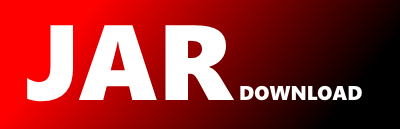
com.alachisoft.ncache.client.internal.command.MessagePublishCommand Maven / Gradle / Ivy
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client.internal.command;
import Alachisoft.NCache.Common.BitSet;
import com.alachisoft.ncache.common.protobuf.CommandProtocol;
import com.alachisoft.ncache.common.protobuf.KeyValuePairProtocol;
import com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import Alachisoft.NCache.Common.Caching.UserBinaryObject;
import com.google.protobuf.ByteString;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.*;
public class MessagePublishCommand extends Command {
private final byte[] payLoad;
private final long creationTime;
private final long expirationTime;
private final Hashtable metadata;
private final BitSet flagMap;
protected com.alachisoft.ncache.common.protobuf.MessagePublishCommandProtocol.MessagePublishCommand _commandInstance;
public MessagePublishCommand(String messageId, byte[] payLoad, long creationTime, long expirationTime, Hashtable metadata, BitSet flagMap) {
name = "PublishMessageCommand";
key = messageId;
this.payLoad = payLoad;
this.creationTime = creationTime;
this.expirationTime = expirationTime;
this.metadata = metadata;
this.flagMap = flagMap;
}
@Override
public CommandType getCommandType() {
return CommandType.PUBLISHMESSAGE;
}
@Override
public RequestType getCommandRequestType() {
return RequestType.AtomicWrite;
}
@Override
protected void createCommand() throws CommandException {
///Get user data and add to protobuf data array
UserBinaryObject userBin = UserBinaryObject.createUserBinaryObject(this.payLoad);
List dataList = userBin.getDataList();
int noOfChunks = dataList.size();
///Copy the chunks to protobuf list
List dataChunks = new ArrayList();
for (int i = 0; i < noOfChunks; i++) {
dataChunks.add(ByteString.copyFrom(dataList.get(i)));
}
MessagePublishCommandProtocol.MessagePublishCommand.Builder messageCommand = MessagePublishCommandProtocol.MessagePublishCommand.newBuilder();
messageCommand.setRequestId(getRequestId())
.setCreationTime(creationTime)
.addAllData(dataChunks)
.setExpiration(expirationTime)
.setFlag(flagMap.getData())
.setMessageId(key);
Set keys = metadata.keySet();
Iterator itr = keys.iterator();
while (itr.hasNext()) {
KeyValuePairProtocol.KeyValuePair.Builder keyValPair = KeyValuePairProtocol.KeyValuePair.newBuilder();
String key = itr.next();
keyValPair.setKey(key);
keyValPair.setValue((String) metadata.get(key));
messageCommand.addKeyValuePair(keyValPair.build());
}
if(this.getIntendedRecipient() != null){
messageCommand.setIntendedRecipient(this.getIntendedRecipient());
}
_commandInstance =messageCommand
.setClientLastViewId(getClientLastViewId())
.setVersion(VERSION)
.setCommandVersion(1)
.build();
}
@Override
public boolean getIsSafe() {
return false;
}
@Override
protected void serializeCommandInternal(ByteArrayOutputStream stream) throws IOException
{
_commandInstance.writeTo(stream);
}
@Override
protected short getCommandHandle()
{
return (short)CommandProtocol.Command.Type.MESSAGE_PUBLISH.getNumber();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy