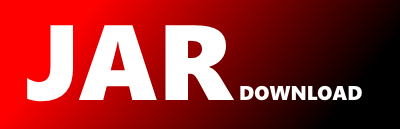
com.alachisoft.ncache.client.internal.datastructure.DistributedMapImpl Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.datastructure;
import Alachisoft.NCache.Common.DataTypes.Dictionary.DictionaryAddOperation;
import Alachisoft.NCache.Common.DataTypes.Dictionary.DictionaryContainsOperation;
import Alachisoft.NCache.Common.DataTypes.KeyValuePairedEnumerationFilter;
import com.alachisoft.ncache.client.datastructures.DistributedMap;
import com.alachisoft.ncache.runtime.caching.WriteThruOptions;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import com.alachisoft.ncache.runtime.caching.DistributedDataStructure;
import com.alachisoft.ncache.runtime.exceptions.*;
import com.alachisoft.ncache.runtime.exceptions.runtime.CacheRuntimeException;
import com.alachisoft.ncache.runtime.exceptions.runtime.GeneralFailureRuntimeException;
import com.alachisoft.ncache.runtime.exceptions.runtime.OperationFailedRuntimeException;
import java.util.*;
class DistributedMapImpl extends DistributedDataStructureImpl implements DistributedMap {
public DistributedMapImpl(String key, CacheImpl cache, WriteThruOptions options,Class> cls) {
super(key, cache, options,cls);
}
@Override
protected DistributedDataStructure getDataType() {
return DistributedDataStructure.Map;
}
@Override
public void insert(Map entries) {
addOrInsertOperation(entries, DictionaryAddOperation.OpType.Insert);
}
@Override
public int remove(Collection keys) {
try {
return removeOperation(keys);
}catch (OperationFailedException | AggregateException e) {
throw new OperationFailedRuntimeException(e);
} catch (GeneralFailureException e) {
throw new GeneralFailureRuntimeException(e);
}
}
@Override
public Collection get(Collection keys) {
try {
return getOperation(keys);
} catch (CacheException e) {
throw new CacheRuntimeException(e);
}
}
@Override
public boolean isEmpty() {
return size() < 1;
}
@Override
public boolean containsKey(Object key) {
try {
return containsOperation(key, DictionaryContainsOperation.OpType.ContainsKey);
} catch (OperationFailedException | AggregateException e) {
throw new OperationFailedRuntimeException(e);
} catch (GeneralFailureException e) {
throw new GeneralFailureRuntimeException(e);
}
}
@Override
public boolean containsValue(Object value) {
// TODO (IN FUTURE) 5.0 SP3: containsValue implementation does not exist
throw new UnsupportedOperationException("containsValue");
}
@Override
public V get(Object key) {
try {
return getItem(key);
} catch (CacheException e) {
throw new CacheRuntimeException(e);
}
}
@Override
public V put(String key, V value) {
// TODO (IN FUTURE) 5.0 SP3: return previous version if present
setItem(key, value);
return null;
}
@Override
public V remove(Object key) {
// TODO (IN FUTURE) 5.0 SP3: remove has synchronization issues
if (key.getClass() != String.class)
throw new ClassCastException("key not String");
if(key==null || ((String) key).isEmpty())
throw new IllegalArgumentException("Value cannot be null"+System.lineSeparator()+"Parameter name: key");
V previousValue = get(key);
ArrayList arr = new ArrayList();
arr.add((String) key);
try {
removeOperation(arr);
} catch (OperationFailedException | AggregateException e) {
throw new OperationFailedRuntimeException(e);
} catch (GeneralFailureException e) {
throw new GeneralFailureRuntimeException(e);
}
return previousValue;
}
@Override
public void putAll(Map extends String, ? extends V> m) {
addOrInsertOperation(m, DictionaryAddOperation.OpType.Insert);
}
@Override
public Set keySet() {
Set keys = new HashSet();
DistributedPairedCollectionIterator enumerator = new DistributedPairedCollectionIterator(getCache(), getKey(), DistributedDataStructure.Map, KeyValuePairedEnumerationFilter.KeysOnly,_valueType);
while (enumerator.hasNext())
{
keys.add(enumerator.next().getKey());
}
return keys;
}
@Override
public Collection values() {
ArrayList values = new ArrayList();
DistributedPairedCollectionIterator enumerator = new DistributedPairedCollectionIterator(getCache(), getKey(), DistributedDataStructure.Map, KeyValuePairedEnumerationFilter.ValuesOnly,_valueType);
while (enumerator.hasNext())
{
values.add(enumerator.next().getValue());
}
return values;
}
@Override
public Set> entrySet() {
Set> entries = new HashSet<>();
DistributedPairedCollectionIterator enumerator = new DistributedPairedCollectionIterator(getCache(), getKey(), DistributedDataStructure.Map, KeyValuePairedEnumerationFilter.BothKeysAndValues,_valueType);
while (enumerator.hasNext())
{
entries.add(enumerator.next());
}
return entries;
}
private V getItem(Object key) throws CacheException {
if (key.getClass() != String.class)
throw new ClassCastException("key not String");
if (((String) key).isEmpty())
throw new IllegalArgumentException("Value cannot be null"+System.lineSeparator()+"Parameter name: key");
ArrayList arr = new ArrayList();
arr.add((String) key);
List value = getCache().dictionaryGet(getKey(), _lockHandle.getLockId(), _lockTimeout, arr,_valueType);
if (value.size() == 0) {
return null;
}
return value.get(0);
}
private void setItem(String key, V value) {
if (key == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: key");
if (key.isEmpty())
throw new IllegalArgumentException("Value cannot be empty."+System.lineSeparator()+"Parameter name: key");
if (value == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: value");
java.util.HashMap dictionary = new java.util.HashMap();
dictionary.put(key, value);
addOrInsertOperation(dictionary, DictionaryAddOperation.OpType.Insert);
}
private void addOrInsertOperation(Map extends String, ? extends V> collection, DictionaryAddOperation.OpType operationType) {
if (collection == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: collection");
for (Entry extends String, ? extends V> item : collection.entrySet()) {
if (item.getKey() == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: key");
if (item.getKey().isEmpty())
throw new IllegalArgumentException("Value cannot be empty."+System.lineSeparator()+"Parameter name: key");
if (item.getValue() == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: value");
validateTypeNotCacheItem(item.getValue().getClass());
}
getCache().dictionaryAdd(getKey(), collection, _lockHandle.getLockId(), _lockTimeout, getWriteThruOptions(), operationType);
}
private Collection getOperation(Collection keys) throws CacheException {
if (keys == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: keys");
for (String key : keys) {
if (key == null || key.isEmpty())
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: key");
}
return getCache().dictionaryGet(getKey(), _lockHandle.getLockId(), _lockTimeout, keys,_valueType);
}
private int removeOperation(Collection keys) throws OperationFailedException, GeneralFailureException, AggregateException {
if (keys == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: keys");
for (String key : keys)
if (key.isEmpty() || key == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: key");
return getCache().dictionaryRemove(getKey(), keys, _lockHandle.getLockId(), _lockTimeout, getWriteThruOptions());
}
private boolean containsOperation(Object key, DictionaryContainsOperation.OpType operationType) throws OperationFailedException, GeneralFailureException, ConnectionException, AggregateException {
return containsOperation(key, null, operationType);
}
private boolean containsOperation(Object key, V value, DictionaryContainsOperation.OpType operationType) throws OperationFailedException, GeneralFailureException, ConnectionException, AggregateException {
if(key == null )
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: key");
if (key.getClass() != String.class)
throw new ClassCastException("Key is not String");
if (((String) key).isEmpty()) {
throw new IllegalArgumentException("Value cannot be empty."+System.lineSeparator()+"Parameter name: key");
}
if (value != null) {
validateTypeNotCacheItem(value.getClass());
}
return getCache().dictionaryContains((String) getKey(), _lockHandle.getLockId(), _lockTimeout, (String) key, value, operationType);
}
@Override
public final void copyTo(java.util.Map.Entry[] array, int arrayIndex) {
try {
CopyToInternal(this.entrySet(), array, arrayIndex, CountInternal(DistributedDataStructure.Map));
} catch (OperationFailedException |GeneralFailureException |AggregateException e) {
throw new OperationFailedRuntimeException();
}
}
@Override
public Iterator> iterator()
{
return new DistributedPairedCollectionIterator(getCache(), getKey(), DistributedDataStructure.Map, KeyValuePairedEnumerationFilter.BothKeysAndValues,_valueType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy