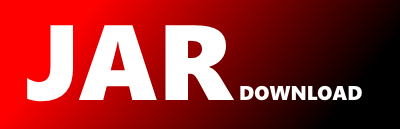
com.alachisoft.ncache.client.services.SearchService Maven / Gradle / Ivy
package com.alachisoft.ncache.client.services;
// Copyright (c) 2019 Alachisoft
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License
import com.alachisoft.ncache.client.CacheReader;
import com.alachisoft.ncache.client.QueryCommand;
import com.alachisoft.ncache.client.TagSearchOptions;
import com.alachisoft.ncache.runtime.caching.Tag;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* This interface contains properties and methods required for Search Service.
*/
public interface SearchService {
///#region execute reader
/**
* Executes Delete statements on cache. Returns number of affected rows after query is executed.
* @param queryCommand {@link QueryCommand} containing query text and values.
* @return Number of rows affected after query is executed.
* @throws CacheException
* @throws IllegalArgumentException
*/
int executeNonQuery(QueryCommand queryCommand) throws CacheException,IllegalArgumentException;
/**
* Performs search on the cache based on the query specified. Returns list of key-value pairs in a data reader which fulfills the query criteria. This key-value pair has cache key and its respective value.You can specify the flag for specifying if you want data with keys.
* @param queryCommand {@link QueryCommand} containing query text and values.
* @return Reads forward-only stream of result sets of the query executed on cache.
* @throws CacheException
* @throws IllegalArgumentException
*/
CacheReader executeReader(QueryCommand queryCommand) throws CacheException,IllegalArgumentException;
/**
* Performs search on the cache based on the query specified. Returns list of key-value pairs in a data reader which fulfills the query criteria. This key-value pair has cache key and its respective value.You can specify the flag for specifying if you want data with keys.
* @param queryCommand {@link QueryCommand} containing query text and values.
* @param getData Flag to indicate whether the resulting values have to be returned with keys or not.
* @param chunkSize Size of data/keys packets received after search, default value is 512*1024 KB.
* @return Reads forward-only stream of result sets of the query executed on cache.
* @throws CacheException
* @throws IllegalArgumentException
*/
CacheReader executeReader(QueryCommand queryCommand, boolean getData, int chunkSize) throws CacheException,IllegalArgumentException;
/**
* Executes the query, and returns the first column of the first row in the result set returned by the query. Additional columns or rows are ignored.
* @param queryCommand {@link QueryCommand} containing query text and values.
* @param cls Specifies the class of value obtained from the cache.
* @param Specifies the type of value obtained from the cache.
* @return The first column of the first row in the result set, or null if the result set is empty.
* @throws CacheException
* @throws IllegalArgumentException
*/
T executeScalar(QueryCommand queryCommand, Class> cls) throws CacheException,IllegalArgumentException;
///#endregion
///#region Tag Based Operations
/**
* Removes the cached objects with the specified tag.
* @param tag A Tag to search cache with.
* @throws CacheException
* @throws IllegalArgumentException
*/
void removeByTag(Tag tag) throws CacheException,IllegalArgumentException;
/**
* Removes the cached objects that have tags with specified {@link TagSearchOptions}.
* @param tags List of tags to search cache with.
* @param type {@link TagSearchOptions} specifies the search type for the tags.
* @throws CacheException
* @throws IllegalArgumentException
*/
void removeByTags(List tags, TagSearchOptions type) throws CacheException,IllegalArgumentException;
/**
* Gets all the cached items with the specified tag.
* @param tag Name of tag to search the cache items with.
* @param Specifies the type of value obtained from the cache.
* @return A Map containing the cache keys and associated objects with the type specified.
* @throws CacheException
* @throws IllegalArgumentException
*/
java.util.Map getByTag(Tag tag) throws CacheException,IllegalArgumentException;
/**
* Gets all the cached objects with the wild card supported tag.
* @param wildCardExpression The wild card Expression to search with.
* @param Specifies the type of value obtained from the cache.
* @return A Map containing the cache keys and associated objects with the type specified.
* @throws CacheException
* @throws IllegalArgumentException
*/
java.util.Map getByTag(String wildCardExpression) throws CacheException,IllegalArgumentException;
/**
* Returns the cached objects that have tags with specified {@link TagSearchOptions}.
* @param tags List of tags to search cache with.
* @param type {@link TagSearchOptions} specifies the search type for the tags
* @param Specifies the type of value obtained from the cache.
* @return A Map containing the cache keys and associated objects with the type specified.
* @throws CacheException
* @throws IllegalArgumentException
*/
java.util.Map getByTags(List tags, TagSearchOptions type) throws CacheException,IllegalArgumentException;
/**
* Gets all keys of the objects with the specified tag.
* @param tag The tag to search the cache with.
* @return Collection containing the cache keys.
* @throws CacheException
* @throws IllegalArgumentException
*/
Collection getKeysByTag(Tag tag) throws CacheException,IllegalArgumentException;
/**
* Gets all the keys with the wild card supported tag.
* @param wildCardExpression The wild card Expression to search with.
* @return Collection containing the cache keys.
* @throws CacheException
* @throws IllegalArgumentException
*/
Collection getKeysByTag(String wildCardExpression) throws CacheException,IllegalArgumentException;
/**
* Returns keys of the cached items that have tags with specified {@link TagSearchOptions}.
* @param tags List of tags to search cache with.
* @param type {@link TagSearchOptions} specifies the search type for the tags.
* @return Collection containing the cache keys.
* @throws CacheException
* @throws IllegalArgumentException
*/
Collection getKeysByTags(List tags, TagSearchOptions type) throws CacheException,IllegalArgumentException;
///#endregion
///#region Group Based Operations
/**
* Remove the data items pertaining to the specified group from cache.
* @param group Name of group to be removed.
* @throws CacheException
* @throws IllegalArgumentException
*/
void removeGroupData(String group) throws CacheException,IllegalArgumentException;
/**
* Retrieves the keys of the items in the specified group.
* @param group Name of group whose keys are to be returned.
* @return Collection of keys of the group.
* @throws CacheException
* @throws IllegalArgumentException
*/
Collection getGroupKeys(String group) throws CacheException,IllegalArgumentException;
/**
* Retrieves the key and value pairs of the specified group.
* @param group Name of group whose data is to be returned.
* @param Specifies the type of value obtained from the cache.
* @return A Map containing key-value pairs of the specified group.
* @throws CacheException
* @throws IllegalArgumentException
*/
Map getGroupData(String group) throws CacheException,IllegalArgumentException;
///#endregion
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy