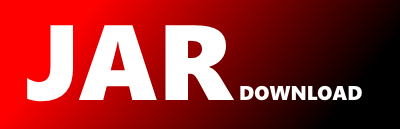
com.alachisoft.ncache.client.internal.command.DeleteQueryCommand Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client.internal.command;
import com.alachisoft.ncache.client.internal.util.IndexHelper;
import com.alachisoft.ncache.client.internal.util.QueryCommandHelper;
import com.alachisoft.ncache.common.protobuf.CommandProtocol;
import com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol;
import com.alachisoft.ncache.common.protobuf.KeyValueProtocol;
import com.alachisoft.ncache.common.protobuf.ValueWithTypeProtocol;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import com.alachisoft.ncache.runtime.util.HelperFxn;
import com.alachisoft.ncache.runtime.util.NCDateTime;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.*;
public final class DeleteQueryCommand extends Command {
private String query;
private HashMap values;
private boolean isRemove;
private int _methodOverload;
protected com.alachisoft.ncache.common.protobuf.DeleteQueryCommandProtocol.DeleteQueryCommand _commandInstance;
//private DeleteQueryCommand _deleteQueryCommand;
public DeleteQueryCommand(String query, java.util.HashMap values, boolean isRemove , int methodOverload) {
this.query = query;
this.values = values;
this.isRemove = isRemove;
_methodOverload = methodOverload;
}
@Override
public CommandType getCommandType() {
return CommandType.DELETEQUERY;
}
@Override
protected void createCommand() throws CommandException {
DeleteQueryCommandProtocol.DeleteQueryCommand.Builder builder =
DeleteQueryCommandProtocol.DeleteQueryCommand.newBuilder();
builder.setQuery(query)
.setIsRemove(isRemove)
.setMethodOverload(_methodOverload);
java.util.List valuesToSend = QueryCommandHelper.getValuesFromMap(values);
if(valuesToSend!=null){
builder.addAllValues(valuesToSend);
}
_commandInstance = builder.setRequestId(super.getRequestId())
.setCommandVersion(1)
.setClientLastViewId(super.getClientLastViewId()).build();
}
@Override
public boolean getIsSafe() {
return false;
}
@Override
public RequestType getCommandRequestType() {
return RequestType.NonKeyBulkWrite;
}
@Override
protected void serializeCommandInternal(ByteArrayOutputStream stream) throws IOException
{
_commandInstance.writeTo(stream);
}
@Override
protected short getCommandHandle()
{
return (short)CommandProtocol.Command.Type.DELETEQUERY.getNumber();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy