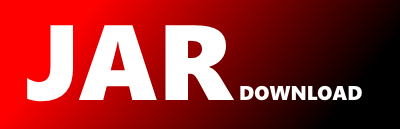
com.alachisoft.ncache.client.internal.command.GetCollectionChunkCommand Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.command;
import Alachisoft.NCache.Common.DataTypes.GetCollectionChunkOperation;
import Alachisoft.NCache.Common.DataTypes.GetDictionaryChunkOperation;
import com.alachisoft.ncache.common.protobuf.CollectionCommandProtocol;
import com.alachisoft.ncache.common.protobuf.CollectionGetChunkProtocol;
import com.alachisoft.ncache.runtime.caching.DistributedDataStructure;
public class GetCollectionChunkCommand extends CollectionCommandBase {
private DistributedDataStructure _collectionType;
int _index;
int _version;
private GetCollectionChunkOperation _operation;
private CollectionGetChunkProtocol.CollectionGetChunk.Builder _collectionGetChunkBuilder;
public GetCollectionChunkCommand(GetCollectionChunkOperation operation)
{
super.name = "CollectionGetChunk";
super.key = operation.getName();
_lockId = (String)operation.getLockId();
_lockTimeout = operation.getLockTimeout();
_collectionType = operation.getType();
_index = operation.getIndex();
_version = operation.getVersion();
_operation = operation;
}
@Override
public RequestType getCommandRequestType()
{
return RequestType.KeyBulkRead;
}
@Override
public CommandType getCommandType()
{
return CommandType.DATA_TYPE;
}
@Override
protected void createCommand()
{
_collectionGetChunkBuilder = CollectionGetChunkProtocol.CollectionGetChunk.newBuilder();
_collectionGetChunkBuilder.setIndex(_index);
_collectionGetChunkBuilder.setVersion(_version);
if (_collectionType == DistributedDataStructure.Map)
{
GetDictionaryChunkOperation getDictionaryChunkOperation = (GetDictionaryChunkOperation)((_operation instanceof GetDictionaryChunkOperation) ? _operation : null);
_collectionGetChunkBuilder.setKeyValuePairedEnumerationFilter( getDictionaryChunkOperation == null ? _collectionGetChunkBuilder.getKeyValuePairedEnumerationFilter() : getDictionaryChunkOperation.getFilter().getValue() );
}
super.createCommand();
_collectionCommandBuilder.setMethod(CollectionCommandProtocol.CollectionCommand.Method.GET_CHUNK);
_collectionCommandBuilder.setCollectionGetChunk(_collectionGetChunkBuilder);
_collectionCommandBuilder.setType(getCollectionType(_collectionType));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy