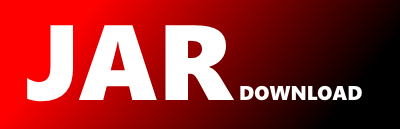
com.alachisoft.ncache.client.internal.command.UnlockCommand Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.command;
import com.alachisoft.ncache.common.protobuf.CommandProtocol;
import com.alachisoft.ncache.common.protobuf.UnlockCommandProtocol;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
public final class UnlockCommand extends Command {
private String _lockId;
private boolean _preemptive;
private int _methodOverload;
protected com.alachisoft.ncache.common.protobuf. UnlockCommandProtocol.UnlockCommand _commandInstance;
public UnlockCommand(String key , int methodOverload) {
super.name = "UnlockCommand";
super.key = key;
this._methodOverload = methodOverload;
this._preemptive = true;
}
public UnlockCommand(String key, String lockId , int methodOverload) {
super.name = "UnlockCommand";
super.key = key;
this._lockId = lockId;
this._preemptive = false;
this._methodOverload = methodOverload;
}
@Override
protected void createCommand() throws CommandException {
// String preemptive = this._preemptive ? "true" : "false";
this._lockId = (this._lockId == null) ? "" : this._lockId;
UnlockCommandProtocol.UnlockCommand.Builder builder =
UnlockCommandProtocol.UnlockCommand.newBuilder();
builder.setRequestId(this.getRequestId())
.setKey(key)
.setLockId(this._lockId)
.setPreemptive(this._preemptive);
_commandInstance =builder.setMethodOverload(_methodOverload).build();
}
public CommandType getCommandType() {
return CommandType.UNLOCK;
}
@Override
public RequestType getCommandRequestType() {
return RequestType.AtomicWrite;
}
@Override
protected void serializeCommandInternal(ByteArrayOutputStream stream) throws IOException
{
_commandInstance.writeTo(stream);
}
@Override
protected short getCommandHandle()
{
return (short)CommandProtocol.Command.Type.UNLOCK.getNumber();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy