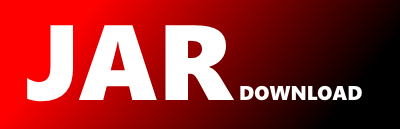
com.alachisoft.ncache.client.internal.util.ConfigReader Maven / Gradle / Ivy
/*
* ConfigReader.java
*
* Created on September 20, 2006, 9:40 AM
*
* Copyright 2005 Alachisoft, Inc. All rights reserved.
* ALACHISOFT PROPRIETARY/CONFIDENTIAL. Use is subject to license terms.
*/
package com.alachisoft.ncache.client.internal.util;
import com.alachisoft.ncache.runtime.exceptions.ConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
/**
* @author Administrator
*/
public class ConfigReader {
String fileName;
public ConfigReader() {
}
public static String ClientCacheID(String fileName, String cacheId, tangible.RefObject isPessimistic) throws Exception {
File file = null;
try {
String path = GetClientConfigurationPath();
file = new File(path);
if (!file.exists()) {
return "";
}
Document configuration = null;
DocumentBuilderFactory builderFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = null;
try {
builder = builderFactory.newDocumentBuilder();
} catch (ParserConfigurationException ex) {
}
configuration = builder.parse(file);
NodeList cacheList = configuration.getElementsByTagName("cache");
for (int i = 0; i < cacheList.getLength(); i++) {
Node cache = cacheList.item(i);
if (cache.hasAttributes()) {
if (cache.getAttributes().getNamedItem("id").getNodeValue().equalsIgnoreCase(cacheId)) {
if (cache.getAttributes().getNamedItem("client-cache-syncmode") != null) {
isPessimistic.argvalue = cache.getAttributes().getNamedItem("client-cache-syncmode").getNodeValue().equalsIgnoreCase("pessimistic");
}
if (cache.getAttributes().getNamedItem("client-cache-id").getNodeValue().toLowerCase().length() != 0) {
return cache.getAttributes().getNamedItem("client-cache-id").getNodeValue();
}
}
}
}
}
catch (Exception e) {
throw new ConfigurationException("An error occured while reading client.ncconf. " + e.getMessage());
} finally {
}
return "";
}
public static java.util.HashMap ReadSecurityParams(String fileName, String cacheId) throws ConfigurationException {
java.util.HashMap securityParams = null;
try {
String filePath = GetClientConfigurationPath();
if (filePath == null) {
return null;
}
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document document = db.parse(new File(filePath));
Element docEle = document.getDocumentElement();
NodeList cacheList = docEle.getElementsByTagName("cache");
NodeList cacheConfig = null;
if (cacheList != null && cacheList.getLength() > 0) {
for (int nodeItem = 0; nodeItem < cacheList.getLength(); nodeItem++) {
Node cacheNode = cacheList.item(nodeItem);
if (cacheNode.getNodeType() == Node.ELEMENT_NODE) {
Element cacheElement = (Element) cacheNode;
if (cacheId.equals(cacheElement.getAttribute("id"))) {
cacheConfig = cacheNode.getChildNodes();
break;
}
}
}
}
if (cacheConfig == null) {
return null;
}
if (cacheConfig != null && cacheConfig.getLength() > 0) {
for (int nodeItem = 0; nodeItem < cacheConfig.getLength(); nodeItem++) {
Node currentConfig = cacheConfig.item(nodeItem);
if (currentConfig.getNodeType() == Node.ELEMENT_NODE) {
Element cacheElement = (Element) currentConfig;
if (cacheElement.getTagName().equals("security")) {
NodeList securityData = currentConfig.getChildNodes();
if (securityData != null) {
securityParams = new java.util.HashMap();
java.util.HashMap tmp = null;
for (int j = 0; j < securityData.getLength(); j++) {
Node securitUser = securityData.item(j);
if (securitUser.getNodeType() == Node.ELEMENT_NODE) {
Element primaryElement = (Element) securitUser;
String tempVar = primaryElement.getTagName();
if (tempVar.equals("primary")) {
tmp = new java.util.HashMap();
securityParams.put("pri-user", tmp);
tmp.put("user-id", primaryElement.getAttribute("user-id"));
tmp.put("password", primaryElement.getAttribute("password"));
}
else if (tempVar.equals("secondary")) {
tmp = new java.util.HashMap();
securityParams.put("sec-user", tmp);
tmp.put("user-id", primaryElement.getAttribute("user-id"));
tmp.put("password", primaryElement.getAttribute("password"));
} else {
throw new com.alachisoft.ncache.runtime.exceptions.ConfigurationException("Invalid XmlNode \'" + primaryElement.getTagName()
+ "\' found in security section");
}
}
}
return securityParams;
}
return null;
}
}
}
}
}
catch (com.alachisoft.ncache.runtime.exceptions.ConfigurationException ex) {
throw ex;
} catch (Exception e) {
throw new ConfigurationException("An error occured while reading client.ncconf. " + e.getMessage());
}
return securityParams;
}
private static String GetClientConfigurationPath() {
return DirectoryUtil.getBaseFilePath("client.ncconf");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy