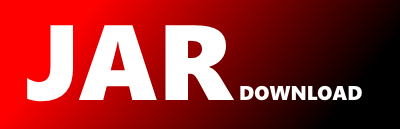
com.alachisoft.ncache.client.internal.caching.CacheDataReader Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.caching;
import Alachisoft.NCache.Caching.CompressedValueEntry;
import Alachisoft.NCache.Common.DataReader.ColumnCollection;
import Alachisoft.NCache.Common.DataReader.RecordSetEnumerator;
import Alachisoft.NCache.Common.DataReader.RecordRow;
import Alachisoft.NCache.Common.Queries.AverageResult;
import Alachisoft.NCache.Common.Queries.QueryKeyWords;
import com.alachisoft.ncache.client.CacheReader;
import Alachisoft.NCache.Common.ErrorHandling.ErrorCodes;
import Alachisoft.NCache.Common.ErrorHandling.ErrorMessages;
import com.alachisoft.ncache.runtime.exceptions.OperationFailedException;
import com.alachisoft.ncache.runtime.JSON.JsonUtil;
import java.io.IOException;
class CacheDataReader extends DataReaderCommon implements CacheReader
{
private RecordSetEnumerator _recordSetEnumerator;
private RecordRow _currentRow;
private ColumnCollection _columns;
private int _hiddenColumnCount = 0;
public CacheDataReader(RecordSetEnumerator recordSetEnumerator)
{
this(null, recordSetEnumerator);
}
public CacheDataReader(CacheImpl cache, RecordSetEnumerator recordSetEnumerator)
{
_cache = cache;
_recordSetEnumerator = recordSetEnumerator;
_columns = _recordSetEnumerator != null ? _recordSetEnumerator.getColumnCollection() : null;
if (_columns != null)
{
_hiddenColumnCount = _columns.getHiddenColumnCount();
}
}
/**
Gets number of visible columns in RecordSet
*/
public int getFieldCount()
{
return _columns == null ? 0 : _columns.getCount() - _hiddenColumnCount;
}
public void close() throws Exception
{
if (_recordSetEnumerator != null)
{
_recordSetEnumerator.close();
}
_currentRow = null;
_columns = null;
}
public boolean read()
{
if (_recordSetEnumerator == null)
{
return false;
}
boolean next = _recordSetEnumerator.moveNext();
if (next)
{
_currentRow = _recordSetEnumerator.getCurrent();
_columns=_currentRow.getColumns();
}
return next;
}
public boolean getBoolean(int index) throws OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
return (boolean)(_currentRow.get(index));
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public String getString(int index) throws OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
Object obj = _currentRow.get(index);
if (obj instanceof AverageResult)
{
return JsonUtil.getValueAs(((AverageResult)obj).getAverage(), String.class);
}
return obj == null ? null: obj.toString();
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public java.math.BigDecimal getBigDecimal(int index) throws OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
Object obj = _currentRow.get(index);
if (obj instanceof AverageResult)
{
return JsonUtil.getValueAs(((AverageResult)obj).getAverage(),java.math.BigDecimal.class);
}
return (java.math.BigDecimal)obj;
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public double getDouble(int index) throws OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
Object obj = _currentRow.get(index);
if (obj instanceof AverageResult)
{
return JsonUtil.getValueAs(((AverageResult)obj).getAverage(),Double.class);
}
return (Double)obj;
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public short getShort(int index) throws OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
Object obj = _currentRow.get(index);
if (obj instanceof AverageResult)
{
return JsonUtil.getValueAs(((AverageResult)obj).getAverage(), Short.class);
}
return (Short)obj;
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public int getInt(int index) throws OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
Object obj = _currentRow.get(index);
if (obj instanceof AverageResult)
{
return JsonUtil.getValueAs(((AverageResult)obj).getAverage(),Integer.class);
}
return (Integer)obj;
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public long getLong(int index) throws OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
Object obj = _currentRow.get(index);
if (obj instanceof AverageResult)
{
return JsonUtil.getValueAs(((AverageResult)obj).getAverage(),Long.class);
}
return (Long)obj;
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public T getValue(int index, Class> cls) throws OperationFailedException, IOException, ClassNotFoundException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
Object obj = _currentRow.getColumnValue(index);
if (!(_currentRow.getColumns().get(index).getIsHidden()))
{
CompressedValueEntry cmpEntry = (CompressedValueEntry)((obj instanceof CompressedValueEntry) ? obj : null);
if (cmpEntry != null)
{
obj = extractFromCompressedValueEntry(cmpEntry,cls );
}
if (obj instanceof AverageResult)
{
return JsonUtil.getValueAs(((AverageResult)obj).getAverage(), cls);
}
else
{
return JsonUtil.getValueAs(obj,cls);
}
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public T getValue(String columnName, Class> cls) throws IOException, ClassNotFoundException, OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (_currentRow.getColumns().contains(columnName))
{
Object obj = _currentRow.getColumnValue(columnName);
if (!_currentRow.getColumns().get(columnName).getIsHidden())
{
CompressedValueEntry cmpEntry = (CompressedValueEntry)((obj instanceof CompressedValueEntry) ? obj : null);
if (cmpEntry != null)
{
obj = extractFromCompressedValueEntry(cmpEntry, cls);
}
}
if (obj instanceof AverageResult)
{
return JsonUtil.getValueAs(((AverageResult)obj).getAverage(), cls);
}
else
{
return JsonUtil.getValueAs(obj,cls);
}
}
else
{
throw new IllegalArgumentException("Invalid columnName. Specified column does not exist in RecordSet.");
}
}
public int getValues(Object[] objects, Class> cls) throws IOException, ClassNotFoundException, OperationFailedException {
if (objects == null)
{
throw new IllegalArgumentException("objects");
}
if (objects.length == 0)
{
throw new IllegalArgumentException("Index was outside the bounds of the array.");
}
if (objects.length < this.getFieldCount())
{
for (int i = 0; i < objects.length; i++)
{
objects[i] = this.getSingleValue(i,cls );
}
return objects.length;
}
else
{
for (int i = 0; i < this.getFieldCount(); i++)
{
objects[i] = this.getSingleValue(i,cls );
}
return this.getFieldCount();
}
}
private T getSingleValue(int index, Class> cls) throws IOException, ClassNotFoundException,
OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
Object obj = _currentRow.getColumnValue(index);
if (!_currentRow.getColumns().get(index).getIsHidden())
{
CompressedValueEntry cmpEntry = (CompressedValueEntry)((obj instanceof CompressedValueEntry) ? obj : null);
if (cmpEntry != null)
{
obj = extractFromCompressedValueEntry(cmpEntry,cls );
}
if (obj instanceof AverageResult)
{
return (T) ((AverageResult)obj).getAverage();
}
else
{
return (T) obj;
}
}
return null;
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public String getName(int columnIndex)
{
if (_columns == null)
{
return null;
}
if (columnIndex >= 0 && columnIndex < getFieldCount())
{
if (_columns.get(columnIndex).getIsHidden())
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
return _columns.getColumnName(columnIndex);
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
public int getOrdinal(String columnName)
{
if (_columns == null)
{
return -1;
}
if (_columns.contains(columnName))
{
if (_columns.get(columnName).getIsHidden())
{
throw new IllegalArgumentException("Invalid columnName. Specified column does not exist in RecordSet.");
}
return _columns.getColumnIndex(columnName);
}
else
{
throw new IllegalArgumentException("Invalid columnName. Specified column does not exist in RecordSet.");
}
}
public boolean getIsClosed()
{
return _recordSetEnumerator == null;
}
public java.util.Date getDate(int index) throws OperationFailedException {
if (_currentRow == null)
{
throw new OperationFailedException(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED, ErrorMessages.getErrorMessage(ErrorCodes.Queries.CURRENT_STATE_NOT_SUPPORTED));
}
if (index >= 0 && index < getFieldCount())
{
Object obj = _currentRow.getColumnValue(index);
return (java.util.Date)obj;
}
else
{
throw new IllegalArgumentException("Invalid index. Specified index does not exist in RecordSet.");
}
}
@Override
protected boolean tryGetKeyFromCurrentRow(tangible.RefObject key)
{
Object possiblyKey = _currentRow.get(QueryKeyWords.KeyColumn);
key.argvalue = possiblyKey == null ? null : possiblyKey.toString();
return key.argvalue != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy