index, int count) ;
/**
* Removes the elements of the specified collection from the List.
* @param items The collection whose elements should be removed from the List.
* @return The number of removed elements.
*/
int removeRange(Collection> items);
/**
* Inserts the element in the list after the first occurrence of specified element.
* @param pivot Element after which value will be inserted.
* @param value Element to insert in the list.
* @return False if the value pivot was not found; else true.
*/
boolean insertAfter(T pivot, T value);
/**
* Inserts the element in the list before the first occurrence of specified element.
* @param pivot Element before which value will be inserted.
* @param value Element to insert in the list.
* @return False if the value pivot was not found; else true.
*/
boolean insertBefore(T pivot, T value);
/**
* Returns the first element of the list.
* @return The first element in the list.
*/
T first();
/**
* Returns the last element of the list.
* @return The last element in the list.
*/
T last();
/**
* Insert the specified value at the head of the list.
* @param value Element to insert in the list.
*/
void insertAtHead(T value);
/**
* Insert the specified value at the tail of the list.
* @param value Element to insert in the list.
*/
void insertAtTail(T value) ;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy