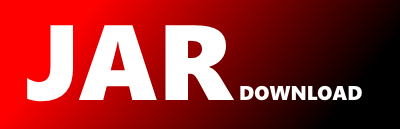
com.alachisoft.ncache.client.internal.datastructure.DistributedPairedCollectionIterator Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.datastructure;
import Alachisoft.NCache.Common.BitSet;
import Alachisoft.NCache.Common.BitSetConstants;
import Alachisoft.NCache.Common.DataTypes.KeyValuePairedEnumerationFilter;
import Alachisoft.NCache.Common.DataTypes.Responses.GetPairedCollectionChunkResponse;
import Alachisoft.NCache.Common.Enum.UserObjectType;
import Alachisoft.NCache.Common.JSON.ExtendedJsonValueBase;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import com.alachisoft.ncache.runtime.caching.DistributedDataStructure;
import com.alachisoft.ncache.runtime.exceptions.*;
import com.alachisoft.ncache.runtime.exceptions.runtime.CacheRuntimeException;
import com.alachisoft.ncache.runtime.exceptions.runtime.OperationFailedRuntimeException;
import java.util.HashMap;
import java.util.Map;
class DistributedPairedCollectionIterator extends DistributedCollectionIteratorImpl> {
private KeyValuePairedEnumerationFilter _filter;
private Class _valueType;
DistributedPairedCollectionIterator(CacheImpl cache, String key, DistributedDataStructure collectionType, KeyValuePairedEnumerationFilter filter,Class> cls) {
super(cache, key, collectionType,cls);
_filter = filter;
}
@Override
protected boolean moveNextInternal(boolean peekOnly) throws OperationFailedException, AggregateException, GeneralFailureException {
if (_index >= _size && !getNextAvailableChunk()) {
return MoveNextRare();
}
GetPairedCollectionChunkResponse response = (GetPairedCollectionChunkResponse) _response;
Map.Entry pair = response.getDataChunk().get(_index);
if (peekOnly) {
return true;
}
try {
_current = GetKeyValueFromProtoPair(pair, _filter);
_index++;
} catch (OperationFailedException e) {
throw new OperationFailedRuntimeException(e);
} catch (CacheException e) {
throw new CacheRuntimeException(e);
}
return true;
}
@Override
protected boolean getNextAvailableChunk() throws OperationFailedException, GeneralFailureException, AggregateException {
if (_response == null || !_response.getIsLastChunk()) {
_response = _cache.getPairedCollectionChunkResponse(_key, _collectionType, _response != null ? _response.getVersion() : 0, _response != null ? _response.getNextIndex() : 0, _filter);
GetPairedCollectionChunkResponse response = (GetPairedCollectionChunkResponse) _response;
if (response.getDataChunk().size() == 0) {
return false;
}
_size = response.getDataChunk().size();
_index = 0;
return true;
} else {
return false;
}
}
private Map.Entry GetKeyValueFromProtoPair(java.util.Map.Entry pair, KeyValuePairedEnumerationFilter filter) throws CacheException {
switch (filter) {
case KeysOnly:
return new HashMap.SimpleEntry((K) ((Object) pair.getKey()), null);
case ValuesOnly:
return new HashMap.SimpleEntry(null, _cache.safeDeserialize(pair.getValue(), _cache.getSerializationContext(), new BitSet((byte) BitSetConstants.JsonData), UserObjectType.DistributedDataType, null));
case BothKeysAndValues:
return new HashMap.SimpleEntry((K) ((Object) pair.getKey()), _cache.safeDeserialize(pair.getValue(), _cache.getSerializationContext(), new BitSet((byte) BitSetConstants.JsonData), UserObjectType.DistributedDataType, null));
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy