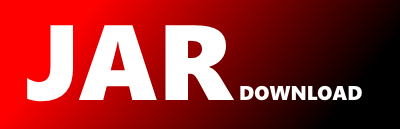
com.alachisoft.ncache.client.internal.caching.CacheHelper Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client.internal.caching;
import Alachisoft.NCache.Caching.CompressedValueEntry;
import Alachisoft.NCache.Common.EncryptionUtil;
import com.alachisoft.ncache.client.CacheItem;
import com.alachisoft.ncache.client.Credentials;
import com.alachisoft.ncache.client.internal.datastructure.DataTypeCreator;
import com.alachisoft.ncache.client.internal.util.ConfigReader;
import com.alachisoft.ncache.common.caching.EntryType;
import com.alachisoft.ncache.runtime.caching.Tag;
import com.alachisoft.ncache.runtime.exceptions.ConfigurationException;
import com.alachisoft.ncache.runtime.exceptions.OperationFailedException;
import com.alachisoft.ncache.runtime.util.NCDateTime;
import com.alachisoft.ncache.runtime.util.TimeSpan;
import com.alachisoft.ncache.security.encryption.EncryptionMgr;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
public class CacheHelper {
public static String _clientCacheID = "";
public static byte[] EncryptData(byte[] data, String cacheName) {
byte[] bite = EncryptionMgr.Encryption(data, cacheName);
return bite;
}
public static byte[] DecryptData(byte[] data, String cacheName) {
byte[] bite = EncryptionMgr.Decrypt(data, cacheName);
return bite;
}
public static T getObjectOrInitializedCollection(String key, EntryType entryType, Object obj, CacheImpl cache,Class> cls) throws OperationFailedException {
switch (entryType) {
case CacheItem:
return (T) obj;
default:
return (T) DataTypeCreator.createDataTypeHandle(entryType, key, cache,cls);
}
}
public static T getSafeValue(Object value) {
return (T) ((value != null) ? value : null);
}
public static Object getObjectOrDataTypeForCacheItem(String key, EntryType entryType, Object obj) {
if (obj.getClass() == ValueEmissary.class) {
return obj;
}
ValueEmissary valueEmissary = new ValueEmissary();
valueEmissary.setKey(key);
valueEmissary.setData(obj);
valueEmissary.setType(entryType);
return valueEmissary;
}
public static byte EvaluateExpirationParameters(java.util.Date absoluteExpiration, TimeSpan slidingExpiration)
{
if (CacheImpl.NoAbsoluteExpiration.equals(absoluteExpiration) && CacheImpl.NoSlidingExpiration.equals(slidingExpiration))
{
return 2;
}
if (CacheImpl.NoAbsoluteExpiration.equals(absoluteExpiration))
{
if (slidingExpiration != CacheImpl.DefaultSliding && slidingExpiration != CacheImpl.DefaultSlidingLonger)
{
if (slidingExpiration.compareTo(TimeSpan.Zero) < 0)
{
throw new IllegalArgumentException("slidingExpiration");
}
}
return 0;
}
if (CacheImpl.NoSlidingExpiration.equals(slidingExpiration))
{
return 1;
}
throw new IllegalArgumentException("You cannot set both sliding and absolute expirations on the same cache item.");
}
public static ArrayList LoadCredentialsFromConfig(String cacheId) throws ConfigurationException {
ArrayList paramsList = new ArrayList();
paramsList.add(new Credentials("", ""));
paramsList.add(new Credentials("", ""));
try {
HashMap tbl = ConfigReader.ReadSecurityParams("client.ncconf", cacheId);
if (tbl != null) {
HashMap primary_user = (HashMap) tbl.get("pri-user");
HashMap secondary_user = (HashMap) tbl.get("sec-user");
if (primary_user != null && primary_user.containsKey("user-id") && primary_user.containsKey("password")) {
String pri_password = (String) primary_user.get("password");
if (!pri_password.equals("")) {
paramsList.set(0, new Credentials((String) primary_user.get("user-id"), EncryptionUtil.Decrypt(Alachisoft.NCache.Common.Base64.decode(pri_password))));
}
}
if (secondary_user != null && secondary_user.containsKey("user-id") && secondary_user.containsKey("password")) {
String sec_password = (String) secondary_user.get("password");
if (!sec_password.equals("")) {
paramsList.set(1, new Credentials((String) secondary_user.get("user-id"), EncryptionUtil.Decrypt(Alachisoft.NCache.Common.Base64.decode(sec_password))));
}
}
}
return paramsList;
} catch (ConfigurationException e) {
throw new ConfigurationException("An error occured while reading client.ncconf. " + e.getMessage());
}
}
public static Map bulkGetObjectOrInitializedCollection(Map returnItems, CacheImpl parent) throws OperationFailedException {
Map updatedMap= new HashMap();
for (Object object : returnItems.entrySet())
{
Map.Entry entry = (Map.Entry)object;
CompressedValueEntry cmpEntry = (CompressedValueEntry)entry.getValue();
cmpEntry.setValue(getObjectOrInitializedCollection(entry.getKey().toString(), cmpEntry.getType(), cmpEntry.getValue(), parent,null));
updatedMap.put(entry.getKey(), entry.getValue());
}
return updatedMap;
}
public static String[] getTags(Tag[] tags)
{
String[] sTags = new String[tags.length];
for (int i = 0; i < tags.length; i++)
{
sTags[i] = tags[i].getTagName();
}
return sTags;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy