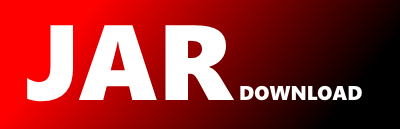
com.alachisoft.ncache.client.internal.command.ContainsBulkCommand Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.command;
import com.alachisoft.ncache.common.protobuf.CommandProtocol;
import com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol;
import com.alachisoft.ncache.common.protobuf.ContainsCommandProtocol;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ContainsBulkCommand extends Command{
private final int _methodOverload;
private String[] keys;
protected com.alachisoft.ncache.common.protobuf.ContainBulkCommandProtocol.ContainsBulkCommand _commandInstance;
public ContainsBulkCommand(String[] keys , int methodOverload) {
super.name = "ContainsBulkCommand";
if (keys == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: keys");
}
if (keys.length == 0) {
throw new IllegalArgumentException(
"There is no key present in keys array");
}
this.keys = keys;
this._methodOverload = methodOverload;
}
protected void createCommand() throws CommandException {
ContainBulkCommandProtocol.ContainsBulkCommand.Builder
builder = ContainBulkCommandProtocol.ContainsBulkCommand.newBuilder();
_commandInstance = builder
.setRequestId(super.getRequestId())
.addAllKeys(Arrays.asList(keys))
.setMethodOverload(_methodOverload)
.build();
}
@Override
public CommandType getCommandType()
{
return CommandType.CONTAINS_BULK;
}
@Override
public RequestType getCommandRequestType()
{
return RequestType.KeyBulkRead;
}
protected void serializeCommandInternal(ByteArrayOutputStream stream) throws IOException
{
_commandInstance.writeTo(stream);
}
@Override
protected short getCommandHandle()
{
return (short)CommandProtocol.Command.Type.CONTAINS_BULK.getNumber();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy