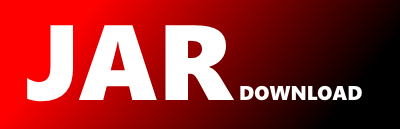
com.alachisoft.ncache.client.internal.command.MessagePublishBulkCommand Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.command;
import Alachisoft.NCache.Caching.Messaging.Message;
import Alachisoft.NCache.Common.Caching.UserBinaryObject;
import com.alachisoft.ncache.common.protobuf.CommandProtocol;
import com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol;
import com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import com.google.protobuf.ByteString;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
public class MessagePublishBulkCommand extends Command {
public com.alachisoft.ncache.common.protobuf.MessagePublishBulkCommandProtocol.MessagePublishBulkCommand _commandInstance;
MessagePublishBulkCommandProtocol.MessagePublishBulkCommand.Builder messageBulkCommand;
public MessagePublishBulkCommand(String topicName, Collection serverMessages, boolean notifyDeliveryFailure)
{
// set command name
messageBulkCommand = MessagePublishBulkCommandProtocol.MessagePublishBulkCommand.newBuilder();
name = "PublishMessageBulkCommand";
// initialize protobuf command
messageBulkCommand.setTopicName(topicName);
messageBulkCommand.setNotifyDeliveryFailure(notifyDeliveryFailure);
// initialize single publish command for each message and add to bulk command
for (Message message : serverMessages)
{
UserBinaryObject ubObject = UserBinaryObject.createUserBinaryObject((byte[])message.getPayLoad());
List dataList = ubObject.getDataList();
int noOfChunks = dataList.size();
///Copy the chunks to protobuf list
List dataChunks = new ArrayList();
for (int i = 0; i < noOfChunks; i++) {
dataChunks.add(ByteString.copyFrom(dataList.get(i)));
}
MessagePublishCommandProtocol.MessagePublishCommand.Builder messageCommand = MessagePublishCommandProtocol.MessagePublishCommand.newBuilder();
messageCommand.setMessageId( message.getMessageId())
.addAllData(dataChunks)
.setFlag(message.getFlagMap().getData())
.setExpiration(message.getMessageMetaData().getExpirationTime())
.setCreationTime(message.getCreationTime().getTime())
.setDeliveryOption(message.getMessageMetaData().getDeliveryOption().getValue());
messageBulkCommand.addMessagePublishCommand(messageCommand);
}
}
@Override
public CommandType getCommandType() {
return CommandType.PUBLISHMESSAGEBULK;
}
@Override
public RequestType getCommandRequestType() {
return RequestType.AtomicWrite;
}
@Override
public boolean getIsSafe() {
return false;
}
@Override
protected void serializeCommandInternal(ByteArrayOutputStream stream) throws IOException
{
_commandInstance.writeTo(stream);
}
@Override
protected short getCommandHandle()
{
return (short) CommandProtocol.Command.Type.MESSAGE_PUBLISH_BULK.getNumber();
}
@Override
protected void createCommand() throws CommandException {
if(this.getIntendedRecipient() != null){
messageBulkCommand.setIntendedRecipient(this.getIntendedRecipient());
}
messageBulkCommand
.setClientLastViewId(getClientLastViewId())
.setVersion(VERSION)
.setCommandVersion(1)
.setRequestId(getRequestId());
_commandInstance=messageBulkCommand.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy