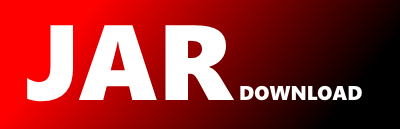
com.alachisoft.ncache.client.internal.datastructure.DistributedQueueImpl Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.datastructure;
import Alachisoft.NCache.Common.DataTypes.Queue.QueueDequeueOperation;
import Alachisoft.NCache.Common.DataTypes.Queue.QueueEnqueueOperation;
import com.alachisoft.ncache.client.datastructures.DistributedQueue;
import com.alachisoft.ncache.runtime.caching.WriteThruOptions;
import com.alachisoft.ncache.client.internal.caching.CacheImpl;
import com.alachisoft.ncache.runtime.caching.DistributedDataStructure;
import com.alachisoft.ncache.runtime.events.DataStructureDataChangeListener;
import com.alachisoft.ncache.runtime.events.DataTypeEventDataFilter;
import com.alachisoft.ncache.runtime.events.EventType;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import java.util.*;
class DistributedQueueImpl extends DistributedDataStructureImpl implements DistributedQueue {
public DistributedQueueImpl(String key, CacheImpl cache, WriteThruOptions options,Class cls) {
super(key, cache, options,cls);
}
@Override
protected DistributedDataStructure getDataType() {
return DistributedDataStructure.Queue;
}
@Override
public void copyTo(T[] array, int arrayIndex) {
CopyToInternal(this, array, arrayIndex, size());
}
@Override
public boolean contains(Object item) {
if (item == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: item");
validateTypeNotCacheItem(item.getClass());
return getCache().queueSearch(getKey(), _lockHandle.getLockId(), _lockTimeout, item);
}
@Override
public Iterator iterator() {
return new DistributedCollectionIteratorImpl<>(getCache(), getKey(), DistributedDataStructure.Queue,_valueType);
}
@Override
public Object[] toArray() {
// int size = size();
Object[] objArr = new Object[size()];
Iterator iterator = iterator();
// while(iterator.hasNext())
// {
// System.out.println(iterator.next().toString());
// }
// iterator = iterator();
int index = 0;
while (iterator.hasNext()) {
objArr[index++] = iterator.next();
}
return objArr;
}
@Override
public T1[] toArray(T1[] a) {
// implementation taken from ArrayList
Object[] elementData = toArray();
int size = elementData.length;
if (a.length < size)
// Make a new array of a's runtime type, but my contents:
return (T1[]) Arrays.copyOf(elementData, size, a.getClass());
System.arraycopy(elementData, 0, a, 0, size);
if (a.length > size)
a[size] = null;
return a;
}
@Override
public boolean add(T item) {
if (item == null)
throw new IllegalArgumentException("Value cannot be null."+System.lineSeparator()+"Parameter name: item");
validateTypeNotCacheItem(item.getClass());
return getCache().queueEnqueue(getKey(), _lockHandle.getLockId(), _lockTimeout, item, QueueEnqueueOperation.OpType.Enqueue, getWriteThruOptions());
}
@Override
public boolean remove(Object o) {
// TODO (IN FUTURE) 5.0 SP3: remove
throw new UnsupportedOperationException("remove");
}
@Override
public boolean containsAll(Collection> c) {
// TODO (IN FUTURE) 5.0 SP3: containsAll
throw new UnsupportedOperationException("containsAll");
}
@Override
public boolean addAll(Collection extends T> c) {
// TODO (IN FUTURE) 5.0 SP3: addAll
throw new UnsupportedOperationException("addAll");
}
@Override
public boolean removeAll(Collection> c) {
// TODO (IN FUTURE) 5.0 SP3: removeAll
throw new UnsupportedOperationException("removeAll");
}
@Override
public boolean retainAll(Collection> c) {
// TODO (IN FUTURE) 5.0 SP3: retainAll
throw new UnsupportedOperationException("retainAll");
}
@Override
public boolean offer(T item) {
return add(item);
}
@Override
public T remove() {
if (isEmpty())
throw new NoSuchElementException("element");
return poll();
}
@Override
public T poll() {
if (isEmpty())
return null;
return getCache().queueDequeue(getKey(), _lockHandle.getLockId(), _lockTimeout, QueueDequeueOperation.OpType.Dequeue, getWriteThruOptions(),_valueType);
}
@Override
public T element() {
if (isEmpty())
throw new NoSuchElementException("element");
return peek();
}
@Override
public T peek() {
if (isEmpty())
return null;
return getCache().queuePeek(getKey(), _lockHandle.getLockId(), _lockTimeout,_valueType);
}
@Override
public void addChangeListener(DataStructureDataChangeListener dataTypeDataNotificationCallback, EnumSet eventType, DataTypeEventDataFilter datafilter) throws CacheException {
if (eventType.contains(EventType.ItemUpdated)) {
throw new IllegalArgumentException("Registration for update event is not supported for this data type.");
} else {
super.addChangeListener(dataTypeDataNotificationCallback, eventType, datafilter);
}
}
private void validateClass(Object o) {
if (o.getClass() != this.getClass())
throw new ClassCastException("Object class is not the same as this class");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy