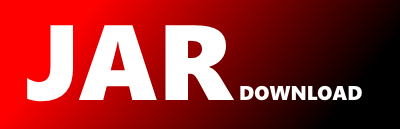
com.alachisoft.ncache.client.internal.caching.DataReaderCommon Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.caching;
import Alachisoft.NCache.Caching.CompressedValueEntry;
import Alachisoft.NCache.Common.BitSetConstants;
import Alachisoft.NCache.Common.Caching.UserBinaryObject;
import Alachisoft.NCache.Common.CompressionUtil;
import com.alachisoft.ncache.client.internal.datastructure.DataTypeCreator;
import com.alachisoft.ncache.runtime.exceptions.OperationFailedException;
import com.alachisoft.ncache.serialization.JSON.JsonBinaryFormatter;
import com.alachisoft.ncache.runtime.JSON.JsonUtil;
import com.alachisoft.ncache.serialization.standard.CompactBinaryFormatter;
import java.io.IOException;
abstract class DataReaderCommon
{
protected CacheImpl _cache;
protected T extractObjectFromCompressedValueEntry(CompressedValueEntry cmpEntry, Class> cls) throws IOException, ClassNotFoundException, OperationFailedException {
Object obj = cmpEntry.getValue();
switch (cmpEntry.getType())
{
case Set:
case List:
case Queue:
case Counter:
case Dictionary:
String key = null;
tangible.RefObject tempRef_key = new tangible.RefObject(key);
boolean tempVar = tryGetKeyFromCurrentRow(tempRef_key) && _cache != null;
key = tempRef_key.argvalue;
if (tempVar)
{
obj = DataTypeCreator.createDataTypeHandle(cmpEntry.getType(), key, _cache,cls);
}
break;
case CacheItem:
obj = convertToUserObject(cmpEntry,cls);
break;
}
return (T) obj;
}
protected T extractFromCompressedValueEntry(CompressedValueEntry cmpEntry, Class> cls) throws OperationFailedException, IOException, ClassNotFoundException {
Object obj = cmpEntry.getValue();
switch (cmpEntry.getType())
{
case Set:
case List:
case Queue:
case Counter:
case Dictionary:
String key = null;
tangible.RefObject tempRef_key = new tangible.RefObject(key);
boolean tempVar = tryGetKeyFromCurrentRow(tempRef_key) && _cache != null;
key = tempRef_key.argvalue;
if (tempVar)
{
obj = DataTypeCreator.createDataTypeHandle(cmpEntry.getType(), key, _cache,cls);
}
break;
case CacheItem:
obj = (T)convertToUserObject(cmpEntry,cls);
break;
}
return JsonUtil.getValueAs(obj,cls);
}
protected T convertToUserObject(CompressedValueEntry cmpEntry, Class> cls) throws OperationFailedException, IOException, ClassNotFoundException {
if (cmpEntry == null)
{
return null;
}
if (cmpEntry.getValue() == null)
{
return null;
}
UserBinaryObject ubObject = (UserBinaryObject)((cmpEntry.getValue() instanceof UserBinaryObject) ? cmpEntry.getValue() : null);
if (ubObject != null)
{
cmpEntry.setValue(ubObject.getFullObject());
}
if (cmpEntry.getValue() instanceof byte[])
{
if (cmpEntry.getFlag().IsBitSet((byte) BitSetConstants.Compressed))
{
cmpEntry.setValue( CompressionUtil.Decompress((byte[])cmpEntry.getValue()));
}
if (_cache != null && _cache.getCacheImpl().getEncryptionEnabled())
{
cmpEntry.setValue( CacheHelper.DecryptData((byte[])cmpEntry.getValue(), _cache.getCacheImpl().getName()));
}
if (cmpEntry.getFlag().IsBitSet((byte) BitSetConstants.BinaryData))
{
return (T)cmpEntry.getValue();
}
if (cmpEntry.getFlag().IsBitSet((byte) BitSetConstants.JsonData))
{
cmpEntry.setValue(JsonBinaryFormatter.fromByteBuffer((byte[])cmpEntry.getValue(),cls));
}
else
{
cmpEntry.setValue(CompactBinaryFormatter.fromByteBuffer((byte[])cmpEntry.getValue(), _cache.getCacheImpl().getName()));
}
}
return (T)cmpEntry.getValue();
}
protected abstract boolean tryGetKeyFromCurrentRow(tangible.RefObject key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy