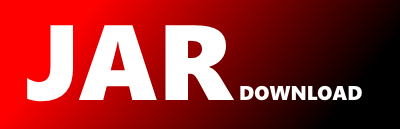
com.alachisoft.ncache.client.services.MessagingService Maven / Gradle / Ivy
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.client.services;
import com.alachisoft.ncache.client.CacheDataModificationListener;
import com.alachisoft.ncache.client.CacheEventDescriptor;
import com.alachisoft.ncache.client.ContinuousQuery;
import com.alachisoft.ncache.runtime.caching.Topic;
import com.alachisoft.ncache.runtime.caching.messaging.TopicPriority;
import com.alachisoft.ncache.runtime.caching.messaging.TopicSearchOptions;
import com.alachisoft.ncache.runtime.events.EventDataFilter;
import com.alachisoft.ncache.runtime.events.EventType;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import java.util.EnumSet;
import java.util.concurrent.FutureTask;
/**
* This interface contains properties and methods required for Messaging Service.
*/
public interface MessagingService {
/**
* Retrieves the topic instance against the topic name.
* @param topicName Name to identify topic.
* @return Returns the topic instance, null if it does not exist.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
Topic getTopic(String topicName) throws CacheException,IllegalArgumentException;
/**
* Retrieves the topic instance against the {@link com.alachisoft.ncache.runtime.caching.messaging.TopicSearchOptions} and provided name or pattern.
* @param topicName Name or pattern to identify topic.
* @param searchOptions {@link TopicSearchOptions} specifies to search topic by name or pattern.
* @return Returns the topic instance, null if it does not exist.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
Topic getTopic(String topicName, TopicSearchOptions searchOptions) throws CacheException,IllegalArgumentException;
/**
* Creates and retrieves the topic instance against the specified topic name and priority.
* @param topicName Name to identify the topic.
* @param topicPriority Specifies the relative priority of the topic stored in cache.
* @return The topic instance.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
Topic createTopic(String topicName, TopicPriority topicPriority) throws CacheException,IllegalArgumentException;
/**
* Creates and retrieves the topic instance against the specified topic name and priority TopicPriority.Normal.
* @param topicName Name to identify the topic.
* @return The topic instance.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
Topic createTopic(String topicName) throws CacheException,IllegalArgumentException;
/**
* Deletes the topic instance against the specified topic name.
* @param topicName Name to identify topic.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
void deleteTopic(String topicName) throws CacheException,IllegalArgumentException;
/**
* Deletes the topic instance asynchronously against the specified topic name.
* @param topicName Name to identify topic.
* @return Future task that performs a delete topic operation in background.Methods of {@link java.util.concurrent.FutureTask} can be used to determine status of the task i.e. isDone(), isCancelled().
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
FutureTask deleteTopicAsync(String topicName) throws CacheException,IllegalArgumentException;
/**
* Registers the specified continuous query with the cache server. You can use this method multiple times in your application depending on its need to receive the notifications for a change in the dataset of your query.
* This method takes as argument an object of ContinuousQuery which has the query and the listeners registered to it.
* @param query SQL-like query to be executed on cache.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
void registerCQ(ContinuousQuery query) throws CacheException,IllegalArgumentException;
/**
* Registers cache notification {@link EventType} of type item added, updated or removed against specified key in cache.
* @param key Unique key to identify the cache item.
* @param listener The specific method of interface {@link CacheDataModificationListener} is invoked when specified event/events are triggered against specified key in cache.
* @param eventTypes An Enumset that specifies which event/ events are to be registered against the key.
* @param eventDataFilter Tells whether to receive metadata, data with metadata or none when a notification is triggered.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
void addCacheNotificationListener(String key, CacheDataModificationListener listener, EnumSet eventTypes, EventDataFilter eventDataFilter) throws CacheException,IllegalArgumentException;
/**
* Registers cache notification against specified keys in cache.
* @param keys {@link Iterable} list of keys to identify the cache item.
* @param listener The specific method of interface {@link CacheDataModificationListener} is invoked when specified event/events are triggered against specified key in cache.
* @param eventTypes An Enumset that specifies which event/ events are to be registered against the key.
* @param eventDataFilter Tells whether to receive metadata, data with metadata or none when a notification is triggered.
* @throws CacheException
* @throws IllegalArgumentException
*/
void addCacheNotificationListener(Iterable keys, CacheDataModificationListener listener, EnumSet eventTypes, EventDataFilter eventDataFilter) throws CacheException,IllegalArgumentException;
/**
* Registers cache notification of specified event types against all keys in the cache.
* @param listener The specific method of interface {@link CacheDataModificationListener} is invoked when specified event/events are triggered against specified key in cache.
* @param eventTypes An Enumset that specifies which event/ events are to be registered against the key.
* @param eventDataFilter Tells whether to receive metadata, data with metadata or none when a notification is triggered.
* @return Instance of {@link CacheEventDescriptor} required to unregister the notifications.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
CacheEventDescriptor addCacheNotificationListener(CacheDataModificationListener listener, EnumSet eventTypes, EventDataFilter eventDataFilter) throws CacheException,IllegalArgumentException;
/**
* Unregisters an already registered continuous query to deactivate it on the cache server. Like registerCQ, it takes as argument an object of ContinuousQuery to unregister the listeners which are no more fired after this call.
* @param query SQL-like query to be executed over the cache.
* @throws Exception So you can catch this exception for all the exceptions thrown from within the NCache.
*/
void unRegisterCQ(ContinuousQuery query) throws Exception;
/**
* Unregisters a cache level event that may have been registered.
* @param descriptor The descriptor returned when the general event was registered.
* @throws Exception So you can catch this exception for all the exceptions thrown from within the NCache.
*/
void removeCacheNotificationListener(CacheEventDescriptor descriptor) throws Exception;
/**
* Unregisters the {@link CacheDataModificationListener} already registered for the specified key.
* @param key Unique key to identify the cache item.
* @param listener The {@link CacheDataModificationListener} that is invoked when specified event/events are triggered in cache.
* @param eventTypes An Enumset that specifies which event/ events are to be unregistered against the key.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
void removeCacheNotificationListener(String key, CacheDataModificationListener listener, EnumSet eventTypes) throws CacheException,IllegalArgumentException;
/**
* Unregisters cache notification against specified keys in cache.
* @param keys {@link Iterable} list of keys to identify the cache item.
* @param listener The {@link CacheDataModificationListener} that is invoked when specified event/events are triggered in cache.
* @param eventTypes An Enumset that specifies which event/ events are to be unregistered against the key.
* @throws CacheException So you can catch this exception for all the exceptions thrown from within the NCache.
* @throws IllegalArgumentException
*/
void removeCacheNotificationListener(Iterable keys, CacheDataModificationListener listener, EnumSet eventTypes) throws CacheException,IllegalArgumentException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy