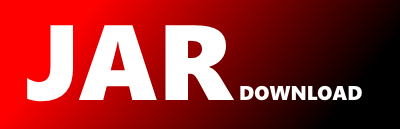
com.alachisoft.ncache.client.internal.caching.DisconnectedClientCache Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.caching;
// Copyright (c) 2018 Alachisoft
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License
import Alachisoft.NCache.Common.*;
import Alachisoft.NCache.Common.Enum.*;
import Alachisoft.NCache.Common.Locking.*;
import Alachisoft.NCache.Common.ErrorHandling.*;
import com.alachisoft.ncache.client.*;
import com.alachisoft.ncache.client.ClientInfo;
import com.alachisoft.ncache.client.datastructures.DataStructureManager;
import com.alachisoft.ncache.client.internal.util.ConversionUtil;
import com.alachisoft.ncache.client.services.MessagingService;
import com.alachisoft.ncache.client.services.NotificationService;
import com.alachisoft.ncache.client.services.SearchService;
import com.alachisoft.ncache.runtime.CacheItemPriority;
import com.alachisoft.ncache.runtime.caching.*;
import com.alachisoft.ncache.runtime.caching.expiration.Expiration;
import com.alachisoft.ncache.runtime.caching.expiration.ExpirationConstants;
import com.alachisoft.ncache.runtime.caching.expiration.ExpirationType;
import com.alachisoft.ncache.runtime.dependencies.CacheDependency;
import com.alachisoft.ncache.runtime.events.EventDataFilter;
import com.alachisoft.ncache.runtime.events.ListenerType;
import com.alachisoft.ncache.runtime.exceptions.*;
import com.alachisoft.ncache.runtime.exceptions.SecurityException;
import com.alachisoft.ncache.runtime.util.NCDateTime;
import com.alachisoft.ncache.runtime.util.TimeSpan;
import com.alachisoft.ncache.security.encryption.EncryptionMgr;
import com.alachisoft.ncache.serialization.standard.CompactBinaryFormatter;
import java.io.IOException;
import java.util.Date;
import java.util.EnumSet;
import java.util.Map;
import java.util.concurrent.FutureTask;
import java.util.concurrent.TimeUnit;
public class DisconnectedClientCache extends CacheImpl{
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Fields
/** level 1 cache
*/
protected CacheImpl _nearCache;
/** level 2 cache
*/
protected CacheImpl _farCache;
private boolean _isPessimistic = false;
protected boolean _clientCacheUsed;
private boolean _sldDefaultEnabled;
private boolean _sldLongerEnabled;
private boolean _absEnabled;
private boolean _absLongerEnabled;
private long _absDefault;
private long _absLonger;
private long _sldDeault;
private long _sldLonger;
private String _userId;
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: private byte[] _password;
private byte[] _password;
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Properties
protected boolean getThrowError()
{
return this.isExceptionsEnabled();
}
@Override
public boolean isExceptionsEnabled()
{
return _farCache.isExceptionsEnabled();
}
@Override
public void setExceptionsEnabled(boolean value)
{
_farCache.setExceptionsEnabled(value);
}
public CacheImpl getNearCacheInstance()
{
return _nearCache;
}
public void setNearCacheProperties() throws Exception {
}
public CacheSyncDependency getCacheSyncDependency(String key)
{
return new CacheSyncDependency(this._farCache._cacheId, key, _userId, _password);
}
@Override
public MessagingService getMessagingService() throws CacheException {
return _farCache.getMessagingService();
}
private SearchService privateSearchService;
@Override
public SearchService getSearchService()
{
return privateSearchService;
}
@Override
public void setSearchService(SearchService value)
{
privateSearchService = value;
}
@Override
public NotificationService getNotificationService() throws CacheException {
return _farCache.getNotificationService();
}
@Override
public java.util.List getConnectedClientList() throws CacheException {
return _farCache.getConnectedClientList();
}
@Override
public DataStructureManager getDataStructuresManager() {
return _farCache.getDataStructuresManager();
}
@Override
public SerializationFormat getSerializationFormat()
{
return _farCache.getSerializationFormat();
}
@Override
public void setSerializationFormat(SerializationFormat value)
{
super.setSerializationFormat(value);
}
@Override
public ClientInfo getClientInfo() throws CacheException {
return _farCache.getClientInfo();
}
public String getUserId()
{
return _userId;
}
public void setUserId(String value)
{
_userId = value;
}
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public byte[] getPassword()
public byte[] getPassword()
{
return _password;
}
//C# TO JAVA CONVERTER WARNING: Unsigned integer types have no direct equivalent in Java:
//ORIGINAL LINE: public void setPassword(byte[] value)
public void setPassword(byte[] value)
{
_password = value;
}
public boolean getSldDefaultEnabled()
{
return _sldDefaultEnabled;
}
public void setSldDefaultEnabled(boolean value)
{
_sldDefaultEnabled = value;
}
public boolean getSldLongerEnabled()
{
return _sldLongerEnabled;
}
public void setSldLongerEnabled(boolean value)
{
_sldLongerEnabled = value;
}
public boolean getAbsDefaultEnabled()
{
return _absEnabled;
}
public void setAbsDefaultEnabled(boolean value)
{
_absEnabled = value;
}
public boolean getAbsLongerEnabled()
{
return _absLongerEnabled;
}
public void setAbsLongerEnabled(boolean value)
{
_absLongerEnabled = value;
}
public long getSldDefault()
{
return _sldDeault;
}
public void setSldDefault(long value)
{
_sldDeault = value;
}
public long getSldLonger()
{
return _sldLonger;
}
public void setSldLonger(long value)
{
_sldLonger = value;
}
public long getAbsDefault()
{
return _absDefault;
}
public void setAbsDefault(long value)
{
_absDefault = value;
}
public long getAbsLonger()
{
return _absLonger;
}
public void setAbsLonger(long value)
{
_absLonger = value;
}
@Override
public boolean getExceptionEnabled()
{
return _farCache.getExceptionEnabled();
}
@Override
public void setExceptionEnabled(boolean value)
{
_farCache.setExceptionsEnabled(value);
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Constructor
public DisconnectedClientCache(CacheImpl l2, CacheImpl l1, String userid, String pswd, boolean isPessimistic) throws Exception {
if (l2 == null)
{
throw new OperationFailedException(ErrorCodes.CacheInit.L2_CACHE_NOT_INIT, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.L2_CACHE_NOT_INIT));
}
_nearCache = l1;
_farCache = l2;
GetClientExpiration();
_isPessimistic = isPessimistic;
setNearCacheProperties();
if (userid != null)
{
_userId = userid;
}
if (pswd != null)
{
_password = EncryptionUtil.Encrypt(pswd);
}
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
@Override
public CacheImpl getCacheInstanceInternal()
{
return _farCache;
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Count
@Override
public long getCount() throws CacheException {
return _farCache.getCount();
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Contains Operations
@Override
public boolean contains(String key) throws CacheException {
return _farCache.contains(key);
}
@Override
public java.util.Map containsBulk(Iterable keys) throws CacheException {
return _farCache.containsBulk(keys);
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Clear Operations
@Override
public void clearClientCache() throws CacheException {
if (_nearCache != null) {
_nearCache.clear();
//Bad code is being added to get around with KeyAlready exist problem. [Asif Imam]
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
}
}
_nearCache.clear();
}
@Override
public void clear() throws CacheException {
_farCache.clear();
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Add Operations
@Override
public CacheItemVersion addOperation(String key, Object value, CacheDependency dependency, CacheSyncDependency syncDependency, java.util.Date absoluteExpiration,
TimeSpan slidingExpiration, CacheItemPriority priority, WriteMode writeOption, DataSourceModifiedListener dataSourceModifiedCallback, EventTypeInternal eventTypeInternal ,
boolean isResyncExpiredItems, String group,
Tag[] tags, String providerName, String resyncProviderName, NamedTagsDictionary namedTags,
CacheDataModificationListener cacheItemUdpatedCallback, CacheDataModificationListener cacheItemRemovedCallaback,
EventDataFilter itemUpdateDataFilter, EventDataFilter itemRemovedDataFilter, tangible.RefObject size,
boolean allowQueryTags, String clientId, short updateCallbackID, short removeCallbackID, short dsItemAddedCallbackID) throws CacheException {
if (_farCache.getCacheImpl() == null)
{
throw new OperationFailedException(ErrorCodes.CacheInit.CACHE_NOT_INIT, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.CACHE_NOT_INIT));
}
CacheItemVersion result = null;
try
{
updateCallbackID = _farCache.registerUpdateSorrogateCallback(null, cacheItemUdpatedCallback, itemUpdateDataFilter);
removeCallbackID = _farCache.registerRemoveSorrogateCallback(null, cacheItemRemovedCallaback, itemRemovedDataFilter);
dsItemAddedCallbackID = _farCache.GetCallbackId(dataSourceModifiedCallback, 1 ,eventTypeInternal);
String clientID = updateCallbackID < 0 && removeCallbackID < 0 && dsItemAddedCallbackID < 0 ? null : _farCache.getCacheImpl().getClientID();
result = getNearCacheInstance().addOperation(key, value, dependency, new CacheSyncDependency(_farCache._cacheId, key, getUserId(), getPassword()), GetDefaultAbsoluteExpiration(absoluteExpiration), GetDefaultSlidingExpiration(slidingExpiration), priority, writeOption, dataSourceModifiedCallback, eventTypeInternal, isResyncExpiredItems, group, tags, providerName, resyncProviderName, namedTags, cacheItemUdpatedCallback, cacheItemRemovedCallaback, itemUpdateDataFilter, itemRemovedDataFilter, size, allowQueryTags, clientID, updateCallbackID, removeCallbackID, dsItemAddedCallbackID);
}
catch (RuntimeException | CacheException e)
{
if (this.getThrowError())
{
throw e;
}
}
return result;
}
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override IDictionary AddBulk(IDictionary items, WriteThruOptions writeThruOptions = null)
@Override
public java.util.Map addBulk(java.util.Map items, WriteThruOptions writeThruOptions) throws OperationFailedException, StreamNotFoundException, StreamException, ConfigurationException, CommandException, AggregateException, GeneralFailureException, OperationNotSupportedException, StreamAlreadyLockedException, LicensingException, SecurityException {
if (items == null)
{
throw new IllegalArgumentException("items");
}
if (items.isEmpty())
{
throw new IllegalArgumentException("Adding empty dictionary into cache.");
}
if (_farCache.getCacheImpl() == null)
{
throw new OperationFailedException(ErrorCodes.CacheInit.CACHE_NOT_INIT, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.CACHE_NOT_INIT));
}
long[] sizes = new long[items.size()];
String providerName = null;
WriteMode mode = WriteMode.None;
DataSourceModifiedListener dataSourceModifiedListener = null;
short updateCallbackID = -1;
short removeCallbackID = -1;
EnumSet eventTypes = EnumSet.of(EventTypeInternal.None);
tangible.RefObject tempRef_mode = new tangible.RefObject(mode);
tangible.RefObject tempRef_providerName = new tangible.RefObject(providerName);
tangible.RefObject tempRef_dataSourceModifiedCallback = new tangible.RefObject(dataSourceModifiedListener);
tangible.RefObject> tempRef_eventTypes = new tangible.RefObject(eventTypes);
ConversionUtil.GetWriteOptions(writeThruOptions, tempRef_mode, tempRef_providerName, tempRef_dataSourceModifiedCallback,tempRef_eventTypes);
mode = tempRef_mode.argvalue;
providerName = tempRef_providerName.argvalue;
dataSourceModifiedListener = tempRef_dataSourceModifiedCallback.argvalue;
eventTypes = tempRef_eventTypes.argvalue;
//Consider only first element from enumset. For now multiple events are not supported.
EventTypeInternal eventTypeInternal = (EventTypeInternal) eventTypes.toArray()[0];
CacheItem cacheItemAtFirstIndex = items.entrySet().iterator().next().getValue();
for (Map.Entry item : items.entrySet())
{
if (item.getValue() == null)
{
throw new IllegalArgumentException("CacheItem cannot be null");
}
CacheItem l2item = item.getValue();
l2item.setExpiration(l2item.getExpiration());
l2item.setSyncDependency(getCacheSyncDependency(item.getKey()));
}
try
{
if (cacheItemAtFirstIndex != null)
{
updateCallbackID = _farCache.registerUpdateSorrogateCallback(null, CacheItemWrapperInternal.getCacheItemUpdatedListener(cacheItemAtFirstIndex), CacheItemWrapperInternal.getItemUpdatedDataFilter(cacheItemAtFirstIndex));
removeCallbackID = _farCache.registerRemoveSorrogateCallback(null, CacheItemWrapperInternal.getCacheItemRemovedListener(cacheItemAtFirstIndex), CacheItemWrapperInternal.getItemRemovedDataFilter(cacheItemAtFirstIndex));
}
short dsItemAddedCallbackID = _farCache.GetCallbackId(dataSourceModifiedListener, items.size(), eventTypeInternal);
String clientID = updateCallbackID < 0 && removeCallbackID < 0 && dsItemAddedCallbackID < 0 ? null : _farCache.getCacheImpl().getClientID();
return getNearCacheInstance().AddBulkInternal(items, mode, dataSourceModifiedListener, eventTypeInternal, providerName, true, clientID, updateCallbackID, removeCallbackID, dsItemAddedCallbackID,
cacheItemAtFirstIndex != null ? CacheItemWrapperInternal.getItemUpdatedDataFilter(cacheItemAtFirstIndex) : EventDataFilter.None,
cacheItemAtFirstIndex != null ? CacheItemWrapperInternal.getItemRemovedDataFilter(cacheItemAtFirstIndex) : EventDataFilter.None, false);
}
catch (CacheException e)
{
if (getThrowError())
{
throw e;
}
}
return null;
}
@Override
public CacheItemVersion insertOperation(String key, Object value, CacheDependency dependency, CacheSyncDependency syncDependency, java.util.Date absoluteExpiration, TimeSpan slidingExpiration, CacheItemPriority priority, WriteMode WriteMode,DataSourceModifiedListener dataSourceModifiedCallback,EventTypeInternal eventType,boolean isResyncExpiredItems, String group,LockHandle lockHandle, CacheItemVersion version, LockAccessType accessType, Tag[] tags, String providerName, String resyncProviderName, NamedTagsDictionary namedTags, CacheDataModificationListener cacheItemUdpatedCallback, CacheDataModificationListener onRemoveCallback, EventDataFilter itemUpdateDataFilter, EventDataFilter itemRemovedDataFilter, boolean allowQueryTags, String clientId, short updateCallbackId, short removeCallbackId, short dataSourceUpdatedCallbackId) throws CacheException {
CacheItemVersion newVersion = null;
Object lockId = (lockHandle == null) ? null : lockHandle.getLockId();
if (_farCache.getCacheImpl() == null)
{
throw new OperationFailedException(ErrorCodes.CacheInit.CACHE_NOT_INIT, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.CACHE_NOT_INIT));
}
try
{
updateCallbackId = _farCache.registerUpdateSorrogateCallback(null, cacheItemUdpatedCallback, itemUpdateDataFilter);
removeCallbackId = _farCache.registerRemoveSorrogateCallback(null, onRemoveCallback, itemRemovedDataFilter);
dataSourceUpdatedCallbackId = _farCache.GetCallbackId(dataSourceModifiedCallback, 1, eventType);
String clientID = updateCallbackId < 0 && removeCallbackId < 0 && dataSourceUpdatedCallbackId < 0 ? null : _farCache.getCacheImpl().getClientID();
newVersion = getNearCacheInstance().insertOperation(key, value, dependency,
new CacheSyncDependency(_farCache._cacheId, key, getUserId(), getPassword()),
GetDefaultAbsoluteExpiration(absoluteExpiration),
GetDefaultSlidingExpiration(slidingExpiration), priority, WriteMode, dataSourceModifiedCallback, eventType,
isResyncExpiredItems, group, lockHandle, version,
accessType, tags, providerName, resyncProviderName, namedTags, cacheItemUdpatedCallback,onRemoveCallback,
itemUpdateDataFilter, itemRemovedDataFilter, allowQueryTags, clientID,
updateCallbackId, removeCallbackId, dataSourceUpdatedCallbackId);
}
catch (CacheException e)
{
if (getThrowError()) throw e;
}
return newVersion;
}
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override IDictionary InsertBulk(IDictionary items, WriteThruOptions writeThruOptions = null)
@Override
public java.util.Map insertBulk(java.util.Map items, WriteThruOptions writeThruOptions) throws CacheException {
String providerName = null;
WriteMode mode = WriteMode.None;
DataSourceModifiedListener dataSourceModifiedCallback = null;
EnumSet eventTypes = EnumSet.of(EventTypeInternal.None);
tangible.RefObject tempRef_mode = new tangible.RefObject(mode);
tangible.RefObject tempRef_providerName = new tangible.RefObject(providerName);
tangible.RefObject tempRef_dataSourceModifiedCallback = new tangible.RefObject(dataSourceModifiedCallback);
tangible.RefObject> tempRef_eventTypes = new tangible.RefObject(eventTypes);
ConversionUtil.GetWriteOptions(writeThruOptions, tempRef_mode, tempRef_providerName, tempRef_dataSourceModifiedCallback,tempRef_eventTypes);
mode = tempRef_mode.argvalue;
providerName = tempRef_providerName.argvalue;
dataSourceModifiedCallback = tempRef_dataSourceModifiedCallback.argvalue;
eventTypes = tempRef_eventTypes.argvalue;
//Consider only first element from enumset. For now multiple events are not supported.
EventTypeInternal eventTypeInternal = (EventTypeInternal) eventTypes.toArray()[0];
if (items == null)
{
throw new java.lang.IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: items");
}
if (items.isEmpty())
{
throw new IllegalArgumentException("There is no key present in keys array");
}
if (_farCache.getCacheImpl() == null)
{
throw new OperationFailedException(ErrorCodes.CacheInit.CACHE_NOT_INIT, ErrorMessages.
getErrorMessage(ErrorCodes.CacheInit.CACHE_NOT_INIT));
}
try
{
long[] updateSize = new long[items.size()];
CacheItem cacheItemAtFirstIndex = items.entrySet().iterator().next().getValue();
short updateCallbackId = -1;
short removeCallbackId = -1;
//C# TO JAVA CONVERTER TODO TASK: There is no equivalent to implicit typing in Java:
for ( Map.Entry item:items.entrySet())
{
CacheItem l2CacheItem = item.getValue();
if (l2CacheItem != null)
{
l2CacheItem.setExpiration (GetExpiration(l2CacheItem.getExpiration()));
l2CacheItem.setSyncDependency( getCacheSyncDependency(item.getKey()));
l2CacheItem.setGroup( l2CacheItem.getGroup());
l2CacheItem.setTags(l2CacheItem.getTags());
l2CacheItem.setNamedTags( l2CacheItem.getNamedTags());
}
}
if (cacheItemAtFirstIndex != null)
{
updateCallbackId = _farCache.registerUpdateSorrogateCallback(null, CacheItemWrapperInternal.getCacheItemUpdatedListener(cacheItemAtFirstIndex), CacheItemWrapperInternal.getItemUpdatedDataFilter(cacheItemAtFirstIndex));
removeCallbackId = _farCache.registerRemoveSorrogateCallback(null, CacheItemWrapperInternal.getCacheItemRemovedListener(cacheItemAtFirstIndex), CacheItemWrapperInternal.getItemRemovedDataFilter(cacheItemAtFirstIndex));
}
short dsItemUpdateCallbackID = _farCache.GetCallbackId(dataSourceModifiedCallback, items.size(), eventTypeInternal);
String clientID = updateCallbackId < 0 && removeCallbackId < 0 && dsItemUpdateCallbackID < 0 ? null : _farCache.getCacheImpl().getClientID();
java.util.Map itemVersions = null;
tangible.RefObject tempRef_itemVersions = new tangible.RefObject(itemVersions);
tangible.RefObject tempRef_updateSize = new tangible.RefObject(updateSize);
return getNearCacheInstance().InsertBulkInternal(items, mode, dataSourceModifiedCallback, eventTypeInternal, providerName, tempRef_updateSize, true, clientID, updateCallbackId, removeCallbackId, dsItemUpdateCallbackID,
cacheItemAtFirstIndex != null ? CacheItemWrapperInternal.getItemUpdatedDataFilter(cacheItemAtFirstIndex) : EventDataFilter.None,
cacheItemAtFirstIndex != null ? CacheItemWrapperInternal.getItemRemovedDataFilter(cacheItemAtFirstIndex) : EventDataFilter.None, false, tempRef_itemVersions);
}
catch (CacheException e)
{
if (getThrowError())
{
throw e;
}
}
return null;
}
@Override
public boolean updateAttributes(String key, CacheItemAttributes attributes) throws CacheException {
boolean success = false;
if (key == null)
{
throw new IllegalArgumentException();
}
if (attributes == null)
{
throw new IllegalArgumentException();
}
if (_farCache == null)
{
throw new OperationFailedException(ErrorCodes.CacheInit.L2_CACHE_NOT_INIT, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.L2_CACHE_NOT_INIT));
}
try
{
success = _farCache.updateAttributes(key, attributes);
if (success)
{
success = getNearCacheInstance().updateAttributes(key, attributes);
}
}
catch (RuntimeException e)
{
if (getThrowError())
{
throw e;
}
}
return success;
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Remove Opewrations
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override bool Remove(string key, out T removedItem, LockHandle lockHandle = null, CacheItemVersion version = null, WriteThruOptions writeThruOptions = null)
@Override
public T remove(String key, LockHandle lockHandle, CacheItemVersion version, WriteThruOptions writeThruOptions,Class> cls) throws CacheException {
return getNearCacheInstance().remove(key, lockHandle, version, writeThruOptions,cls);
}
@Override
public void delete(String key, LockHandle lockHandle, CacheItemVersion version, WriteThruOptions writeThruOptions) throws CacheException {
getNearCacheInstance().delete(key, lockHandle, version, writeThruOptions);
}
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override void RemoveBulk(IEnumerable keys, out IDictionary removedItems, WriteThruOptions writeThruOption = null)
@Override
public Map removeBulk(Iterable keys, WriteThruOptions writeThruOption, Class> cls) throws CacheException {
return getNearCacheInstance().removeBulk(keys, writeThruOption,cls);
}
@Override
public void deleteBulk(Iterable keys, WriteThruOptions writeThruOptions) throws CacheException {
getNearCacheInstance().deleteBulk(keys, writeThruOptions);
}
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override void RemoveBulk(IEnumerable keys, WriteThruOptions writeThruOption = null)
// @Override
// public Map removeBulk(Iterable keys, WriteThruOptions writeThruOption, Class> cls) throws CacheException {
// return _nearCache.removeBulk(keys, writeThruOption,cls);
// }
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override Task RemoveAsync(string key, WriteThruOptions writeThruOptions = null)
@Override
public FutureTask removeAsync(String key, WriteThruOptions writeThruOptions, Class> cls)
{
return getNearCacheInstance().removeAsync(key, writeThruOptions,cls);
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Get Operations
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override T Get(string key, ReadThruOptions readThruOptions = null)
@Override
public T get(String key, ReadThruOptions readThruOptions, Class> cls) throws CacheException {
T result = null;
if (_isPessimistic)
{
CacheItemVersion version = new CacheItemVersion();
T localItem = getNearCacheInstance().get(key, version, readThruOptions,cls);
try
{
if (localItem!=null)
{
result = _farCache.getIfNewer(key, version,cls);
//if l2 does not return the item because item there is not
//newer then return the local item. otherwise return null because
//item is removed from l2.
if (result==null)
{
if (version.getVersion() == new CacheItemVersion().getVersion())
{
return localItem;
}
else
{
return null;
}
}
}
}
catch (CacheException e)
{
if (getThrowError())
{
throw e;
}
}
}
else
{
try
{
CacheItem cacheItem = getNearCacheInstance().getCacheItem(key, readThruOptions);
CacheItemWrapperInternal.setCacheInstance(cacheItem, this);
return cacheItem != null ? cacheItem.getValue(cls) : null;
}
catch (RuntimeException e2)
{
if (getThrowError())
{
throw e2;
}
}
}
return result;
}
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override T Get(string key, ref CacheItemVersion version, ReadThruOptions readThruOptions = null)
@Override
public T get(String key, CacheItemVersion version, ReadThruOptions readThruOptions, Class> cls) throws CacheException {
CacheItem cacheItem = getNearCacheInstance().getCacheItem(key, version, readThruOptions);
if (cacheItem != null)
{
if ( cacheItem.getCacheItemVersion().getVersion()!= version.getVersion())
{
version = cacheItem.getCacheItemVersion();
return null;
}
else
{
version = cacheItem.getCacheItemVersion();
}
}
return cacheItem != null ? cacheItem.getValue(cls) : null;
}
@Override
public T get(String key, boolean acquireLock, TimeSpan lockTimeout, LockHandle lockHandle, Class> cls) throws CacheException {
try
{
return getNearCacheInstance().get(key, acquireLock, lockTimeout, lockHandle,cls);
}
catch (RuntimeException | CacheException e)
{
if (getThrowError())
{
throw e;
}
}
return null;
}
@Override
public T getInternal(String key, CacheItemVersion version,LockAccessType accessType, TimeSpan lockTimeout, LockHandle lockHandle, ReadThruOptions readOptions, Class> cls) throws CacheException {
T result = null;
if (_isPessimistic)
{
try
{
CacheItem cItem = _farCache.getCacheItem(key, version,readOptions);
T localItem = null;
if (cItem != null)
{
localItem = cItem.getValue(cls);
version= cItem.getCacheItemVersion();
}
if (localItem==null)
{
result = _farCache.getIfNewer(key, version,cls);
//muds:
//if l2 does not return the item because item there is not
//newer then return the local item. otherwise return null because
//item is removed from l2.
if (result==null)
{
if (version == null)
{
return localItem;
}
else
{
return null;
}
}
}
}
catch (CacheException e)
{
if (getThrowError())
{
throw e;
}
}
}
else
{
try
{
result = getNearCacheInstance().getInternal(key, version, accessType, lockTimeout, lockHandle, readOptions,cls);
}
catch (RuntimeException e2)
{
if (getThrowError())
{
throw e2;
}
}
}
return result;
}
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override IDictionary GetBulk(IEnumerable keys, ReadThruOptions readThruOptions = null)
@Override
public java.util.Map getBulk(Iterable keys, ReadThruOptions readThruOptions, Class> cls) throws CacheException {
java.util.Map clientResult = null;
try
{
clientResult = getNearCacheInstance().getBulk(keys, readThruOptions,cls);
}
catch (RuntimeException | CacheException e)
{
if (getThrowError())
{
throw e;
}
}
return clientResult;
}
//C# TO JAVA CONVERTER TODO TASK: C# optional parameters are not converted to Java:
//ORIGINAL LINE: public override CacheItem GetCacheItem(string key, ReadThruOptions readThruOptions = null)
@Override
public CacheItem getCacheItem(String key, ReadThruOptions readThruOptions) throws CacheException {
CacheItem cacheItem = null;
if (_isPessimistic)
{
try
{
CacheItemVersion version = new CacheItemVersion();
cacheItem = getNearCacheInstance().getCacheItem(key,version, readThruOptions);
if (cacheItem != null)
{
Object result = _farCache.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy