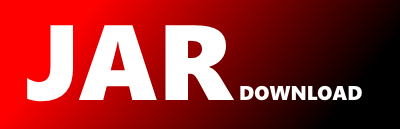
com.alachisoft.ncache.client.internal.caching.CacheStreamImpl Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client.internal.caching;
import com.alachisoft.ncache.client.CacheStream;
import com.alachisoft.ncache.client.StreamMode;
import com.alachisoft.ncache.runtime.exceptions.*;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
/**
* CacheStreamImpl is derived from System.IO.Stream. It is designed to put/fetch BLOB using standard
* Stream interface.
*/
final class CacheStreamImpl implements CacheStream {
private StreamMode _mode;
private CacheStream cacheStream;
void setCacheStream(CacheStream cacheStream) {
if (cacheStream != null) {
this.cacheStream = cacheStream;
_mode = cacheStream instanceof OutputCacheStream ? StreamMode.Write : StreamMode.Read;
}
}
void setMode(StreamMode mode)
{
_mode = mode;
}
/**
* Gets java.io.BufferedInputStream of given buffer size. CacheStreamImpl does not buffer the data. Each read/write operation performed on the stream is
* propagated to cache. However this can cause performance issues if small chunks of data are being
* read/written from/to stream. java.io.BufferedInputStream supports buffering of data. This method
* returns an instance of java.io.BufferedInputStream which encapsulates CacheStreamImpl.
*
* @param bufferSize size of of buffer stream
* @return An instance ofjava.io.BufferedInputStream of specified buffer size.
* @throws Exception
*/
@Override
public BufferedInputStream getBufferedInputStream(int bufferSize) throws Exception {
if (_mode == StreamMode.Write) {
throw new NotSupportedException("Stream does not support reading.");
}
if (bufferSize > 0)
return new BufferedInputStream((InputStream) cacheStream, bufferSize);
else
throw new IllegalArgumentException("buffer size should be greater than zero");
}
/**
* Gets java.io.BufferedOutputStream of given buffer size. CacheStreamImpl does not buffer the data. Each read/write operation performed on the stream is
* propagated to cache. However this can cause performance issues if small chunks of data are being
* read/written from/to stream. java.io.BufferedOutputStream supports buffering of data. This method
* returns an instance of java.io.BufferedOutputStream which encapsulates CacheStreamImpl.
*
* @param bufferSize size of of buffer stream
* @return An instance ofjava.io.BufferedOutputStream of specified buffer size.
* @throws Exception
*/
@Override
public BufferedOutputStream getBufferedOutputStream(int bufferSize) throws Exception {
if (_mode != StreamMode.Write) {
throw new NotSupportedException("Stream does not support writing.");
}
if (bufferSize > 0)
return new BufferedOutputStream((OutputStream) cacheStream, bufferSize);
else
throw new IllegalArgumentException("buffer size should be greater than zero");
}
/**
* Gets java.io.BufferedInputStream of given buffer size. CacheStreamImpl does not buffer the data. Each read/write operation performed on the stream is
* propagated to cache. However this can cause performance issues if small chunks of data are being
* read/written from/to stream. java.io.BufferedInputStream supports buffering of data. This method
* returns an instance of java.io.BufferedInputStream which encapsulates CacheStreamImpl.
*
* @return An instance ofjava.io.BufferedInputStream of 4kb.
* @throws Exception
*/
@Override
public BufferedInputStream getBufferedInputStream() throws Exception {
if (_mode == StreamMode.Write) {
throw new NotSupportedException("Stream does not support reading.");
}
return new BufferedInputStream((InputStream) cacheStream, 4096);
}
/**
* Gets java.io.BufferedOutputStream of given buffer size. CacheStreamImpl does not buffer the data. Each read/write operation performed on the stream is
* propagated to cache. However this can cause performance issues if small chunks of data are being
* read/written from/to stream. java.io.BufferedOutputStream supports buffering of data. This method
* returns an instance of java.io.BufferedOutputStream which encapsulates CacheStreamImpl.
*
* @return An instance ofjava.io.BufferedOutputStream of specified buffer size.
* @throws Exception
*/
@Override
public BufferedOutputStream getBufferedOutputStream() throws Exception {
if (_mode != StreamMode.Write) {
throw new NotSupportedException("Stream does not support writing.");
}
return new BufferedOutputStream((OutputStream) cacheStream, 4096);
}
/**
* Gets the length of the stream.
*
* @return length of current stream
* @throws Exception
*/
@Override
public long length()
throws Exception {
if (cacheStream.closed()) throw new IllegalAccessException("Methods were called after the stream was closed.");
return cacheStream.length();
}
/**
* Check whether stream supports reading.
*
* @return True' if stream is opened with either StreamMode.Read or StreamMode.ReadWithoutLock.
*/
@Override
public boolean canRead() {
return _mode != StreamMode.Write ? true : false;
}
/**
* Gets a value indicating whether the current stream supports seeking.
*
* @return Alwasy returns 'False' because CacheStreamImpl does not support seek operation.
*/
@Override
public boolean canSeek() {
return false;
}
/**
* Gets a value indicating whether the current stream supports writing.
*
* @return Returns 'True' if stream is opened with StreamMode.Write
*/
@Override
public boolean canWrite() {
return _mode == StreamMode.Write ? true : false;
}
/**
* Gets a value indicating whether stream is closed.
*
* @return Returns 'True' if stream is closed
*/
@Override
public boolean closed() {
return cacheStream.closed();
}
@Override
public void flush() {
}
/**
* Gets the position within current stream.
*
* @return Always return 'False' as Stream does not support seeking
* @throws Exception
*/
@Override
public long position() throws Exception {
if (!canSeek())
throw new NotSupportedException("Operation not supported");
return 0;
}
/**
* Reads a sequence of bytes from the current stream and advances the position within the stream by the number of bytes read.
*
* @param buffer An array of bytes. When this method returns, the buffer contains the specified
* byte array with the values between offset and (offset + count - 1) replaced by the bytes read from the current source.
* @param offset The zero-based byte offset in buffer at which to begin storing the data read from the current stream.
* @param count The maximum number of bytes to be read from the current stream.
* @return The total number of bytes read into the buffer. This can be less than the number of bytes requested if that many bytes are not currently available or zero (0) if the end of the stream has been reached.
* @throws Exception
*/
@Override
public int read(byte[] buffer, int offset, int count) throws Exception {
if (_mode == StreamMode.Write)
throw new NotSupportedException("Stream does not support reading.");
int bytesRead = ((InputCacheStream) cacheStream).read(buffer, offset, count);
return bytesRead;
}
/**
* Sets the position within the current stream.
*
* @param offset A value of type SeekOrigin indicating the reference point used to obtain the new position.
* @return A value of type SeekOrigin indicating the reference point used to obtain the new position.
* @throws NotSupportedException Stream does not support seeking
*/
@Override
public long seek(long offset) throws NotSupportedException {
throw new NotSupportedException("Stream does not support seeking.");
}
/**
* Sets the length of the stream.
*
* @param value The desired length of the current stream in bytes.
* @return The desired length of the current stream in bytes
* @throws NotSupportedException Stream does not support both writing and seeking
*/
@Override
public void setLength(long value) throws NotSupportedException {
throw new NotSupportedException("Stream does not support both writing and seeking");
}
/**
* Writes a sequence of bytes to the current stream and advances the current position within this stream by the number of bytes written.
*
* @param buffer An array of bytes. This method copies count bytes from buffer to the current stream.
* @param offset The zero-based byte offset in buffer at which to begin copying bytes to the current stream.
* @param count The number of bytes to be written to the current stream.
* @return The total number of bytes read into the buffer. This can be less than the number of bytes requested if that many bytes are not currently available or zero (0) if the end of the stream has been reached.
* @throws Exception
*/
@Override
public void write(byte[] buffer, int offset, int count) throws Exception {
if (_mode == StreamMode.Read || _mode == StreamMode.ReadWithoutLock)
throw new StreamException("Stream does not support writing.");
((OutputCacheStream) cacheStream).write(buffer, offset, count);
}
/**
* Closes the current stream and releases any resources associated with the current stream.
*
* @return The total number of bytes read into the buffer. This can be less than the number of bytes requested if that many bytes are not currently available or zero (0) if the end of the stream has been reached.
* @throws Exception
*/
@Override
public void close()
throws Exception {
//Lets release the lock on the stream.
if (_mode == StreamMode.Read || _mode == StreamMode.ReadWithoutLock) {
((InputCacheStream) cacheStream).close();
} else {
((OutputCacheStream) cacheStream).close();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy