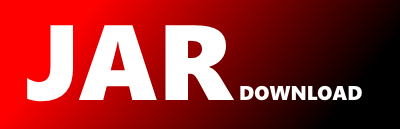
com.alachisoft.ncache.client.internal.communication.RPCTransport Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.communication;
import Alachisoft.NCache.Common.Communication.IChannelFormatter;
import Alachisoft.NCache.Common.Extensibility.Client.RPC.*;
import com.alachisoft.ncache.client.Cache;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import com.alachisoft.ncache.client.internal.caching.ClientCache;
import com.alachisoft.ncache.client.internal.caching.DisconnectedClientCache;
import com.alachisoft.ncache.client.internal.caching.InprocCache;
import com.alachisoft.ncache.runtime.exceptions.CommandException;
import com.alachisoft.ncache.runtime.exceptions.LicensingException;
import com.alachisoft.ncache.runtime.exceptions.OperationFailedException;
public class RPCTransport implements IRPCTransport {
public String module;
public String version;
private CacheImpl _cacheInstance;
private IRPCCallBuilder _builder;
public RPCTransport(Cache cache, IChannelFormatter formatter) {
if (cache == null) {
throw new NullPointerException("cache");
}
if (formatter == null) {
throw new NullPointerException("formatter");
}
if (_cacheInstance.getCacheImpl() instanceof InprocCache) {
throw new UnsupportedOperationException("Local InProc topology is not supported. ");
}
if (_cacheInstance instanceof DisconnectedClientCache)
{
this._cacheInstance = ((DisconnectedClientCache)_cacheInstance).getCacheInstanceInternal();
}
else if (_cacheInstance instanceof ClientCache)
{
this._cacheInstance = ((ClientCache)_cacheInstance).getCacheInstanceInternal();
}
else
{
this._cacheInstance = (CacheImpl) cache;
}
_builder = new DistributedCallBuilder(this, formatter);
}
public final PartitioningStrategy GetPartitioningStrategy() {
if (_cacheInstance.getCacheImpl() != null) {
return _cacheInstance.getCacheImpl().getPartitioningStrategy();
}
return null;
}
@Override
public final void ExecuteConstructor(IRPCConstructorCall constructor) throws Exception {
DistributedConstructorCall constructorCall = constructor instanceof DistributedConstructorCall ? (DistributedConstructorCall) constructor : null;
constructorCall.SetModule(this.module);
constructorCall.SetVersion(this.version);
constructorCall.PrepareCall();
constructorCall.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy