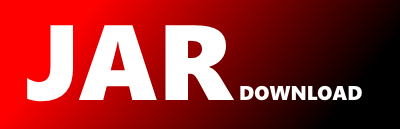
com.alachisoft.ncache.client.internal.datastructure.DistributedListImpl Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.datastructure;
import Alachisoft.NCache.Caching.Util.CollectionUtil;
import Alachisoft.NCache.Common.DataTypes.List.*;
import com.alachisoft.ncache.client.datastructures.DistributedList;
import com.alachisoft.ncache.runtime.caching.WriteThruOptions;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import com.alachisoft.ncache.runtime.caching.DistributedDataStructure;
import com.alachisoft.ncache.runtime.events.DataStructureDataChangeListener;
import com.alachisoft.ncache.runtime.events.DataTypeEventDataFilter;
import com.alachisoft.ncache.runtime.events.EventType;
import com.alachisoft.ncache.runtime.exceptions.*;
import com.alachisoft.ncache.runtime.exceptions.runtime.OperationFailedRuntimeException;
import java.util.*;
class DistributedListImpl extends DistributedDataStructureImpl implements DistributedList {
public DistributedListImpl(String key, CacheImpl cache, WriteThruOptions options,Class> cls) {
super(key, cache, options,cls);
}
@Override
protected DistributedDataStructure getDataType() {
return DistributedDataStructure.List;
}
@Override
public void trim(int start, int end) {
validateIndex(start);
validateIndex(end);
getCache().listRemoveWithIndex(getKey(), _lockHandle.getLockId(), _lockTimeout, start, end, ListRemoveWithIndexOperation.OpType.Trim, getWriteThruOptions());
}
@Override
public List getRange(int start, int count) {
validateIndex(start);
validateIndex(count);
return getCache().listGetBulk(getKey(), _lockHandle.getLockId(), _lockTimeout, start, count,_valueType);
}
@Override
public void addRange(Collection extends T> collection){
if (collection == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: collection");
}
CollectionUtil.verifyCollection(collection);
getCache().listAddWithoutIndex(getKey(), _lockHandle.getLockId(), _lockTimeout, collection, ListAddWithoutIndexOperation.OpType.AddRange, getWriteThruOptions());
}
@Override
public void removeRange(int index, int count) {
validateIndex(index);
if (count < 0) {
throw new IllegalArgumentException("Specified argument was out of the range of valid values");
}
getCache().listRemoveWithIndex(getKey(), _lockHandle.getLockId(), _lockTimeout, index, count, ListRemoveWithIndexOperation.OpType.RemoveRange, getWriteThruOptions());
}
@Override
public int removeRange(Collection> collection) {
if (collection == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: collection");
return getCache().listRemoveWithoutIndex(getKey(), _lockHandle.getLockId(), _lockTimeout, collection, getWriteThruOptions());
}
@Override
public boolean insertAfter(T pivot, T value) {
if (pivot == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: pivot");
if (value == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: value");
validateTypeNotCacheItem(value.getClass());
return getCache().listAddWithPivot(getKey(), _lockHandle.getLockId(), _lockTimeout, pivot, value, ListAddWithPivotOperation.OpType.InsertAfter, getWriteThruOptions());
}
@Override
public boolean insertBefore(T pivot, T value) {
if (pivot == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: pivot");
if (value == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: value");
validateTypeNotCacheItem(value.getClass());
return getCache().listAddWithPivot(getKey(), _lockHandle.getLockId(), _lockTimeout, pivot, value, ListAddWithPivotOperation.OpType.InsertBefore, getWriteThruOptions());
}
@Override
public T first() {
return getCache().listGetItem(getKey(), _lockHandle.getLockId(), _lockTimeout, -1, ListGetItemOperation.OpType.First,_valueType);
}
@Override
public T last() {
return getCache().listGetItem(getKey(), _lockHandle.getLockId(), _lockTimeout, -1, ListGetItemOperation.OpType.Last,_valueType);
}
@Override
public void insertAtHead(T value) {
if (value == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: value");
validateTypeNotCacheItem(value.getClass());
ArrayList arr = new ArrayList<>();
arr.add(value);
getCache().listAddWithoutIndex(getKey(), _lockHandle.getLockId(), _lockTimeout, arr, ListAddWithoutIndexOperation.OpType.InsertAtHead, getWriteThruOptions());
}
@Override
public void insertAtTail(T value) {
if (value == null) {
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: value");
}
validateTypeNotCacheItem(value.getClass());
ArrayList arrayList = new ArrayList<>();
arrayList.add(value);
getCache().listAddWithoutIndex(getKey(), _lockHandle.getLockId(), _lockTimeout, arrayList, ListAddWithoutIndexOperation.OpType.InsertAtTail, getWriteThruOptions());
}
@Override
public boolean contains(Object o) {
return indexOf(o) >= 0;
}
@Override
public Iterator iterator() {
return new DistributedCollectionIteratorImpl<>(getCache(), getKey(), DistributedDataStructure.List,_valueType);
}
@Override
public Object[] toArray() {
Object[] objArr = new Object[size()];
Iterator iterator = iterator();
int index = 0;
while (iterator.hasNext()) {
objArr[index++] = iterator.next();
}
return objArr;
}
/**
* TAKEN FROM LIST
* Returns an array containing all of the elements in this list in
* proper sequence (from first to last element); the runtime type of
* the returned array is that of the specified array. If the list fits
* in the specified array, it is returned therein. Otherwise, a new
* array is allocated with the runtime type of the specified array and
* the size of this list.
*
* If the list fits in the specified array with room to spare (i.e.,
* the array has more elements than the list), the element in the array
* immediately following the end of the list is set to {@code null}.
* (This is useful in determining the length of the list only if
* the caller knows that the list does not contain any null elements.)
*
*
Like the {@link #toArray()} method, this method acts as bridge between
* array-based and collection-based APIs. Further, this method allows
* precise control over the runtime type of the output array, and may,
* under certain circumstances, be used to save allocation costs.
*
*
Suppose {@code x} is a list known to contain only strings.
* The following code can be used to dump the list into a newly
* allocated array of {@code String}:
*
*
{@code
* String[] y = x.toArray(new String[0]);
* }
*
* Note that {@code toArray(new Object[0])} is identical in function to
* {@code toArray()}.
*
* @param a the array into which the elements of this list are to
* be stored, if it is big enough; otherwise, a new array of the
* same runtime type is allocated for this purpose.
* @return an array containing the elements of this list
* @throws ArrayStoreException if the runtime type of the specified array
* is not a supertype of the runtime type of every element in
* this list
* @throws NullPointerException if the specified array is null
*/
@Override
public T1[] toArray(T1[] a) {
int size = size();
Object[] elementData = toArray();
if (a.length < size)
// Make a new array of a's runtime type, but my contents:
return (T1[]) Arrays.copyOf(elementData, size, a.getClass());
System.arraycopy(elementData, 0, a, 0, size);
if (a.length > size)
a[size] = null;
return a;
}
@Override
public boolean add(T item) {
if (item == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: item");
validateTypeNotCacheItem(item.getClass());
ArrayList list = new ArrayList();
list.add(item);
addRange(list);
return true;
}
@Override
public boolean remove(Object item) {
if (item == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: item");
validateTypeNotCacheItem(item.getClass());
java.util.ArrayList