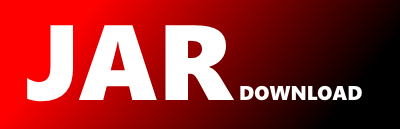
com.alachisoft.ncache.client.internal.caching.SyncCache Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.caching;
import Alachisoft.NCache.Caching.CacheSynchronization.ISyncCache;
import Alachisoft.NCache.Caching.CacheSynchronization.ISyncCacheEventsListener;
import Alachisoft.NCache.Common.BitSet;
import Alachisoft.NCache.Common.BitSetConstants;
import Alachisoft.NCache.Common.CompressionUtil;
import Alachisoft.NCache.Common.ErrorHandling.ErrorCodes;
import Alachisoft.NCache.Common.ErrorHandling.ErrorMessages;
import Alachisoft.NCache.Common.Event.PollingResult;
import com.alachisoft.ncache.client.*;
import com.alachisoft.ncache.client.ServerInfo;
import com.alachisoft.ncache.client.internal.util.ConfigReader;
import com.alachisoft.ncache.runtime.CacheItemPriority;
import com.alachisoft.ncache.runtime.caching.ReadMode;
import com.alachisoft.ncache.runtime.caching.ReadThruOptions;
import com.alachisoft.ncache.runtime.caching.datasource.ResyncOptions;
import com.alachisoft.ncache.runtime.caching.expiration.Expiration;
import com.alachisoft.ncache.runtime.dependencies.CacheDependency;
import com.alachisoft.ncache.runtime.events.EventDataFilter;
import com.alachisoft.ncache.runtime.events.EventType;
import com.alachisoft.ncache.runtime.events.ListenerType;
import com.alachisoft.ncache.runtime.exceptions.*;
import com.alachisoft.ncache.runtime.util.TimeSpan;
import com.alachisoft.ncache.serialization.core.io.ICompactSerializable;
import com.alachisoft.ncache.serialization.core.io.NCacheObjectInput;
import com.alachisoft.ncache.serialization.core.io.NCacheObjectOutput;
import tangible.RefObject;
import java.io.IOException;
import java.io.Serializable;
import java.lang.SecurityException;
import java.util.*;
import static tangible.DotNetToJavaStringHelper.isNullOrEmpty;
public class SyncCache implements ISyncCache, ICompactSerializable, Serializable, CacheStatusEventListener, CacheDataModificationListener, CacheClearedListener, CacheStoppedListener, PollNotificationListener {
private CacheImpl _syncCache;
private String _cacheId;
private String _server;
private int _port;
private ArrayList _synEventListeners = new ArrayList();
private ArrayList _synPollEventListeners = new ArrayList();
//private static final int _compressed = BitSetConstants.Compressed;
private boolean _modeInproc = false;
private CacheDataModificationListener _updateListener;
private CacheDataModificationListener _removedListener;
private CacheClearedListener _cacheClearListener;
private CacheStoppedListener cacheStoppedListener;
private PollNotificationListener pollNotificationListener;
///
/// Given CacheID, server and port creates an instance of SyncCache.
///
/// Cache ID to sync with.
/// server to connect.
/// server port.
public SyncCache(String cacheId, String server, int port, boolean isInproc) {
_cacheId = cacheId;
_server = server;
_port = port;
_modeInproc = isInproc;
}
/**
* @deprecated Only to be used by Serialization
*/
@Deprecated
public SyncCache() {
}
public boolean getIsModeInProc() {
return _modeInproc;
}
// #region/ --- CacheEvent listener ---- /
///
/// Raises CacheCleared event when SyncCache is cleared.
///
public void cacheCleared() {
if (_synEventListeners != null) {
for (Object eventListener : _synEventListeners) {
((ISyncCacheEventsListener) eventListener).CacheCleared();
}
}
}
///
/// Raises CacheStopped event when Cache Stops.
///
/// CacheId of the cache stopped.
///
/// Raises SyncItemUpdated event when item is updated in the cache.
///
/// updated key
public void itemChangedListener(String key, CacheEventArg eventArgs) {
if (_synEventListeners != null) {
if (eventArgs.getEventType() == EventType.ItemUpdated) {
for (Object eventListener : _synEventListeners) {
((ISyncCacheEventsListener) eventListener).SyncItemUpdated(key);
}
} else if (eventArgs.getEventType() == EventType.ItemRemoved) {
for (Object eventListener : _synEventListeners) {
((ISyncCacheEventsListener) eventListener).SyncItemRemoved(key);
}
}
}
}
///
/// Raises SyncItemRemoved event when item is removed.
///
/// key of the item removed.
/// value of the item removed.
/// reason for the item removed.
public void itemRemovedListener(String key, CacheEventArg eventArgs) {
if (_synEventListeners != null) {
for (Object eventListener : _synEventListeners) {
((ISyncCacheEventsListener) eventListener).SyncItemRemoved(key);
}
}
}
// #endregion
//
// #region/ --- ISyncCache Members --- /
///
/// Gets the item for the given key.
///
/// the key to fetch from cache.
/// Value of the item. null if item does not exist in the cache.
//#if VS2003
// [CLSCompliant(false)]
//#endif
@Override
public Object Get(String key, RefObject version, RefObject flag, RefObject absoluteExpiration, RefObject slidingExpiration, RefObject group, RefObject queryInfo, RefObject cacheDependency, RefObject resyncOptions, RefObject priority) throws Exception {
Object val = null;
CacheItem item = null;
if (_syncCache != null) {
val = _syncCache.getSerializedObject(key, new ReadThruOptions(ReadMode.None, ""), version, flag, absoluteExpiration, slidingExpiration, group, queryInfo, cacheDependency, resyncOptions, priority);
item = val instanceof CacheItem? (CacheItem) val : null;
if(item == null)
return null;
if (_modeInproc) {
if (item.getValue(Object.class) instanceof byte[]) {
if (CacheItemWrapperInternal.getFlagValue(item).IsBitSet((byte) BitSetConstants.Compressed) == true) {
try {
item.setValue(CompressionUtil.Decompress((byte[]) item.getValue(Object.class)));
} catch (Exception e) {
}
}
}
item.setValue(CacheHelper.DecryptData((byte[]) item.getValue(Object.class), _syncCache.getSerializationContext()));
item.setValue(_syncCache.getDeserializedObject(item.getValue(Object.class), _syncCache.getSerializationContext(), CacheItemWrapperInternal.getFlagValue(item)));
}
}
return item.getValue(Object.class);
//return val;
}
///
/// Registers the Sync Key notification with the cache.
///
/// key
/// eventlistener to be notified.
@Override
public void RegisterSyncKeyNotifications(String key, ISyncCacheEventsListener eventListener,ListenerType listenerType) throws GeneralFailureException, OperationFailedException {
try {
if (!_synEventListeners.contains(eventListener)) {
_synEventListeners.add(eventListener);
}
if (_syncCache != null && listenerType == ListenerType.PushBasedNotification) {
_syncCache.addCacheDataNotificationListener(new String[] { key }, _updateListener, EnumSet.of(EventType.ItemUpdated,EventType.ItemRemoved), EventDataFilter.None, true);
}
} catch (Exception e) {
throw new OperationFailedException(e.getMessage());
}
}
///
/// Unregister the snckey notifications.
///
/// key
/// eventlistener to unregister.
@Override
public void UnRegisterSyncKeyNotifications(String key, ISyncCacheEventsListener eventListener) throws GeneralFailureException, OperationFailedException {
if (!_synEventListeners.contains(eventListener)) {
_synEventListeners.remove(eventListener);
}
}
///
/// CacheId
///
public String getCacheId() {
return this._cacheId;
}
@Override
public void setCacheId(String value) {
throw new UnsupportedOperationException("Not supported yet.");
}
///
/// Initializes the SyncCache.
///
@Override
public void Initialize(String userId, String password) throws Exception {
try {
CacheConnectionOptions cacheConnectionOptions = new CacheConnectionOptions();
if (isNullOrEmpty(_server) && _port > 0) {
cacheConnectionOptions.getServerList().add(new ServerInfo(_server, _port));
}
cacheConnectionOptions.setLoadBalance(true);
if (!isNullOrEmpty(userId) && !isNullOrEmpty(password)) {
cacheConnectionOptions.setUserCredentials(new Credentials(userId, password));
}
_syncCache = CacheManager.getCache(_cacheId, cacheConnectionOptions, false, false);
_updateListener = this;
_removedListener = this;
_cacheClearListener = this;
cacheStoppedListener = cacheName -> onCacheStopped(cacheName);
pollNotificationListener = () -> onPollNotified();
_synEventListeners = new ArrayList();
if (_syncCache != null) {
_syncCache.setExceptionEnabled(true);
_syncCache.getNotificationService().addCacheClearedListener(_cacheClearListener);
}
} catch (Exception ex) {
throw new Exception("Unable to initialize " + _cacheId + " while creating CacheSyncDependency", ex);
}
}
// public void cacheDataModified(String key, CacheEventArg eventArgs) {
//
// if (eventArgs != null) {
// switch (eventArgs.getEventType()) {
// case ItemUpdated:
// itemChangedListener(key, eventArgs);
// break;
//
// case ItemRemoved:
// itemRemovedListener(key, eventArgs);
// break;
//
// }
// }
//
// }
///
/// Disposes this SyncCache Instance.
///
public void Dispose() {
if (_syncCache != null) {
DisposeInternal(_syncCache);
}
}
@Override
public void RegisterBulkSyncKeyNotifications(String[] keys, ISyncCacheEventsListener eventListener, ListenerType listenerType) throws GeneralFailureException, OperationFailedException {
try {
if (!_synEventListeners.contains(eventListener)) {
_synEventListeners.add(eventListener);
}
if (_syncCache != null&& listenerType == ListenerType.PushBasedNotification) {
_syncCache.addCacheDataNotificationListener(keys, _updateListener, EnumSet.of(EventType.ItemUpdated,EventType.ItemRemoved), EventDataFilter.None, true);
}
} catch (Exception e) {
throw new OperationFailedException(e.getMessage());
}
}
@Override
public void UnRegisterBulkSyncKeyNotifications(String key, ISyncCacheEventsListener eventListener) throws GeneralFailureException, OperationFailedException {
if (!_synEventListeners.contains(eventListener)) {
_synEventListeners.remove(eventListener);
}
}
@Override
public ListenerType GetNotificationType(String cacheId) {
return ListenerType.PushBasedNotification;
}
@Override
public void IsAuthorized(String cacheID, String password, String userId) throws OperationFailedException {
if(cacheID==null)
throw new OperationFailedException(ErrorCodes.CacheInit.CACHE_ID_NULL, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.CACHE_ID_NULL));
if(!_syncCache.checkUserAuthorization(cacheID.toLowerCase(),password,userId))
throw new SecurityException("You do not have permissions to initialize this cache");
}
@Override
public void PerformTouch(ArrayList keys) throws OperationNotSupportedException, CommandException, GeneralFailureException, StreamNotFoundException, ConfigurationException, StreamException, AggregateException, OperationFailedException, StreamAlreadyLockedException, LicensingException, com.alachisoft.ncache.runtime.exceptions.SecurityException {
_syncCache.touch(keys);
}
private void DisposeInternal(Object param) {
try {
((Cache) param).close();
} catch (Exception e) {
}
}
@Override
public void serialize(NCacheObjectOutput writer) throws IOException {
writer.writeObject(_cacheId);
writer.writeObject(_server);
writer.writeInt(_port);
}
@Override
public void deserialize(NCacheObjectInput reader) throws IOException, ClassNotFoundException {
Object tempVar = reader.readObject();
_cacheId = (String) ((tempVar instanceof String) ? tempVar : null);
Object tempVar2 = reader.readObject();
_server = (String) ((tempVar2 instanceof String) ? tempVar2 : null);
_port = reader.readInt();
}
public void cacheItemAdded(CacheEvent e) {
throw new UnsupportedOperationException("Not supported yet.");
}
public void cacheItemRemoved(CacheEvent e) {
throw new UnsupportedOperationException("Not supported yet.");
}
public void cacheItemUpdated(CacheEvent e) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public void onCacheCleared() {
cacheCleared();
}
@Override
public void onCacheStopped(String cacheName) {
if (_synEventListeners != null) {
for (Object eventListener : _synEventListeners) {
((ISyncCacheEventsListener) eventListener).CacheStopped(cacheName);
}
}
}
@Override
public void onPollNotified() {
if (_synEventListeners != null) {
for (Object eventListener : _synPollEventListeners) {
((ISyncCacheEventsListener) eventListener).PollEventNotified();
}
}
}
@Override
public void onCacheStatusChanged(ClusterEvent event) {
if(event.getEventType()== CacheStatusNotificationType.CacheStopped){
if (_synEventListeners != null) {
for (Object eventListener : _synEventListeners) {
((ISyncCacheEventsListener) eventListener).CacheStopped(event.getCacheId());
}
}
}
if(event.getEventType()== CacheStatusNotificationType.MemberJoined){
}
if(event.getEventType()== CacheStatusNotificationType.MemberLeft){
}
}
@Override
public void onCacheDataModified(String key, CacheEventArg eventArgs) {
if (eventArgs != null) {
switch (eventArgs.getEventType()) {
case ItemUpdated:
itemChangedListener(key, eventArgs);
break;
case ItemRemoved:
itemRemovedListener(key, eventArgs);
break;
}
}
}
@Override
public void onCacheCleared(String cacheName) {
cacheCleared();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy