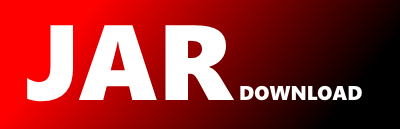
com.alachisoft.ncache.client.internal.caching.ClientCacheSearchService Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.caching;
import com.alachisoft.ncache.client.services.SearchService;
import Alachisoft.NCache.Caching.TagComparisonType;
import com.alachisoft.ncache.client.TagSearchOptions;
import com.alachisoft.ncache.client.internal.util.ConversionUtil;
import com.alachisoft.ncache.client.services.SearchService;
import com.alachisoft.ncache.runtime.caching.Tag;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import java.util.List;
public class ClientCacheSearchService extends SearchServiceImpl implements SearchService {
CacheImpl _nearCacheContainer;
protected boolean _clientCacheUsed;
CacheImpl getNearCacheContainer() {
_clientCacheUsed=true;
return _nearCacheContainer;
}
void setNearCacheContainer(CacheImpl nearCacheContainer) {
this._nearCacheContainer = nearCacheContainer;
}
public ClientCacheSearchService(CacheImpl farCache, CacheImpl nearCache) {
super(farCache);
_nearCacheContainer = nearCache;
}
///#region Tag Based Operations
@Override
public void removeByTag(Tag tag) throws CacheException {
if (tag == null || tag.getTagName() == null)
{
throw new IllegalArgumentException("Tag cannot be null.");
}
getNearCacheContainer().removeByTag(new Tag[] {tag}, TagComparisonType.BY_TAG);
if (_clientCacheUsed) getCacheContainer().removeByTag(new Tag[] {tag}, TagComparisonType.BY_TAG);
}
@Override
public void removeByTags(List tags, TagSearchOptions type) throws CacheException {
if (tags == null)
{
throw new IllegalArgumentException("Tags cannot be null.\nParameter name: tags");
}
Tag[] tagsList = (new java.util.ArrayList(tags)).toArray(new Tag[0]);
if (tagsList.length == 0)
{
throw new IllegalArgumentException("At least one Tag required.");
}
removeDuplicateTags(tagsList);
getNearCacheContainer().removeByTag(tagsList, getTagComparisonType(type));
if (_clientCacheUsed) getCacheContainer().removeByTag(tagsList, getTagComparisonType(type));
}
///#endregion
///#region Group Based Operations
@Override
public void removeGroupData(String group) throws CacheException {
getNearCacheContainer().removeGroupData(group);
if (_clientCacheUsed) getCacheContainer().removeGroupData(group);
}
///#endregion
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy