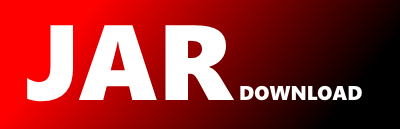
com.alachisoft.ncache.client.internal.command.CollectionCommandBase Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.command;
import com.alachisoft.ncache.common.protobuf.CollectionCommandProtocol;
import com.alachisoft.ncache.common.protobuf.CommandProtocol;
import com.alachisoft.ncache.common.protobuf.LockInfoProtocol;
import com.alachisoft.ncache.runtime.caching.DistributedDataStructure;
import com.alachisoft.ncache.runtime.caching.WriteThruOptions;
import com.alachisoft.ncache.runtime.util.TimeSpan;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public abstract class CollectionCommandBase extends Command {
protected String _lockId;
protected TimeSpan _lockTimeout;
protected WriteThruOptions _writeThruOption;
protected CollectionCommandProtocol.CollectionCommand.Builder _collectionCommandBuilder;
@Override
protected short getCommandHandle()
{
return (short) CommandProtocol.Command.Type.COLLECTION_COMMAND.getNumber();
}
@Override
protected void serializeCommandInternal(ByteArrayOutputStream stream) throws IOException {
_collectionCommandBuilder.build().writeTo(stream);
}
@Override
protected void createCommand()
{
_collectionCommandBuilder = CollectionCommandProtocol.CollectionCommand.newBuilder();
_collectionCommandBuilder.setRequestId(super.getRequestId());
_collectionCommandBuilder.setName(key);
LockInfoProtocol.LockInfo.Builder lockBuilder = LockInfoProtocol.LockInfo.newBuilder();
if(_lockId != null)
lockBuilder.setLockId(_lockId);
if(_lockTimeout != null)
lockBuilder.setLockTimeout(_lockTimeout.getTotalTicks());
_collectionCommandBuilder.setLockInfo(lockBuilder);
if (_writeThruOption != null)
{
_collectionCommandBuilder.setDsWriteOption(_writeThruOption.getMode().getValue());
if(_writeThruOption.getProviderName() != null) _collectionCommandBuilder.setProviderName(_writeThruOption.getProviderName());
}
}
protected static CollectionCommandProtocol.CollectionCommand.Type getCollectionType(DistributedDataStructure _collectionType)
{
switch (_collectionType)
{
case List:
return CollectionCommandProtocol.CollectionCommand.Type.LIST;
case Queue:
return CollectionCommandProtocol.CollectionCommand.Type.QUEUE;
case Map:
return CollectionCommandProtocol.CollectionCommand.Type.DICTIONARY;
case Set:
return CollectionCommandProtocol.CollectionCommand.Type.SET;
}
throw new RuntimeException("Invalid data type specified.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy