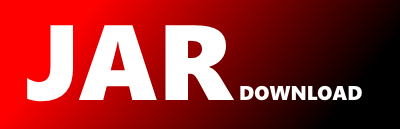
com.alachisoft.ncache.client.internal.caching.OutputCacheStream Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client.internal.caching;
import com.alachisoft.ncache.client.CacheStream;
import com.alachisoft.ncache.client.StreamMode;
import com.alachisoft.ncache.runtime.exceptions.NotSupportedException;
import com.alachisoft.ncache.runtime.util.HelperFxn;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.IOException;
import java.io.OutputStream;
/**
* OutputCacheStream is derived from System.IO.OutputStream. It is designed to put/fetch BLOB using standard OutputStream Interface
*
* @author Administrator
*/
class OutputCacheStream extends OutputStream implements CacheStream {
private CacheImpl _cacheHandle;
private StreamMode _mode;
private String _key;
private long _position;
private String _lockHandle;
private boolean _closed;
private long _length;
OutputCacheStream(String key, StreamMode mode, CacheImpl cacheHandle, String lockHandle, long length) {
_cacheHandle = cacheHandle;
_mode = mode;
_key = key;
_length = length;
_lockHandle = lockHandle;
if (_mode == StreamMode.Write)
_position = _length;
}
@Override
public void write(int b) throws IOException {
//throw new UnsupportedOperationException("Not supported yet.");
byte[] buffer = new byte[1];
buffer[0] = (byte) b;
write(buffer, 0, buffer.length);
}
@Override
public void write(byte b[], int off, int len) throws IOException {
if (_mode == StreamMode.Read || _mode == StreamMode.ReadWithoutLock)
throw new IOException("Stream does not support writing.");
if (_closed) throw new IOException("Methods were called after the stream was closed.");
if (b == null) throw new IllegalArgumentException("Value cannot be null.\r\nParameter name: buffer");
if (off + len > b.length) throw new IOException("Sum of offset and count is greater than the buffer length.");
if (off < 0 || len < 0) throw new IOException("offset or count is negative.");
byte[] bufferCopy = b;
if (len != b.length) {
bufferCopy = new byte[len];
HelperFxn.BlockCopy(b, off, bufferCopy, 0, len);
off = 0;
}
try {
_cacheHandle.writeToStream(_key, _lockHandle, bufferCopy, off, (int) _position, len);
} catch (Exception exception) {
throw new IOException(exception.getMessage());
}
_position += len;
}
@Override
public void close() throws IOException {
_closed = true;
//Lets release the lock on the stream.
if (_mode == StreamMode.Write) {
try {
_cacheHandle.closeStream(_key, _lockHandle);
} catch (Exception exception) {
throw new IOException(exception.getMessage());
}
}
}
public boolean canRead() {
return _mode != StreamMode.Write ? true : false;
}
public boolean canSeek() {
return false;
}
public boolean canWrite() {
return _mode == StreamMode.Write ? true : false;
}
public boolean closed() {
return _closed;
}
public void flush() {
}
public long length() throws Exception {
if (_closed) throw new IllegalAccessException("Methods were called after the stream was closed.");
return _cacheHandle.getStreamLength(_key, _lockHandle);
}
public long position() throws Exception {
if (!canSeek())
throw new NotSupportedException("Operation not supported");
return 0;
}
public long seek(long offset) throws NotSupportedException {
throw new UnsupportedOperationException("Not supported yet.");
}
public void setLength(long value) throws NotSupportedException {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public BufferedInputStream getBufferedInputStream(int bufferSize) throws Exception {
return null;
}
@Override
public BufferedInputStream getBufferedInputStream() throws Exception {
return null;
}
@Override
public BufferedOutputStream getBufferedOutputStream(int bufferSize) throws Exception {
return null;
}
@Override
public BufferedOutputStream getBufferedOutputStream() throws Exception {
return null;
}
@Override
public int read(byte[] buffer, int offset, int count) throws Exception {
return 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy