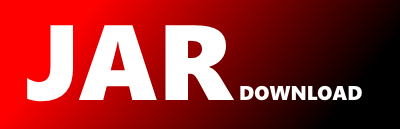
com.alachisoft.ncache.client.internal.command.DictionaryRemoveCommand Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.command;
import Alachisoft.NCache.Common.Caching.UserBinaryObject;
import Alachisoft.NCache.Common.DataTypes.Dictionary.DictionaryRemoveOperation;
import Alachisoft.NCache.Common.JSON.ExtendedJsonValueBase;
import com.alachisoft.ncache.common.protobuf.CollectionItemProtocol;
import com.alachisoft.ncache.common.protobuf.DictionaryCommandProtocol;
import com.alachisoft.ncache.common.protobuf.DictionaryRemoveProtocol;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import com.google.protobuf.ByteString;
import java.nio.charset.StandardCharsets;
import java.util.List;
import java.util.Map;
public class DictionaryRemoveCommand extends DictionaryCommandBase {
private List _collection;
private Map.Entry _keyValuePair;
private DictionaryRemoveOperation.OpType _operationType;
private DictionaryRemoveProtocol.DictionaryRemove.Builder _dictionaryRemoveBuilder;
public DictionaryRemoveCommand(DictionaryRemoveOperation operation)
{
super.name = "DictionaryRemove";
super.key = operation.getName();
_writeThruOption = operation.getWriteThruOptions();
_lockId = (String)operation.getLockId();
_lockTimeout = operation.getLockTimeout();
_operationType = operation.getOperationType();
_collection = operation.getCollection();
_keyValuePair = operation.getKeyValue();
}
@Override
public RequestType getCommandRequestType()
{
return RequestType.AtomicRead;
}
@Override
public CommandType getCommandType()
{
return CommandType.DATA_TYPE;
}
@Override
protected void createCommand() throws CommandException {
_dictionaryRemoveBuilder = DictionaryRemoveProtocol.DictionaryRemove.newBuilder();
switch (_operationType)
{
case Remove:
_dictionaryRemoveBuilder.addAllCollection(_collection);
_dictionaryRemoveBuilder.setOperationType(DictionaryRemoveProtocol.DictionaryRemove.OperationType.Remove);
break;
case KeyValueRemove:
_dictionaryRemoveBuilder.setKey(_keyValuePair.getKey());
if (_keyValuePair.getValue() != null)
{
UserBinaryObject valueObject = UserBinaryObject.createUserBinaryObject(_keyValuePair.getValue().toJson().getBytes(StandardCharsets.UTF_8));
CollectionItemProtocol.CollectionItem.Builder valueItemBuilder = CollectionItemProtocol.CollectionItem.newBuilder();
for(byte[] bytes : valueObject.getDataList())
valueItemBuilder.addData(ByteString.copyFrom(bytes));
_dictionaryRemoveBuilder.setValue(valueItemBuilder);
}
_dictionaryRemoveBuilder.setOperationType(DictionaryRemoveProtocol.DictionaryRemove.OperationType.KeyValueRemove);
break;
}
super.createCommand();
_dictionaryCommandBuilder.setType(DictionaryCommandProtocol.DictionaryCommand.Type.DICTIONARY_REMOVE);
_dictionaryCommandBuilder.setDictionaryRemove(_dictionaryRemoveBuilder);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy