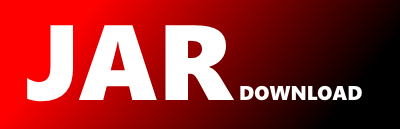
com.alachisoft.ncache.client.internal.caching.ContinuousQueryManager Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client.internal.caching;
import Alachisoft.NCache.Common.BitSet;
import Alachisoft.NCache.Common.TopicConstant;
import com.alachisoft.ncache.client.ContinuousQuery;
import com.alachisoft.ncache.client.EventCacheItem;
import com.alachisoft.ncache.runtime.caching.messaging.MessageReceivedListener;
import com.alachisoft.ncache.runtime.events.EventDataFilter;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import java.util.ArrayList;
import java.util.List;
/**
* @author Administrator
*/
public class ContinuousQueryManager {
Object sync = new Object();
private ArrayList queries = new ArrayList();
private TopicSubscriptionImpl _cqEventsSubscription;
private static boolean tracingEnabled;
CacheImplBase cacheImpl;
CQEventMessageReceivedListener cQEventMessageReceivedListener = null;
public ContinuousQueryManager() {
String eventsTracingEnabled = System.getProperty("EventsTracingEnabled");
tracingEnabled = eventsTracingEnabled != null && eventsTracingEnabled.toLowerCase().equals("true");
if(cQEventMessageReceivedListener == null){
cQEventMessageReceivedListener = new CQEventMessageReceivedListener(this);
}
}
public static boolean getTracingEnabled() {
return tracingEnabled;
}
public static void setTracingEnabled(boolean value) {
tracingEnabled = value;
}
public void register(ContinuousQuery query, CacheImpl cache) throws CacheException {
cacheImpl = cache.getCacheImpl();
cQEventMessageReceivedListener.setCacheImplBase(cacheImpl);
if (tracingEnabled) {
System.out.println("CQManager.RegisterQuery() -> query : {query.Command} server id: {query.ServerUniqueID}");
}
synchronized (sync) {
if (!queries.contains(query)) {
queries.add(query);
} else {
queries.remove(query);
queries.add(query);
}
}
if (_cqEventsSubscription == null) {
TopicImpl topic = (TopicImpl) cache._messagingService.getTopic(TopicConstant.CQEventsTopic, true);
_cqEventsSubscription = (TopicSubscriptionImpl) topic.createEventSubscription(cQEventMessageReceivedListener);
}
}
public void unRegister(ContinuousQuery query) throws Exception {
synchronized (sync) {
queries.remove(query);
}
if (queries.size() == 0) {
if (_cqEventsSubscription != null) {
_cqEventsSubscription.unSubscribeEventTopic();
_cqEventsSubscription = null;
}
}
}
public void notify(String queryId, QueryChangeType changeType, String key, boolean notifyAsync, EventCacheItem item, EventCacheItem oldItem, BitSet flag, String cacheName, EventDataFilter datafilter) {
List registeredQueries = null;
//Copy to a temp list to avoid enumeration exception when notification and query registration occurs in parallel.
synchronized (sync) {
if (queries.size() == 0) {
return;
}
registeredQueries = new ArrayList<>(queries);
}
if (tracingEnabled) {
System.out.println("CQManager.Notify() -> queries# ({queries.Count}) change event :{changeType}");
}
for (ContinuousQuery query : registeredQueries) {
try {
if (query.getServerUniqueIDInternal().equals(queryId)) {
if (tracingEnabled) {
System.out.println("CQManager.Notify() -> query ({queryId}) change event :{changeType}");
}
if (changeType == QueryChangeType.Add) {
query.fireCQEventsInternal(key, EventTypeInternal.ItemAdded, item, oldItem, notifyAsync, cacheName, flag, datafilter);
} else if (changeType == QueryChangeType.Remove) {
query.fireCQEventsInternal(key, EventTypeInternal.ItemRemoved, item, oldItem, notifyAsync, cacheName, flag, datafilter);
} else {
query.fireCQEventsInternal(key, EventTypeInternal.ItemUpdated, item, oldItem, notifyAsync, cacheName, flag, datafilter);
}
}
} catch (Throwable e) {
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy