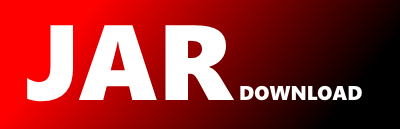
com.alachisoft.ncache.client.internal.command.OpenStreamCommand Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client.internal.command;
import Alachisoft.NCache.Common.Util.DependencyHelper;
import com.alachisoft.ncache.client.StreamMode;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import com.alachisoft.ncache.runtime.caching.expiration.ExpirationConstants;
import com.alachisoft.ncache.common.protobuf.CommandProtocol;
import com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol;
import com.alachisoft.ncache.runtime.CacheItemPriority;
import com.alachisoft.ncache.runtime.dependencies.CacheDependency;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import com.alachisoft.ncache.runtime.util.HelperFxn;
import com.alachisoft.ncache.runtime.util.TimeSpan;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.Date;
//import com.alachisoft.ncache.web.caching.StreamMode;
/**
* @author Asad Hasnain
*/
public final class OpenStreamCommand extends Command {
private OpenStreamCommandProtocol.OpenStreamCommand.Builder openStreamCommand;
private String _key;
private StreamMode _mode;
private String _group;
private Date _absExpiration;
private TimeSpan _slidingExpiration;
private CacheDependency _dependency;
private CacheItemPriority _priority;
private int _methodOverload;
protected com.alachisoft.ncache.common.protobuf.OpenStreamCommandProtocol.OpenStreamCommand _commandInstance;
public OpenStreamCommand(String key, StreamMode mode, String group, Date absoluteExpiration, TimeSpan slidingExpiration, CacheDependency dependency, CacheItemPriority priority , int methodOverload) {
if (key == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: key");
}
if (key.equals("")) {
throw new IllegalArgumentException("Value cannot be empty.\nParameter name: key");
}
if (slidingExpiration._ticks != 0 && absoluteExpiration != ExpirationConstants.AbsoluteNoneExpiration)
throw new IllegalArgumentException(
"You cannot set both sliding and absolute expirations on the same cached item");
_key = key;
_mode = mode;
_group = group;
_absExpiration = absoluteExpiration;
_slidingExpiration = slidingExpiration;
_dependency = dependency;
_priority = priority;
_methodOverload = methodOverload;
openStreamCommand = OpenStreamCommandProtocol.OpenStreamCommand.newBuilder();
}
public void createCommand() throws CommandException {
openStreamCommand.setKey(_key);
if (_group != null) {
openStreamCommand.setGroup(_group);
}
openStreamCommand.setStreamMode(_mode.ordinal());
long sldExp = 0, absExp = 0;
if (_absExpiration != CacheImpl.NoAbsoluteExpiration)
//absExp = ticks(_absExpiration);
absExp = HelperFxn.getUTCTicks(_absExpiration);
else
absExp = 0;
if (_slidingExpiration != CacheImpl.NoSlidingExpiration)
sldExp = _slidingExpiration.getTotalTicks();
else
sldExp = 0;
openStreamCommand.setAbsoluteExpiration(absExp);
openStreamCommand.setSlidingExpiration(sldExp);
openStreamCommand.setPriority(_priority.value());
if (_dependency != null) {
try {
openStreamCommand = openStreamCommand.setDependency(DependencyHelper.getDependencyProtocol(_dependency));
} catch (Exception ex) {
throw new CommandException(ex.getMessage());
}
}
_commandInstance =openStreamCommand
.setRequestId(this.getRequestId())
.setMethodOverload(_methodOverload)
.build();
}
public boolean parseCommand() {
return true;
}
public CommandType getCommandType() {
return CommandType.OPEN_STREAM;
}
@Override
public RequestType getCommandRequestType() {
return RequestType.AtomicWrite;
}
@Override
protected void serializeCommandInternal(ByteArrayOutputStream stream) throws IOException
{
_commandInstance.writeTo(stream);
}
@Override
protected short getCommandHandle()
{
return (short)CommandProtocol.Command.Type.OPEN_STREAM.getNumber();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy