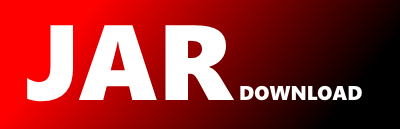
com.alachisoft.ncache.client.internal.datastructure.CounterImpl Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.datastructure;
import Alachisoft.NCache.Common.DataTypes.Counter.CounterOperation;
import com.alachisoft.ncache.client.datastructures.Counter;
import com.alachisoft.ncache.runtime.caching.WriteThruOptions;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import com.alachisoft.ncache.runtime.caching.DistributedDataStructure;
import com.alachisoft.ncache.runtime.events.DataStructureDataChangeListener;
import com.alachisoft.ncache.runtime.events.DataTypeEventDataFilter;
import com.alachisoft.ncache.runtime.events.EventType;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import java.util.EnumSet;
class CounterImpl extends DistributedDataStructureImpl implements Counter {
// region ---------- Constructors ----------
public CounterImpl(String key, CacheImpl cache, WriteThruOptions options,Class> cls) {
super(key, cache, options,cls);
}
// endregion
@Override
public long setValue(long value) {
return getCache().counterOperations(getKey(), _lockHandle.getLockId(), _lockTimeout, value, CounterOperation.OpType.SetValue, getWriteThruOptions());
}
@Override
public long getValue() {
return getCache().counterGetOperation(getKey(), _lockHandle.getLockId(), _lockTimeout);
}
@Override
public long increment() {
return getCache().counterOperations(getKey(), _lockHandle.getLockId(), _lockTimeout, 1, CounterOperation.OpType.IncrementBy, getWriteThruOptions());
}
@Override
public long decrement() {
return getCache().counterOperations(getKey(), _lockHandle.getLockId(), _lockTimeout, 1, CounterOperation.OpType.DecrementBy, getWriteThruOptions());
}
@Override
public long incrementBy(long value) {
if (value == 0) {
throw new IllegalArgumentException("value cannot be " + value);
}
return getCache().counterOperations(getKey(), _lockHandle.getLockId(), _lockTimeout, value, CounterOperation.OpType.IncrementBy, getWriteThruOptions());
}
@Override
public long decrementBy(long value) {
if (value == 0) {
throw new IllegalArgumentException("value cannot be " + value);
}
return getCache().counterOperations(getKey(), _lockHandle.getLockId(), _lockTimeout, value, CounterOperation.OpType.DecrementBy, getWriteThruOptions());
}
@Override
protected DistributedDataStructure getDataType() {
return DistributedDataStructure.Counter;
}
@Override
public int compareTo(Object o) {
if (o == null) {
return -1;
}
if (Counter.class.isAssignableFrom(o.getClass())) {
return Long.compare(((Counter) o).getValue(), getValue());
}
if (long.class.isAssignableFrom(o.getClass())) {
return Long.compare((long) o, getValue());
}
return -1;
}
// region Notifications
@Override
public void addChangeListener(DataStructureDataChangeListener dataTypeDataChangeListener, EnumSet eventType, DataTypeEventDataFilter dataFilter) throws CacheException {
if ((eventType.contains(EventType.ItemAdded) || eventType.contains(EventType.ItemRemoved))) {
throw new IllegalArgumentException("Registration for Add and Remove event is not supported for this dataType.");
}
else {
super.addChangeListener(dataTypeDataChangeListener, eventType, dataFilter);
}
}
// endregion
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (Counter.class.isAssignableFrom(obj.getClass())) {
return ((Counter) obj).getValue() == getValue();
}
if (long.class.isAssignableFrom(obj.getClass())) {
return (long) obj == getValue();
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy