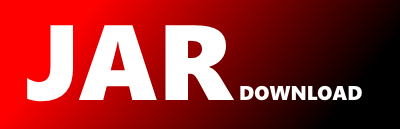
com.alachisoft.ncache.client.internal.caching.FailSafeClientCacheSearchService Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.caching;
import com.alachisoft.ncache.client.CacheConnectionOptions;
import com.alachisoft.ncache.client.services.SearchService;
import Alachisoft.NCache.Caching.TagComparisonType;
import com.alachisoft.ncache.client.TagSearchOptions;
import com.alachisoft.ncache.client.internal.util.ConversionUtil;
import com.alachisoft.ncache.client.services.SearchService;
import com.alachisoft.ncache.runtime.caching.Tag;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import java.util.List;
public class FailSafeClientCacheSearchService extends ClientCacheSearchService
{
protected boolean _isClientCacheActive;
boolean getIsClientCacheActive()
{
return _isClientCacheActive;
}
void setIsClientCacheActive(boolean value)
{
_isClientCacheActive= value;
}
public FailSafeClientCacheSearchService(CacheImpl cache, CacheImpl nearCache)
{
super(cache, nearCache);
if (nearCache == null)
{
setIsClientCacheActive(false);
_clientCacheUsed = false;
}
else
{
setIsClientCacheActive(true);
_clientCacheUsed = true;
}
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Tag Based Operations
@Override
public void removeByTag(Tag tag) throws CacheException {
try
{
super.removeByTag(tag);
}
catch (RuntimeException ex)
{
if (IsConnectivityErrorFromClientCache(ex))
{
SwitchToClusteredCache();
super.removeByTag(tag);
}
else
{
throw ex;
}
}
}
@Override
public void removeByTags(List tags, TagSearchOptions type) throws CacheException {
try
{
super.removeByTags(tags, type);
}
catch (RuntimeException ex)
{
if (IsConnectivityErrorFromClientCache(ex))
{
SwitchToClusteredCache();
super.removeByTags(tags, type);
}
else throw ex;
}
}
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#endregion
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
///#region Group Based Operations
@Override
public void removeGroupData(String group) throws CacheException {
try
{
super.removeGroupData(group);
}
catch (RuntimeException ex)
{
if (IsConnectivityErrorFromClientCache(ex))
{
SwitchToClusteredCache();
super.removeGroupData(group);
}
else
{
throw ex;
}
}
}
private boolean IsConnectivityErrorFromClientCache(RuntimeException exception)
{
return exception != null && exception.getMessage().contains("No server is available") && _clientCacheUsed;
}
private void SwitchToClusteredCache()
{
synchronized (this)
{
setIsClientCacheActive(false);
}
}
@Override
public CacheImpl getNearCacheContainer()
{
if (getIsClientCacheActive())
{
_clientCacheUsed = true;
return _nearCacheContainer;
}
_clientCacheUsed = false;
return cacheContainer;
}
public final void SetClientCacheActive(CacheImpl cache)
{
synchronized (this)
{
setIsClientCacheActive(true);
_nearCacheContainer = cache;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy