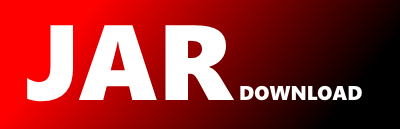
com.alachisoft.ncache.client.internal.datastructure.DistributedDataStructureImpl Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.datastructure;
import Alachisoft.NCache.Caching.Util.CollectionUtil;
import com.alachisoft.ncache.client.CacheItem;
import com.alachisoft.ncache.client.EventUtil;
import com.alachisoft.ncache.client.LockHandle;
import com.alachisoft.ncache.client.datastructures.DistributedDataStructure;
import com.alachisoft.ncache.client.internal.caching.*;
import Alachisoft.NCache.Common.ErrorHandling.ErrorCodes;
import Alachisoft.NCache.Common.ErrorHandling.ErrorMessages;
import com.alachisoft.ncache.runtime.caching.WriteMode;
import com.alachisoft.ncache.runtime.caching.WriteThruOptions;
import com.alachisoft.ncache.runtime.events.DataStructureDataChangeListener;
import com.alachisoft.ncache.runtime.events.DataTypeEventDataFilter;
import com.alachisoft.ncache.runtime.events.EventType;
import com.alachisoft.ncache.runtime.exceptions.*;
import com.alachisoft.ncache.runtime.exceptions.runtime.CacheRuntimeException;
import com.alachisoft.ncache.runtime.exceptions.runtime.GeneralFailureRuntimeException;
import com.alachisoft.ncache.runtime.exceptions.runtime.OperationFailedRuntimeException;
import com.alachisoft.ncache.runtime.util.TimeSpan;
import java.util.Date;
import java.util.EnumSet;
import java.util.List;
import java.util.Objects;
abstract class DistributedDataStructureImpl implements DistributedDataStructure {
// region Properties
protected Class _valueType;
protected TimeSpan _lockTimeout = new TimeSpan();
protected LockHandle _lockHandle;
private String _key;
private CacheImpl _cache;
private WriteThruOptions _writeThruOptions;
// endregion
// region Getters / Setters
DistributedDataStructureImpl(String key, CacheImpl cache, WriteThruOptions options,Class> cls) {
_key = key;
_cache = cache;
_lockTimeout = TimeSpan.MaxValue;
_valueType=cls;
_lockHandle = new LockHandle(null, new Date(Long.MAX_VALUE));
if (options == null)
{
_writeThruOptions = new WriteThruOptions(WriteMode.None, "");
}
else
{
_writeThruOptions = options;
}
}
@Override
public String getKey() {
return _key;
}
@Override
public WriteThruOptions getWriteThruOptions() {
return _writeThruOptions;
}
@Override
public void setWriteThruOptions(WriteThruOptions value) {
if (value == null) {
_writeThruOptions = new WriteThruOptions();
} else {
_writeThruOptions = new WriteThruOptions(value.getMode(), value.getProviderName());
}
}
@Override
public boolean lock(TimeSpan timeout) {
_lockTimeout = timeout;
_lockHandle = new LockHandle(_lockHandle);
boolean tempVar = false;
try {
tempVar = getCache().lock(getKey(), timeout, _lockHandle);
} catch (CacheException e) {
throw new CacheRuntimeException(e);
}
return tempVar;
}
@Override
public void unlock() {
try {
getCache().unlock(getKey(), _lockHandle);
} catch (CacheException e) {
throw new CacheRuntimeException(e);
}
_lockHandle = new LockHandle(null, new Date(Long.MAX_VALUE));
}
// endregion
// region Overridden methods of Collection
CacheImpl getCache() {
if (_cache == null)
throw new OperationFailedRuntimeException(ErrorCodes.CacheInit.CACHE_NOT_INIT, ErrorMessages.getErrorMessage(ErrorCodes.DataTypes.COUNT_NON_POSITIVE));
return _cache;
}
public int size() {
try {
return CountInternal(getDataType());
} catch (OperationFailedException | AggregateException e) {
throw new OperationFailedRuntimeException(e);
} catch (GeneralFailureException e) {
throw new GeneralFailureRuntimeException(e);
}
}
public void clear() {
try {
ClearInternal(getDataType());
}catch (OperationFailedException | AggregateException e) {
throw new OperationFailedRuntimeException(e);
} catch (GeneralFailureException e) {
throw new GeneralFailureRuntimeException(e);
}
}
// endregion
///#region ----------------- Constructors -----------------
public boolean isEmpty() {
return size() < 1;
}
///#endregion
///#region ---------------- Public Methods ----------------
public void addChangeListener(DataStructureDataChangeListener dataTypeDataChangeListener, EnumSet eventType, DataTypeEventDataFilter datafilter) throws CacheException
{
if (getCache() == null) {
throw new OperationFailedRuntimeException(ErrorCodes.CacheInit.CACHE_NOT_INIT, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.CACHE_NOT_INIT));
}
if (dataTypeDataChangeListener == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: dataTypeDataChangeListener");
}
short[] callbackRefs = getCache().getEventManager().registerCollectionEvent(dataTypeDataChangeListener, EventUtil.getEventTypeInternalEnumSet(eventType), datafilter);
getCache().addCollectionNotificationListener(_key, callbackRefs[0], callbackRefs[1], callbackRefs[2], datafilter, getDataType(), true);
}
public void removeChangeListener(DataStructureDataChangeListener dataTypeDataChangeListener, EnumSet eventType) throws Exception
{
if (getCache() == null) {
throw new OperationFailedRuntimeException(ErrorCodes.CacheInit.CACHE_NOT_INIT, ErrorMessages.getErrorMessage(ErrorCodes.CacheInit.CACHE_NOT_INIT));
}
if (dataTypeDataChangeListener == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: dataTypeDataChangeListener");
}
short[] callbackRefs = getCache().getEventManager().UnregisterCollectionNotification(dataTypeDataChangeListener, EventUtil.getEventTypeInternalEnumSet(eventType));
getCache().removeCollectionNotificationListener(_key, callbackRefs[0], callbackRefs[1], callbackRefs[2], EventUtil.getEventTypeInternal(eventType), getDataType());
}
///#endregion
///#region -------- Protected Methods & Properties --------
final void validateTypeNotCacheItem(java.lang.Class type) {
if (type.equals(CacheItem.class)) {
throw new OperationFailedRuntimeException(ErrorMessages.getErrorMessage(ErrorCodes.DataTypes.CACHEITEM_IN_DATA_STRUCTURES));
}
}
protected abstract com.alachisoft.ncache.runtime.caching.DistributedDataStructure getDataType();
protected final void ClearInternal(com.alachisoft.ncache.runtime.caching.DistributedDataStructure dataType) throws OperationFailedException, GeneralFailureException, AggregateException {
getCache().clearCollection(getKey(), dataType, _lockHandle.getLockId(), _lockTimeout);
}
final int CountInternal(com.alachisoft.ncache.runtime.caching.DistributedDataStructure dataType) throws OperationFailedException, GeneralFailureException, AggregateException {
return getCache().collectionCount(getKey(), dataType, _lockHandle.getLockId(), _lockTimeout);
}
final void CopyToInternal(Iterable source, T[] destination, int destinationStartIndex, int sourceCount) {
if (destination == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: destination");
}
if (!CollectionUtil.validateCopyToParams(destinationStartIndex, destination.length, sourceCount)) {
return;
}
for (T item : source) {
destination[destinationStartIndex++] = item;
}
}
protected final void CopyToInternal(Iterable source, List destination, int destinationStartIndex, int sourceCount) {
if (destination == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: destination");
}
if (!CollectionUtil.validateCopyToParams(destinationStartIndex, destination.size(), sourceCount)) {
return;
}
for (T item : source) {
destination.set(destinationStartIndex++, item);
}
}
///#endregion
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy