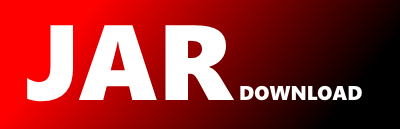
com.alachisoft.ncache.client.internal.command.HybridBulkCommand Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client.internal.command;
import Alachisoft.NCache.Common.BitSet;
import Alachisoft.NCache.Common.BitSetConstants;
import Alachisoft.NCache.Common.CompressionUtil;
import Alachisoft.NCache.Common.DataStructures.BridgeOperation;
import com.alachisoft.ncache.common.protobuf.CommandProtocol;
import com.alachisoft.ncache.common.protobuf.HybridBulkCommandProtocol;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import com.alachisoft.ncache.serialization.standard.CompactBinaryFormatter;
import com.google.protobuf.ByteString;
import java.io.IOException;
/**
* @author Basit Anwer
*/
public class HybridBulkCommand extends Command {
//private Alachisoft.NCache.Common.Protobuf.HybridBulkCommand _hybridBulkCommand;
private byte[] data;
private int flags;
public HybridBulkCommand(BridgeOperation[] bridgeOperations, boolean compress, String cacheContext) {
this.name = "HybridBulkCommand";
byte[] buffer = null;
BitSet flagMap = null;
super.setBridgeOperations(bridgeOperations);
try {
buffer = CompactBinaryFormatter.toByteBuffer(bridgeOperations, cacheContext);
flagMap = new BitSet();
tangible.RefObject tempRef_flagMap = new tangible.RefObject(flagMap);
if (compress) {
buffer = CompressionUtil.Compress(buffer, flagMap);
}
} catch (IOException ioE) {
// System.out.println("Error: " +ioE.getMessage());
}
if (buffer != null) {
this.data = buffer;
}
if (flagMap != null) {
this.flags = BitSetConstants.getBitSetData(flagMap);
}
// _hybridBulkCommand = new Alachisoft.NCache.Common.Protobuf.HybridBulkCommand();
// _hybridBulkCommand.data.Add(data);
// _hybridBulkCommand.flag = flags.Data;
// _hybridBulkCommand.requestId = base.RequestId;
}
@Override
public com.alachisoft.ncache.client.internal.command.CommandType getCommandType() {
return CommandType.HYBRID_BULK;
}
@Override
protected void createCommand() throws CommandException {
HybridBulkCommandProtocol.HybridBulkCommand.Builder builder =
HybridBulkCommandProtocol.HybridBulkCommand.newBuilder();
builder.addData(ByteString.copyFrom(data))
.setFlag(this.flags)
.setRequestId(this.getRequestId());
CommandProtocol.Command.Builder commandBuilder =
CommandProtocol.Command.newBuilder();
try {
commandBuilder = commandBuilder.setHybridBulkCommand(builder)
.setRequestID(this.getRequestId())
.setType(CommandProtocol.Command.Type.HYBRID_BULK);
super.commandBytes = this.constructCommand(commandBuilder.build().toByteArray());
} catch (IOException ex) {
throw new CommandException(ex.getMessage());
}
}
@Override
public RequestType getCommandRequestType() {
return RequestType.KeyBulkRead;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy