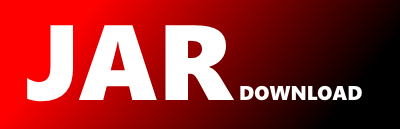
com.alachisoft.ncache.client.internal.command.DictionaryAddCommand Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.command;
import Alachisoft.NCache.Common.DataTypes.Dictionary.DictionaryAddOperation;
import Alachisoft.NCache.Common.JSON.ExtendedJsonValueBase;
import com.alachisoft.ncache.common.protobuf.CollectionItemProtocol;
import com.alachisoft.ncache.common.protobuf.DictionaryAddProtocol;
import com.alachisoft.ncache.common.protobuf.DictionaryCommandProtocol;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import com.google.protobuf.ByteString;
import java.util.Map;
public class DictionaryAddCommand extends DictionaryCommandBase {
private DictionaryAddOperation.OpType _operationType;
private Map _collection;
private DictionaryAddProtocol.DictionaryAdd.Builder _dictionaryAddBuilder;
public DictionaryAddCommand(DictionaryAddOperation operation) {
super.name = "DictionaryAdd";
super.key = operation.getName();
_writeThruOption = operation.getWriteThruOptions();
_lockId = (String)operation.getLockId();
_lockTimeout = operation.getLockTimeout();
_operationType = operation.getOperationType();
_collection = operation.getCollection();
}
@Override
public CommandType getCommandType() {
return CommandType.DATA_TYPE;
}
@Override
public RequestType getCommandRequestType() {
return RequestType.KeyBulkWrite;
}
@Override
protected void createCommand() throws CommandException {
_dictionaryAddBuilder = DictionaryAddProtocol.DictionaryAdd.newBuilder();
_dictionaryAddBuilder.addAllKeys(_collection.keySet());
for (ExtendedJsonValueBase value : _collection.values())
{
CollectionItemProtocol.CollectionItem.Builder valueItemBuilder = CollectionItemProtocol.CollectionItem.newBuilder();
for(byte[] bytes : value.toUserBinaryObject().getDataList())
valueItemBuilder.addData(ByteString.copyFrom(bytes));
_dictionaryAddBuilder.addValues(valueItemBuilder.build());
}
switch (_operationType)
{
case Add:
_dictionaryAddBuilder.setOperationType(DictionaryAddProtocol.DictionaryAdd.OperationType.Add);
break;
case Insert:
_dictionaryAddBuilder.setOperationType(DictionaryAddProtocol.DictionaryAdd.OperationType.Insert);
break;
}
super.createCommand();
_dictionaryCommandBuilder.setType(DictionaryCommandProtocol.DictionaryCommand.Type.DICTIONARY_ADD);
_dictionaryCommandBuilder.setDictionaryAdd(_dictionaryAddBuilder);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy