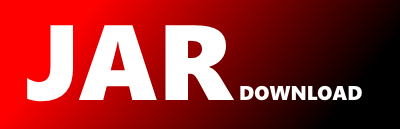
com.alachisoft.ncache.client.internal.util.ClientConfiguration Maven / Gradle / Ivy
package com.alachisoft.ncache.client.internal.util;
import Alachisoft.NCache.Common.ServicePropValues;
import Alachisoft.NCache.Config.Mapping;
import com.alachisoft.ncache.client.CacheConnectionOptions;
import com.alachisoft.ncache.client.CacheManager;
import com.alachisoft.ncache.client.LogLevel;
import com.alachisoft.ncache.client.ClientCacheSyncMode;
import com.alachisoft.ncache.client.ServerInfo;
import com.alachisoft.ncache.runtime.exceptions.ConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import java.io.File;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Hashtable;
import java.util.Iterator;
import static com.alachisoft.ncache.client.LogLevel.*;
import static tangible.DotNetToJavaStringHelper.isNullOrEmpty;
public final class ClientConfiguration {
//region Fields
private boolean _balanceNodes = true;
private boolean _loadServersFromConfigFile = true;
private boolean _loadCacheConfiguration = true;
private int _serverPort = 9800;
private int _clientRequestTimeout = 90 * 1000;
private int _connectionTimeout = 5000;
private int _retries = 5;
private int _retryInternal = 1000;
private int _itemSizeThreshHold = 0;
private double _retryConnectionDelay = 10; // 10 min
private String _cacheId;
private String _clientCacheID = "";
private String _defaultReadThruProvider;
private String _defaultWriteThruProvider;
private ArrayList _servers = new ArrayList();
private Hashtable _compactTypes = new Hashtable();
private ClientCacheSyncMode _clientCacheSyncMode = ClientCacheSyncMode.Optimistic;
private CacheConnectionOptions _cacheConnectionOptions;
private Hashtable _mappedServer = new Hashtable();
private Search _search = Search.LocalSearch;
private Search _result = Search.LocalSearch;
private String _localServerIP;
private boolean enableClientLogs;
private boolean enableDetailedClientLogs;
private boolean iPMappingConfigured;
private int currentServerIndex;
private boolean importHashmap = true;
private String clientCacheID;
private int configServerPort = 9800;
private int commandRetries =1;
private float commandRetryInterval;
private boolean _enableKeepAlive = false;
private int keepAliveInterval= 30;
private LogLevel logLevels;
private static String _installDir = null;
String _configFile = "client.ncconf";
private boolean _skipUnAvailableClientCache= true;
private int _retryL1ConnectionInterval= 10;
//endregion
// region Properties
public boolean isSkipUnAvailableClientCache() {
return _skipUnAvailableClientCache;
}
public void setSkipUnAvailableClientCache(boolean skipUnAvailableClientCache) {
_skipUnAvailableClientCache = skipUnAvailableClientCache;
}
public int getRetryL1ConnectionInterval() {
return _retryL1ConnectionInterval;
}
public void setRetryL1ConnectionInterval(int retryL1ConnectionInterval) {
_retryL1ConnectionInterval = _retryL1ConnectionInterval;
}
public ClientConfiguration(String cacheId) {
_cacheId = cacheId;
_cacheConnectionOptions = null;
}
public ClientConfiguration(String cacheId, CacheConnectionOptions cacheConnectionOptions) {
_cacheId = cacheId;
_cacheConnectionOptions = (CacheConnectionOptions) cacheConnectionOptions.clone();
if (_cacheConnectionOptions != null) {
if (_cacheConnectionOptions.getServerList() != null && _cacheConnectionOptions.getServerList().size() > 0) {
for (ServerInfo serverInfo : _cacheConnectionOptions.getServerList()) {
serverInfo.setIsUserProvidedInternal(true);
addServer(serverInfo);
}
_loadServersFromConfigFile = false;
}
}
}
public CacheConnectionOptions getCacheConnectionOptions() {
return _cacheConnectionOptions;
}
public void setLoadCacheConfiguration(boolean load) {
_loadCacheConfiguration = load;
}
public String getLocalServerIP() {
return this._localServerIP;
}
public void setLocalServerIP(String ip) {
this._localServerIP = ip;
}
public boolean getEnableClientLogs() {
return enableClientLogs;
}
public void setEnableClientLogs(boolean value) {
enableClientLogs = value;
}
public boolean getEnableDetailedClientLogs() {
return enableDetailedClientLogs;
}
public void setEnableDetailedClientLogs(boolean value) {
enableDetailedClientLogs = value;
}
public boolean getIPMappingConfigured() {
return iPMappingConfigured;
}
public void setIPMappingConfigured(boolean value) {
iPMappingConfigured = value;
}
public int getServerCount() {
return _servers.size();
}
public int getCurrentServerIndex() {
return currentServerIndex;
}
public void setCurrentServerIndex(int value) {
currentServerIndex = value;
}
public int getItemSizeThreshHold() {
return _itemSizeThreshHold;
}
public boolean getBalanceNodes() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.LOADBALANCE)) {
return _cacheConnectionOptions.getLoadBalance();
}
return _balanceNodes;
}
public void setBalanceNodes(boolean value) {
_balanceNodes = value;
}
public boolean getImportHashmap() {
return importHashmap;
}
public void setImportHashmap(boolean value) {
importHashmap = value;
}
public boolean getClientCache() {
return getClientCacheID().trim().length() > 0;
}
public String getClientCacheID() {
return clientCacheID;
}
public void setClientCacheID(String value) {
clientCacheID = value;
}
public ClientCacheSyncMode getCacheSyncMode() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.CACHESYNCMODE)) {
return _cacheConnectionOptions.getClientCacheMode();
}
return _clientCacheSyncMode;
}
public void setCacheSyncMode(ClientCacheSyncMode value) {
_clientCacheSyncMode = value;
}
public String getDefaultReadThru() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.DEFAULTREADTHRUPROVIDER)) {
return _cacheConnectionOptions.getDefaultReadThruProvider();
}
return _defaultReadThruProvider;
}
public String getDefaultWriteThru() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.DEFAULTWRITETHRUPROVIDER)) {
return _cacheConnectionOptions.getDefaultWriteThruProvider();
}
return _defaultWriteThruProvider;
}
public String getBindIP() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.BINDIP)) {
return _cacheConnectionOptions.getClientBindIP();
}
File file;
String bindIP = "";
try {
String path = DirectoryUtil.getBaseFilePath("client.ncconf");
file = new File(path);
} catch (Exception e) {
return bindIP;
}
Document configuration = null;
DocumentBuilderFactory builderFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = null;
try {
builder = builderFactory.newDocumentBuilder();
configuration = builder.parse(file);
NodeList clientNodesServer = configuration.getElementsByTagName("ncache-server");
NodeList clientNodesClient = configuration.getElementsByTagName("ncache-client");
NodeList clientNodes = (clientNodesClient != null && clientNodesClient.getLength() > 0) ? clientNodesClient : clientNodesServer;
if (clientNodes != null && clientNodes.getLength() > 0) {
Node childNode = clientNodes.item(0);
if (childNode != null) {
NamedNodeMap attributes = childNode.getAttributes();
if (attributes != null) {
String currentAttrib = "";
if (attributes.getNamedItem("local-server-ip") != null) {
currentAttrib = attributes.getNamedItem("local-server-ip").getNodeValue();
if (currentAttrib != null) {
bindIP = currentAttrib;
}
}
}
}
}
return bindIP;
} catch (Exception ex) {
return bindIP;
}
}
public java.util.ArrayList getServerList() {
synchronized (_servers) {
Object tempVar = _servers.clone();
return (java.util.ArrayList) ((tempVar instanceof java.util.ArrayList) ? tempVar : null);
}
}
public ServerInfo getServerAt(int index) {
ServerInfo nextServer = null;
synchronized (_servers) {
if (_servers != null && _servers.size() > 0 && index < _servers.size()) {
nextServer = (ServerInfo) ((_servers.get(index) instanceof ServerInfo) ? _servers.get(index) : null);
}
}
return nextServer;
}
public ServerInfo getNextServer() {
ServerInfo nextServer = null;
synchronized (_servers) {
if (_servers != null && _servers.size() > 0) {
if (getCurrentServerIndex() > _servers.size()) {
setCurrentServerIndex(0);
}
if (getCurrentServerIndex() < 0) {
setCurrentServerIndex(0);
}
nextServer = (ServerInfo) ((_servers.get(getCurrentServerIndex()) instanceof ServerInfo) ? _servers.get(getCurrentServerIndex()) : null);
setCurrentServerIndex(getCurrentServerIndex() + 1);
if (getCurrentServerIndex() > _servers.size() - 1) {
setCurrentServerIndex(0);
}
}
}
return nextServer;
}
public int getConfigServerPort() {
return configServerPort;
}
private void setConfigServerPort(int value) {
configServerPort = value;
}
public int getClientRequestTimeout() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.CLIENTREQUESTOPTIME)) {
return (int) _cacheConnectionOptions.getClientRequestTimeOut().getTotalMiliSeconds();
}
return _clientRequestTimeout;
}
public int getConnectionTimeout() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.CONNECTIONTIMEOUT)) {
return (int) _cacheConnectionOptions.getConnectionTimeout().getTotalMiliSeconds();
}
return _connectionTimeout;
}
public double getRetryConnectionDelay() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.RETRYCONNECTIONDELAY)) {
return _cacheConnectionOptions.getRetryConnectionDelay().getTotalMinutes();
}
return _retryConnectionDelay;
}
public int getConnectionRetries() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.CONNECTIONRETRIES)) {
return _cacheConnectionOptions.getConnectionRetries();
}
return _retries;
}
public int getRetryInterval() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.RETRYINTERVAL)) {
return (int) _cacheConnectionOptions.getRetryInterval().getTotalMiliSeconds();
}
return _retryInternal;
}
public int getCommandRetries() {
return commandRetries;
}
private void setCommandRetries(int value) {
commandRetries = value;
}
public float getCommandRetryInterval() {
return commandRetryInterval;
}
private void setCommandRetryInterval(float value) {
commandRetryInterval = value;
}
public boolean getEnableKeepAlive() {
return _enableKeepAlive;
}
public void setEnableKeepAlive(boolean value) {
_enableKeepAlive = value;
}
public int getKeepAliveInterval() {
return keepAliveInterval;
}
private void setKeepAliveInterval(int value) {
keepAliveInterval = value;
}
public LogLevel getLogLevels() {
return logLevels;
}
private void setLogLevels(LogLevel value) {
logLevels = value;
}
//endregion
// region Constructors
public int getServerPort() {
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.PORT)) {
return _cacheConnectionOptions.getPort();
}
return _serverPort;
}
public void setServerPort(int value) {
_serverPort = value;
}
// endregion
//region Methods
public void addServer(ServerInfo server) {
if (_servers != null && (!isNullOrEmpty(server.getName()) || server.getIP() != null)) {
synchronized (_servers) {
if (!_servers.contains(server)) {
_servers.add(server);
}
}
}
}
public void removeServer(ServerInfo server) {
if (_servers != null && server != null) {
synchronized (_servers) {
if (_servers.contains(server)) {
ServerInfo existingServer = (ServerInfo) _servers.get(_servers.indexOf(server));
if (!existingServer.getIsUserProvidedInternal()) {
if (getCurrentServerIndex() == (_servers.size() - 1)) {
int index = getCurrentServerIndex();
setCurrentServerIndex(index--);
}
_servers.remove(server);
}
}
}
}
}
public ServerInfo getMappedServer(String ip, int port) {
ServerInfo mapping = null;
if (_mappedServer != null || !_mappedServer.isEmpty()) {
for (Iterator it = _mappedServer.keySet().iterator(); it.hasNext(); ) {
ServerInfo rm = (ServerInfo) it.next();
if (rm.getName().equals(ip)) {
mapping = new ServerInfo();
mapping = (ServerInfo) _mappedServer.get(rm);
}
}
}
//Incase the map is null the method will return the original IP and Port
if (mapping == null) {
mapping = new ServerInfo(ip, port);
}
return mapping;
}
private void loadRemoteServerConfig(NodeList cacheConfig) {
try {
if (!_loadServersFromConfigFile) {
return;
}
int PriorityCounter = 1;
for (int i = 0; i < cacheConfig.getLength(); i++) {
Node currentConfig = cacheConfig.item(i);
if (currentConfig.getNodeName().equals("server")) {
ServerInfo remoteServer = new ServerInfo();
try {
remoteServer.setName(currentConfig.getAttributes().getNamedItem("name").getNodeValue());
remoteServer.setPriorityInternal((short) PriorityCounter);
PriorityCounter = PriorityCounter + 1;
if (currentConfig.getAttributes().getNamedItem("port-range") != null) {
remoteServer.setPortRangeInternal(Short.parseShort(currentConfig.getAttributes().getNamedItem("port-range").getNodeValue()));
}
} catch (Exception ignored) {
}
remoteServer.setPort(getServerPort());
if ((remoteServer.getName() != null || remoteServer.getIP() != null) && remoteServer.getPort() != -1) {
synchronized (_servers) {
if (!_servers.contains(remoteServer)) {
if (_mappedServer != null && _mappedServer.size() != 0) {
ServerInfo rm = getMappedServer(remoteServer.getName(), remoteServer.getPort());
remoteServer.setName(rm.getName());
remoteServer.setPort(rm.getPort());
if (!_servers.contains(remoteServer)) {
_servers.add(remoteServer);
}
} else {
remoteServer.setIsUserProvidedInternal(true);
_servers.add(remoteServer);
}
}
}
}
}
}
synchronized (_servers) {
Collections.sort(_servers);
}
} catch (Exception ignored) {
}
}
private void loadRemoteServerMappingConfig(NodeList cacheConfig) {
Hashtable updatedServerMap = new Hashtable();
try {
for (int i = 0; i < cacheConfig.getLength(); i++) {
Node currentConfig = cacheConfig.item(i);
if (currentConfig.getNodeName().equals("server-end-point")) {
NodeList _mappingConfig = currentConfig.getChildNodes();
for (int j = 0; j < _mappingConfig.getLength(); j++) {
Node mapNodeConfig = _mappingConfig.item(j);
if (mapNodeConfig.getNodeName().equals("end-point")) {
ServerInfo publicServer = new ServerInfo();
ServerInfo privateServer = new ServerInfo();
try {
privateServer.setName(mapNodeConfig.getAttributes().getNamedItem("private-ip").getNodeValue());
privateServer.setPort(Integer.parseInt(mapNodeConfig.getAttributes().getNamedItem("private-port").getNodeValue()));
publicServer.setName(mapNodeConfig.getAttributes().getNamedItem("public-ip").getNodeValue());
publicServer.setPort(Integer.parseInt(mapNodeConfig.getAttributes().getNamedItem("public-port").getNodeValue()));
} catch (Exception ignored) {
}
if (privateServer.getName() != null) {
synchronized (_mappedServer) {
if (_mappedServer.size() != 0) {
for (ServerInfo rm : (ArrayList) _mappedServer.keySet()) {
if (!rm.getName().equals(privateServer.getName())) {
updatedServerMap.put(privateServer, publicServer);
}
}
} else {
_mappedServer.put(privateServer, publicServer);
}
}
}
}
}
setIPMappingConfigured(true);
}
}
for (ServerInfo rms : (ArrayList) updatedServerMap.keySet()) {
_mappedServer.put(rms, updatedServerMap.get(rms));
}
} catch (Exception e2) {
}
}
public void addMappedServers(ArrayList mappedServerList) {
Hashtable updatedServerMap = new Hashtable();
if (mappedServerList != null) {
for (Mapping node : mappedServerList) {
ServerInfo publicServer = new ServerInfo();
ServerInfo privateServer = new ServerInfo();
privateServer.setName(node.getPrivateIP());
privateServer.setPort(node.getPrivatePort());
publicServer.setName(node.getPublicIP());
publicServer.setPort(node.getPublicPort());
if (privateServer.getName() != null) {
synchronized (_mappedServer) {
if (_mappedServer.size() != 0) {
boolean keyExists = false;
for (ServerInfo rm : (ArrayList) _mappedServer.keySet()) {
if (!rm.getName().equals(privateServer.getName())) {
keyExists = false;
} else {
keyExists = true;
ServerInfo originalPublicServer = (ServerInfo) _mappedServer.get(rm);
String existingServer = originalPublicServer.getName() + ":" + originalPublicServer.getPort();
String newServer = publicServer.getName() + ":" + publicServer.getPort();
if (!existingServer.equals(newServer)) {
updatedServerMap.put(privateServer, publicServer);
}
break;
}
}
if (keyExists == false) {
updatedServerMap.put(privateServer, publicServer);
}
} else {
_mappedServer.put(privateServer, publicServer);
}
}
}
}
for (ServerInfo rms : (ArrayList) updatedServerMap.keySet()) {
_mappedServer.put(rms, updatedServerMap.get(rms));
}
}
}
public boolean isDifferentParamPort(int usedParamPort) {
return usedParamPort != getConfigServerPort();
}
public void loadConfiguration() throws ConfigurationException {
String c_configFileName = null;
try {
if (_cacheId == null && _loadCacheConfiguration) {
return;
}
tangible.OutObject tempOut_Result = new tangible.OutObject();
c_configFileName = DirectoryUtil.getBaseFilePath("client.ncconf", _search, tempOut_Result);
_result = tempOut_Result.argValue;
String path = getConfigPath();
File f = new File(path);
if (!f.exists()) {
throw new Exception("'client.ncconf' not found or does not contain server information");
}
Document response = null;
DocumentBuilderFactory builderFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = null;
try {
builder = builderFactory.newDocumentBuilder();
} catch (ParserConfigurationException ignored) {
}
response = builder.parse(f);
boolean serverPortFound = false;
NodeList serverPortNL = response.getElementsByTagName("ncache-server");
if (serverPortNL != null && serverPortNL.getLength() > 0) {
Node ncacheServerDetails = serverPortNL.item(0);
if (ncacheServerDetails != null) {
NamedNodeMap attributes = ncacheServerDetails.getAttributes();
if (attributes != null) {
String currentAttributes = "";
if (attributes.getNamedItem("port") != null && attributes.getNamedItem("port").getNodeValue() != null) {
setConfigServerPort(Integer.parseInt(attributes.getNamedItem("port").getNodeValue()));
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getPort() != null) {
setServerPort(_cacheConnectionOptions.getPort());
} else {
setServerPort(getConfigServerPort());
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getClientRequestTimeOut() != null) {
_clientRequestTimeout = Math.toIntExact(_cacheConnectionOptions.getClientRequestTimeOut().getTotalMiliSeconds());
} else {
if (attributes.getNamedItem("client-request-timeout") != null && attributes.getNamedItem("client-request-timeout").getNodeValue() != null) {
_clientRequestTimeout = Integer.parseInt(attributes.getNamedItem("client-request-timeout").getNodeValue()) * 1000;
}
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getConnectionRetries() != null) {
_retries = _cacheConnectionOptions.getConnectionRetries();
} else {
if (attributes.getNamedItem("connection-retries") != null && attributes.getNamedItem("connection-retries").getNodeValue() != null) {
_retries = Integer.parseInt(attributes.getNamedItem("connection-retries").getNodeValue());
}
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getRetryInterval() != null) {
_retryInternal = Math.toIntExact(_cacheConnectionOptions.getRetryInterval().getTotalMiliSeconds());
} else {
if (attributes.getNamedItem("retry-interval") != null && attributes.getNamedItem("retry-interval").getNodeValue() != null) {
_retryInternal = Integer.parseInt(attributes.getNamedItem("retry-interval").getNodeValue()) * 1000;
}
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getCommandRetries() != null) {
setCommandRetries(_cacheConnectionOptions.getCommandRetries());
} else {
if (attributes.getNamedItem("command-retries") != null && attributes.getNamedItem("command-retries").getNodeValue() != null) {
setCommandRetries(Integer.parseInt(attributes.getNamedItem("command-retries").getNodeValue()));
}
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.COMMANDRETRYINTERVAL)) {
setCommandRetryInterval(new Double(_cacheConnectionOptions.getCommandRetryInterval().getTotalSeconds()).floatValue());
} else {
if (attributes.getNamedItem("command-retry-interval") != null && attributes.getNamedItem("command-retry-interval").getNodeValue() != null) {
setCommandRetryInterval(Float.parseFloat(attributes.getNamedItem("command-retry-interval").getNodeValue()));
}
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.ENABLEKEEPALIVE)) {
setEnableKeepAlive(_cacheConnectionOptions.getEnableKeepAlive());
} else {
if (attributes.getNamedItem("enable-keep-alive") != null && attributes.getNamedItem("enable-keep-alive").getNodeValue() != null) {
setEnableKeepAlive(Boolean.parseBoolean(attributes.getNamedItem("enable-keep-alive").getNodeValue()));
}
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.KEEPALIVEINTERVAL)) {
setKeepAliveInterval((int) _cacheConnectionOptions.getKeepAliveInterval().getTotalSeconds());
} else {
if (attributes.getNamedItem("keep-alive-interval") != null && attributes.getNamedItem("keep-alive-interval").getNodeValue() != null) {
setKeepAliveInterval(Integer.parseInt(attributes.getNamedItem("keep-alive-interval").getNodeValue()));
if (getKeepAliveInterval() < 1 || getKeepAliveInterval() > 2 * 60 * 60) {
setKeepAliveInterval(30);
}
}
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getConnectionTimeout() != null) {
_connectionTimeout = (int) _cacheConnectionOptions.getConnectionTimeout().getTotalMiliSeconds();
} else {
if (attributes.getNamedItem("connection-timeout") != null && attributes.getNamedItem("connection-timeout").getNodeValue() != null) {
_connectionTimeout = Integer.parseInt(attributes.getNamedItem("connection-timeout").getNodeValue()) * 1000;
}
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.IsSet(ConnectionStrings.RETRYCONNECTIONDELAY)) {
_retryConnectionDelay = (int) _cacheConnectionOptions.getRetryConnectionDelay().getTotalMinutes();
} else {
if (attributes.getNamedItem("retry-connection-delay") != null && attributes.getNamedItem("retry-connection-delay").getNodeValue() != null) {
_retryConnectionDelay = Integer.parseInt(attributes.getNamedItem("retry-connection-delay").getNodeValue()) / 60;
}
}
}
serverPortFound = true;
Node localServerIPNode = attributes.getNamedItem("local-server-ip");
if (localServerIPNode != null)
{
setLocalServerIP((localServerIPNode.getTextContent()));
}
}
}
if(!_loadCacheConfiguration) return;
if (!serverPortFound) {
throw new ConfigurationException("ncache-server missing in client confiugration");
}
NodeList cacheNL = response.getElementsByTagName("cache");
if (cacheNL.getLength() == 0) {
throw new Exception("'client.ncconf' not found or does not contain server information");
}
NodeList serversNL = null;
Node cacheN = null;
for (int i = 0; i < cacheNL.getLength(); i++) {
cacheN = cacheNL.item(i);
NamedNodeMap attributes = cacheN.getAttributes();
if (attributes.getNamedItem("id").getNodeValue().toLowerCase().equals(_cacheId.toLowerCase())) {
if (attributes.getNamedItem("load-balance") != null) {
_balanceNodes = Boolean.parseBoolean(attributes.getNamedItem("load-balance").getNodeValue());
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getLoadBalance() != null) {
_balanceNodes = _cacheConnectionOptions.getLoadBalance();
}
if (attributes.getNamedItem("client-cache-id") != null) {
setClientCacheID(attributes.getNamedItem("client-cache-id").getNodeValue());
}
if (attributes.getNamedItem("default-readthru-provider") != null) {
_defaultReadThruProvider = attributes.getNamedItem("default-readthru-provider").getNodeValue();
}
if (_cacheConnectionOptions != null && !isNullOrEmpty(_cacheConnectionOptions.getDefaultReadThruProvider())) {
_defaultReadThruProvider = _cacheConnectionOptions.getDefaultReadThruProvider();
}
if (attributes.getNamedItem("default-writethru-provider") != null) {
_defaultWriteThruProvider = attributes.getNamedItem("default-writethru-provider").getNodeValue();
}
if (_cacheConnectionOptions != null && !isNullOrEmpty(_cacheConnectionOptions.getDefaultWriteThruProvider())) {
_defaultWriteThruProvider = _cacheConnectionOptions.getDefaultWriteThruProvider();
}
if (attributes.getNamedItem("client-cache-syncmode") != null) {
_clientCacheSyncMode = attributes.getNamedItem("client-cache-syncmode").getNodeValue().toLowerCase().equals("pessimistic") ? ClientCacheSyncMode.Pessimistic : ClientCacheSyncMode.Optimistic;
}
if (attributes.getNamedItem("reconnect-client-cache-interval") != null && attributes.getNamedItem("skip-client-cache-if-unavailable").getNodeValue() != null) {
_retryL1ConnectionInterval= Integer.parseInt(attributes.getNamedItem("reconnect-client-cache-interval").getNodeValue());
}
if (attributes.getNamedItem("skip-client-cache-if-unavailable") != null) {
_skipUnAvailableClientCache= Boolean.parseBoolean(attributes.getNamedItem("skip-client-cache-if-unavailable").getNodeValue());
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getClientCacheMode() != null) {
_clientCacheSyncMode = _cacheConnectionOptions.getClientCacheMode();
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getEnableClientLogs() != null) {
setEnableClientLogs(_cacheConnectionOptions.getEnableClientLogs());
} else {
if (attributes.getNamedItem("enable-client-logs") != null) {
setEnableClientLogs(Boolean.parseBoolean(attributes.getNamedItem("enable-client-logs").getNodeValue()));
}
}
if (_cacheConnectionOptions != null && _cacheConnectionOptions.getLogLevel() != null) {
setLogLevels(_cacheConnectionOptions.getLogLevel());
switch (getLogLevels()) {
case Debug:
case Info:
setEnableDetailedClientLogs(true);
break;
case Error:
setEnableDetailedClientLogs(false);
break;
}
} else {
if (attributes.getNamedItem("log-level") != null) {
String logLevel = attributes.getNamedItem("log-level").getNodeValue().toLowerCase();
if (logLevel.equals("info")) {
setLogLevels(Info);
setEnableDetailedClientLogs(true);
} else if (logLevel.equals("debug")) {
setLogLevels(Debug);
setEnableDetailedClientLogs(true);
} else if (logLevel.equals("error")) {
setLogLevels(Error);
setEnableDetailedClientLogs(false);
}
}
}
setImportHashmap(true);
serversNL = cacheN.getChildNodes();
break;
}
}
if (serversNL == null) {
if (!isNullOrEmpty(_cacheId)) {
if (_result != Search.GlobalSearch) {
_search = Search.forValue(_result.getValue() + 1);
loadConfiguration();
}
}
return;
}
_search = _result;
loadRemoteServerMappingConfig(serversNL);
loadRemoteServerConfig(serversNL);
} catch (Exception e) {
throw new ConfigurationException(e.getMessage());
}
}
private String getConfigPath() throws Exception {
String separator = System.getProperty("file.separator");
String path = "";
//Making SetConfigPath property available on Windows.
path = CacheManager.getConfigPath();
if (path != null && path.equalsIgnoreCase("") == false) {
if (!path.endsWith(separator)) {
path = path.concat(separator);
}
return path.concat(_configFile);
}
if (System.getProperty("os.name").toLowerCase().startsWith("win")) {
//
//get current execution path
path = System.getProperty("user.dir");
if (path != null) {
if (!path.endsWith(separator)) {
path = path.concat(separator);
}
path = path.concat(_configFile);
if (fileExists(path)) {
return path;
}
}
//get ncache installation path
path = getInstallDir();
if (path != null) {
if (path != null) {
if (!path.endsWith(separator)) {
path = path.concat(separator);
}
path = path.concat("config" + separator + _configFile);
if (fileExists(path)) {
return path;
}
}
}
String delim = "ncache" + separator + "bin";
String librarypath = System.getProperty("java.library.path");
String[] tokans = librarypath.toLowerCase().split(System.getProperty("path.separator"));
for (int i = 0; i < tokans.length; i++) {
if (tokans[i].contains(delim)) {
path = tokans[i].substring(0, tokans[i].lastIndexOf("bin"));
path += "config";
break;
}
}
//
} else {
//
//path = System.getenv("NCACHE_ROOT");
path = CacheManager.getConfigPath();
if (path != null && path.equalsIgnoreCase("") == false) {
return path.concat(separator + _configFile);
} else {
path = ServicePropValues.getTGHome();
if (path != null && path.equalsIgnoreCase("") == false) {
path = path.concat(separator + "config");
} else {
path = ServicePropValues.INSTALLDIR_DIR;
if (path != null && path.equalsIgnoreCase("") == false) {
path = path.concat(separator + "config");
}
//
}
if (path == null || path.equalsIgnoreCase("") == true) {
path = "/opt/ncache/config/" + _configFile;
if (fileExists(path))
return path;
path = System.getProperty("user.dir");
if (path != null) {
if (!path.endsWith(separator)) {
path = path.concat(separator);
}
path = path.concat(_configFile);
if (fileExists(path)) {
return path;
}
}
}
}
}
if (path == null) {
throw new Exception("Unable to find " + _configFile + "; please reset Enviorment variables");
}
return path.concat(separator + _configFile);
}
private boolean fileExists(String path) {
File check = new File(path);
try {
return check.exists();
} catch (SecurityException se) {
}
return false;
}
private String getInstallDir() {
if (_installDir == null) {
_installDir = ServicePropValues.getTGHome();
}
return _installDir;
}
public void loadLocalServerIP() throws ConfigurationException {
try {
tangible.OutObject tempOut_Result = new tangible.OutObject();
String path = DirectoryUtil.getBaseFilePath("client.ncconf", _search, tempOut_Result);
File f = new File(path);
if (!f.exists()) {
throw new Exception("'client.ncconf' not found or does not contain server information");
}
DocumentBuilderFactory builderFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = builderFactory.newDocumentBuilder();
Document response = builder.parse(f);
NodeList serverPortNL = response.getElementsByTagName("ncache-server");
if (serverPortNL == null && serverPortNL.getLength() < 0) return;
Node ncacheServerDetails = serverPortNL.item(0);
if (ncacheServerDetails == null) return;
NamedNodeMap attributes = ncacheServerDetails.getAttributes();
if (attributes == null) return;
Node localServerIPNode = attributes.getNamedItem("local-server-ip");
if (localServerIPNode == null) return ;
setLocalServerIP((localServerIPNode.getTextContent()));
}catch(Exception ex)
{
throw new ConfigurationException(ex.toString());
}
}
//endregion
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy