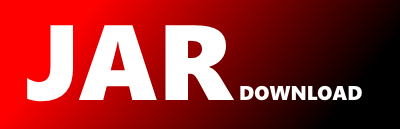
com.alachisoft.ncache.client.internal.datastructure.DistributedHashSetImpl Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.datastructure;
import Alachisoft.NCache.Caching.Util.CollectionUtil;
import Alachisoft.NCache.Common.DataTypes.CollectionCreateOrGetOperation;
import com.alachisoft.ncache.client.datastructures.DistributedHashSet;
import com.alachisoft.ncache.runtime.caching.ReadMode;
import com.alachisoft.ncache.runtime.caching.ReadThruOptions;
import com.alachisoft.ncache.runtime.caching.WriteThruOptions;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import Alachisoft.NCache.Common.ErrorHandling.ErrorCodes;
import Alachisoft.NCache.Common.ErrorHandling.ErrorMessages;
import com.alachisoft.ncache.runtime.caching.DistributedDataStructure;
import com.alachisoft.ncache.runtime.events.DataStructureDataChangeListener;
import com.alachisoft.ncache.runtime.events.DataTypeEventDataFilter;
import com.alachisoft.ncache.runtime.events.EventType;
import com.alachisoft.ncache.runtime.exceptions.*;
import com.alachisoft.ncache.runtime.exceptions.runtime.GeneralFailureRuntimeException;
import com.alachisoft.ncache.runtime.exceptions.runtime.OperationFailedRuntimeException;
import java.util.*;
class DistributedHashSetImpl extends DistributedDataStructureImpl implements DistributedHashSet {
public DistributedHashSetImpl(String key, CacheImpl cache, WriteThruOptions options,Class> cls) {
super(key, cache, options,cls);
}
@Override
protected DistributedDataStructure getDataType() {
return DistributedDataStructure.Set;
}
@Override
public void addRange(Collection collection) {
addOrInsertOperation((Collection extends T>) collection);
}
@Override
public T removeRandom() {
return removeRandomOperation();
}
@Override
public T getRandom() {
return getRandomOperation(1)[0];
}
@Override
public T[] getRandom(int count) {
return getRandomOperation(count);
}
@Override
public int remove(Collection items) {
return removeOperation(items);
}
@Override
public Collection union(String other) throws CacheException {
Set tmp = new HashSet<>(GetOtherSet(other,_valueType));
if (tmp == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: other");
tmp.addAll(this);
return tmp;
}
@Override
public Collection intersect(String other) throws CacheException {
Set tmp = new HashSet<>((GetOtherSet(other,_valueType)));
if (tmp == null)
throw new OperationFailedException(other+" does not exist.");
Set result = new HashSet<>();
for (T item : this) {
if (tmp.contains(item))
result.add(item);
}
return result;
}
@Override
public Collection difference(String other) throws CacheException {
Set otherHashSet = GetOtherSet(other,_valueType);
if (otherHashSet == null)
throw new OperationFailedException(other+" does not exist.");
HashSet setA= new HashSet(this);
setA.removeAll(otherHashSet);
return setA;
}
@Override
public DistributedHashSet storeUnion(String destination, String other) throws CacheException {
DistributedHashSet finalSet = GetOrCreateOtherSet(destination,_valueType);
DistributedHashSet otherHashSet = GetOtherSet(other,_valueType);
if (otherHashSet == null)
throw new OperationFailedException(other+" does not exist.");
finalSet.addRange(this.union(other));
return finalSet;
}
@Override
public DistributedHashSet storeDifference(String destination, String other) throws CacheException {
DistributedHashSet finalSet = GetOrCreateOtherSet(destination,_valueType);
DistributedHashSet otherHashSet = GetOtherSet(other,_valueType);
if (otherHashSet == null)
throw new OperationFailedException(other+" does not exist.");
finalSet.addRange(this.difference(other));
return finalSet;
}
@Override
public DistributedHashSet storeIntersection(String destination, String other) throws CacheException {
DistributedHashSet finalSet = GetOrCreateOtherSet(destination,_valueType);
DistributedHashSet otherHashSet = GetOtherSet(other,_valueType);
if (otherHashSet == null)
throw new OperationFailedException(other+" does not exist.");
finalSet.addRange(this.intersect(other));
return finalSet;
}
@Override
public boolean isEmpty() {
return size() < 1;
}
@Override
public boolean contains(Object o) {
try {
return containsOperation((T) o);
} catch (GeneralFailureException e) {
throw new GeneralFailureRuntimeException(e);
} catch (OperationFailedException | AggregateException e) {
throw new OperationFailedRuntimeException(e);
}
}
@Override
public Iterator iterator() {
return new DistributedCollectionIteratorImpl(getCache(), getKey(), DistributedDataStructure.Set,_valueType);
}
@Override
public Object[] toArray() {
int queueCount = size();
Object[] array = new Object[queueCount];
int x = 0;
for (T t : this) {
array[x++] = t;
}
return array;
}
@Override
public T1[] toArray(T1[] a) {
// implementation taken from ArrayList
int size = size();
Object[] elementData = toArray();
if (a.length < size)
// Make a new array of a's runtime type, but my contents:
return (T1[]) Arrays.copyOf(elementData, size, a.getClass());
System.arraycopy(elementData, 0, a, 0, size);
if (a.length > size)
a[size] = null;
return a;
}
@Override
public boolean add(T item) {
if (item == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: item");
HashSet hashSet = new HashSet();
hashSet.add(item);
addOrInsertOperation(hashSet);
return false;
}
@Override
public boolean remove(Object o) {
ArrayList arr = new ArrayList<>();
arr.add((T) o);
return removeOperation(arr) > 0;
}
@Override
public boolean containsAll(Collection> c) {
// TODO (IN FUTURE) 5.0 SP3: containsAll
throw new UnsupportedOperationException("containsAll");
}
@Override
public boolean addAll(Collection extends T> c) {
addOrInsertOperation(c);
return true;
}
@Override
public boolean retainAll(Collection> c) {
// TODO (IN FUTURE) 5.0 SP3: retainAll
throw new UnsupportedOperationException("retainAll");
}
@Override
public final void copyTo(T[] array, int arrayIndex){
try{
CopyToInternal(this, array, arrayIndex, CountInternal(DistributedDataStructure.Set));
}catch (OperationFailedException |GeneralFailureException |AggregateException e) {
throw new OperationFailedRuntimeException();
}
}
@Override
public boolean removeAll(Collection> c) {
return remove(c);
}
private void addOrInsertOperation(Collection extends T> collection) {
if (collection == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: collection");
for (T item : collection) {
if (item == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: item");
validateTypeNotCacheItem(item.getClass());
}
verifyItemsType(collection);
getCache().hashSetAdd(getKey(), _lockHandle.getLockId(), _lockTimeout, collection, getWriteThruOptions());
}
private void verifyItemsType(Collection extends T> collection) {
for (T item : collection) {
CollectionUtil.validateTypeForHashSet(item.getClass());
}
}
private int removeOperation(Collection items) {
if (items == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: items");
}
for (T item : items) {
if (item == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: item");
}
validateTypeNotCacheItem(item.getClass());
}
return getCache().hashSetRemove(getKey(), _lockHandle.getLockId(), _lockTimeout, items, getWriteThruOptions());
}
private T removeRandomOperation(){
return getCache().hashSetRemoveRandom(getKey(), _lockHandle.getLockId(), _lockTimeout, getWriteThruOptions(),_valueType);
}
private boolean containsOperation(T value) throws ConnectionException, GeneralFailureException, OperationFailedException, AggregateException {
if (value == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: value");
validateTypeNotCacheItem(value.getClass());
return getCache().hashSetContains(getKey(), _lockHandle.getLockId(), _lockTimeout, value);
}
private T[] getRandomOperation(int count) {
if (count <= 0) {
throw new OperationFailedRuntimeException(ErrorCodes.DataTypes.COUNT_NON_POSITIVE, ErrorMessages.getErrorMessage(ErrorCodes.DataTypes.COUNT_NON_POSITIVE));
}
return getCache().hashSetGetRandom(getKey(), _lockHandle.getLockId(), _lockTimeout, count,_valueType);
}
private DistributedHashSet GetOtherSet(String other,Class> cls) throws CacheException {
CollectionCreateOrGetOperation tempVar = new CollectionCreateOrGetOperation(other, DistributedDataStructure.Set);
tempVar.setReadThruOptions(new ReadThruOptions(ReadMode.None));
if (getCache().getDataType(tempVar)) {
return new DistributedHashSetImpl(other, getCache(), null,cls);
}
return null;
}
private DistributedHashSet GetOrCreateOtherSet(String other,Class> cls) throws CacheException {
if (GetOtherSet(other,cls) == null) {
CollectionCreateOrGetOperation tempVar = new CollectionCreateOrGetOperation(other, DistributedDataStructure.Set);
tempVar.setReadThruOptions(new ReadThruOptions(ReadMode.None));
CollectionCreateOrGetOperation operation = tempVar;
getCache().createDataType(operation, null);
}
return new DistributedHashSetImpl(other, getCache(), null,cls);
}
@Override
public void addChangeListener(DataStructureDataChangeListener dataTypeDataNotificationCallback, EnumSet eventType, DataTypeEventDataFilter datafilter) throws CacheException {
if (eventType.contains(EventType.ItemUpdated)) {
throw new IllegalArgumentException("Registration for update event is not supported for this data type.");
} else {
super.addChangeListener(dataTypeDataNotificationCallback, eventType, datafilter);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy