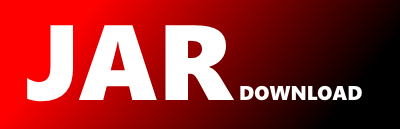
com.alachisoft.ncache.client.internal.datastructure.DistributedCollectionIteratorImpl Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.datastructure;
import Alachisoft.NCache.Common.BitSet;
import Alachisoft.NCache.Common.BitSetConstants;
import Alachisoft.NCache.Common.DataTypes.Responses.GetCollectionChunkResponse;
import Alachisoft.NCache.Common.DataTypes.Responses.GetCollectionChunkResponseBase;
import Alachisoft.NCache.Common.Enum.UserObjectType;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import com.alachisoft.ncache.runtime.caching.DistributedDataStructure;
import com.alachisoft.ncache.runtime.exceptions.*;
import com.alachisoft.ncache.runtime.exceptions.runtime.GeneralFailureRuntimeException;
import com.alachisoft.ncache.runtime.exceptions.runtime.OperationFailedRuntimeException;
import java.util.Iterator;
class DistributedCollectionIteratorImpl implements Iterator {
protected int _index;
protected String _key;
protected CacheImpl _cache;
int _size;
T _current;
private Class _valueType;
DistributedDataStructure _collectionType;
GetCollectionChunkResponseBase _response;
DistributedCollectionIteratorImpl(CacheImpl cache, String key, DistributedDataStructure collectionType,Class> cls) {
_key = key;
_cache = cache;
_index = _size = 0;
_current = null;
_valueType=cls;
_collectionType = collectionType;
}
protected boolean moveNextInternal(boolean peekOnly) throws ConnectionException, OperationFailedException, AggregateException, GeneralFailureException {
if (_index >= _size && !getNextAvailableChunk()) {
return MoveNextRare();
}
GetCollectionChunkResponse response = (GetCollectionChunkResponse) _response;
T temp;
try {
temp = _cache.safeDeserialize(response.getDataChunk().get(_index), _cache.getSerializationContext(), new BitSet((byte) BitSetConstants.JsonData), UserObjectType.DistributedDataType, _valueType);
} catch (CacheException e) {
return false;
}
if (peekOnly) {
return true;
}
_current = temp;
_index++;
return true;
}
protected boolean getNextAvailableChunk() throws ConnectionException, OperationFailedException, GeneralFailureException, AggregateException {
if (_response == null || !_response.getIsLastChunk()) {
_response = _cache.getCollectionChunkResponse(_key, _collectionType, _response!=null?_response.getVersion():0, _response!=null?_response.getNextIndex():0);
GetCollectionChunkResponse response = (GetCollectionChunkResponse) _response;
if (response.getDataChunk().size() == 0) {
return false;
}
_size = response.getDataChunk().size();
_index = 0;
return true;
} else {
return false;
}
}
boolean MoveNextRare() {
_index = _size + 1;
_current = null;
return false;
}
@Override
public boolean hasNext() {
try {
return moveNextInternal(true);
} catch (OperationFailedException | AggregateException e) {
throw new OperationFailedRuntimeException(e);
} catch (GeneralFailureException e) {
throw new GeneralFailureRuntimeException(e);
}
}
@Override
public T next() {
try {
moveNextInternal(false);
} catch (OperationFailedException | AggregateException e) {
throw new OperationFailedRuntimeException(e);
} catch (GeneralFailureException e) {
throw new GeneralFailureRuntimeException(e);
}
return _current;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy