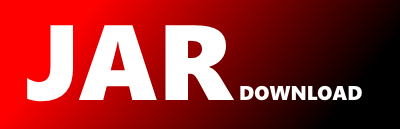
com.alachisoft.ncache.client.internal.command.SearchCQCommand Maven / Gradle / Ivy
/*
* Command.java
*
* Created on September 8, 2006, 11:45 AM
*
* Copyright 2005 Alachisoft, Inc. All rights reserved.
* ALACHISOFT PROPRIETARY/CONFIDENTIAL. Use is subject to license terms.
*/
package com.alachisoft.ncache.client.internal.command;
import com.alachisoft.ncache.common.protobuf.CommandProtocol;
import com.alachisoft.ncache.common.protobuf.KeyValueProtocol;
import com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol;
import com.alachisoft.ncache.common.protobuf.ValueWithTypeProtocol;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import com.alachisoft.ncache.runtime.util.HelperFxn;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.*;
/**
* @author Administrator
* @version 1.0
*/
public final class SearchCQCommand extends Command {
private static char Delimitor = '|';
private String query;
private HashMap values;
private boolean isSearchEntries;
private String clientUniqueId;
private boolean notifyAdd;
private boolean notifyUpdate;
private boolean notifyRemove;
private int addDF;
private int removeDF;
private int updateDF;
private int _methodOverload = 0;
protected com.alachisoft.ncache.common.protobuf.SearchCQCommandProtocol.SearchCQCommand _commandInstance;
/**
* Creates a new instance of SearchCommand
*
* @param query
* @throws java.io.UnsupportedEncodingException
* @throws java.io.IOException
*/
public SearchCQCommand(String query, HashMap values, String clientUniqueId,
boolean isSearchEntries, boolean notifyAdd, boolean notifyUpdate,
boolean notifyRemove, int addDF, int removeDF, int updateDF) {
this.query = query;
this.values = values;
this.isSearchEntries = isSearchEntries;
this.clientUniqueId = clientUniqueId;
this.notifyAdd = notifyAdd;
this.notifyRemove = notifyRemove;
this.notifyUpdate = notifyUpdate;
this.addDF = addDF;
this.removeDF = removeDF;
this.updateDF = updateDF;
}
public void createCommand() throws CommandException {
SearchCQCommandProtocol.SearchCQCommand.Builder builder =
SearchCQCommandProtocol.SearchCQCommand.newBuilder();
builder = builder.setRequestId(this.getRequestId())
.setQuery(this.query)
.setSearchEntries(this.isSearchEntries)
.setNotifyAdd(this.notifyAdd)
.setNotifyUpdate(this.notifyUpdate)
.setNotifyRemove(this.notifyRemove)
.setClientUniqueId(this.clientUniqueId)
.setAddDataFilter(addDF)
.setRemvoeDataFilter(removeDF)
.setUpdateDataFilter(updateDF);
int totalValuesCount = 0;
Set keySet = values.keySet();
Iterator iterator = keySet.iterator();
while (iterator.hasNext()) {
try {
String typeKey = (String) iterator.next();
KeyValueProtocol.KeyValue.Builder keyValPair =
KeyValueProtocol.KeyValue.newBuilder();
keyValPair = keyValPair.setKey(typeKey);
if (values.get(typeKey) instanceof ArrayList) {
ArrayList list = (ArrayList) values.get(typeKey);
for (int i = 0; i < list.size(); i++) {
Object typeValue = list.get(i);
keyValPair = keyValPair.addValue(ValueWithTypeProtocol.ValueWithType.newBuilder()
.setType(typeValue.getClass().getName())
.setValue(getValueString(typeValue)).build());
}
} else {
Object typeValue = values.get(typeKey);
keyValPair = keyValPair.addValue(ValueWithTypeProtocol.ValueWithType.newBuilder()
.setType(typeValue.getClass().getName()));
}
builder = builder.addValues(keyValPair);
} catch (Exception e) {
throw new CommandException("Error parsing values.");
}
}
_commandInstance =builder
.setClientLastViewId(getClientLastViewId())
.setMethodOverload(_methodOverload)
.build();
}
/**
* @return
*/
private String getValueString(Object value) throws Exception {
if (value == null) {
throw new CommandException("NCache query does not support null values");
}
if (value instanceof String) {
value = (Object) (value.toString()).toLowerCase();
}
if (value instanceof Date) {
value = HelperFxn.getTicks((Date) value);
}
return value.toString();
}
public CommandType getCommandType() {
return CommandType.SEARCH_CQ;
}
@Override
public RequestType getCommandRequestType() {
return RequestType.NonKeyBulkRead;
}
@Override
protected void serializeCommandInternal(ByteArrayOutputStream stream) throws IOException
{
_commandInstance.writeTo(stream);
}
@Override
protected short getCommandHandle()
{
return (short)CommandProtocol.Command.Type.SEARCH_CQ.getNumber();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy