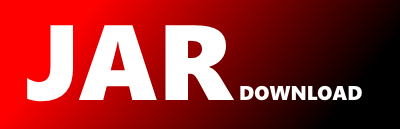
com.alachisoft.ncache.spring.NCacheCache Maven / Gradle / Ivy
/*
* Alachisoft (R) NCache Integrations
* NCache Provider for Spring
* ===============================================================================
* Copyright © Alachisoft. All rights reserved.
* THIS CODE AND INFORMATION IS PROVIDED "AS IS" WITHOUT WARRANTY
* OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING BUT NOT
* LIMITED TO THE IMPLIED WARRANTIES OF MERCHANTABILITY AND
* FITNESS FOR A PARTICULAR PURPOSE.
* ===============================================================================
*/
package com.alachisoft.ncache.spring;
import com.alachisoft.ncache.spring.configuration.SpringCacheConfiguration;
import com.alachisoft.ncache.client.CacheItem;
import com.alachisoft.ncache.client.CacheManager;
import com.alachisoft.ncache.runtime.caching.expiration.Expiration;
import com.alachisoft.ncache.runtime.caching.expiration.ExpirationType;
import com.alachisoft.ncache.runtime.caching.Tag;
import com.alachisoft.ncache.runtime.exceptions.CacheException;
import com.alachisoft.ncache.runtime.exceptions.ConfigurationException;
import com.alachisoft.ncache.runtime.exceptions.GeneralFailureException;
import com.alachisoft.ncache.runtime.exceptions.OperationFailedException;
import com.alachisoft.ncache.runtime.exceptions.runtime.CacheRuntimeException;
import com.alachisoft.ncache.runtime.util.TimeSpan;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.springframework.cache.Cache;
public class NCacheCache implements Cache {
private com.alachisoft.ncache.spring.configuration.SpringCacheConfiguration cacheConfig = null;
private com.alachisoft.ncache.client.Cache cache = null;
private String cacheName = null;
private Logger logger = null;
public NCacheCache(String cacheName, SpringCacheConfiguration cacheConfig, Logger logger) throws CacheException, GeneralFailureException, Exception {
this.cacheName = cacheName;
this.cacheConfig = cacheConfig;
this.logger = logger;
cache = CacheManager.getCache(cacheConfig.getCacheidInstanceName());
}
@Override
public String getName() {
return cacheName;
}
@Override
public Object getNativeCache() {
return cache;
}
@Override
public ValueWrapper get(Object key) {
try {
Object item = cache.get(getKey(key), Object.class);
if(item != null)
return new com.alachisoft.ncache.spring.CacheObject(item);
} catch (Exception ex) {
logger.log(Level.SEVERE, ex.getMessage());
}
return null;
}
@Override
public T get(Object key, Class type) {
try {
CacheItem item = cache.getCacheItem(getKey(key));
return item.getValue(type);
} catch (Exception ex){
throw new CacheRuntimeException(ex.hashCode(),ex.getMessage(),ex.getStackTrace().toString());
}
}
@Override
public T get(Object obj, Callable callable) {
try {
T val = (T) get(obj, Object.class);
if (val != null)
return val;
Object userObject = callable.call();
put(obj, userObject);
return (T) userObject;
}
catch (Exception ex){
throw new CacheRuntimeException(ex.hashCode(),ex.getMessage(),ex.getStackTrace().toString());
}
}
@Override
public void put(Object key, Object value) {
try {
cache.insert(getKey(key), getCacheItem(value));
} catch (Exception ex) {
logger.log(Level.SEVERE, ex.getMessage());
}
}
@Override
public ValueWrapper putIfAbsent(Object key, Object value) {
try
{
CacheItem item = cache.getCacheItem(getKey(key));
if (item == null)
{
put(key, value);
}
return new com.alachisoft.ncache.spring.CacheObject(item.getValue(Object.class));
}
catch (Exception ex)
{
logger.log(Level.SEVERE, ex.getMessage());
}
return null;
}
@Override
public void evict(Object key) {
try {
cache.delete(getKey(key));
} catch (Exception ex) {
logger.log(Level.SEVERE, ex.getMessage());
}
}
@Override
public boolean evictIfPresent(Object key) {
boolean result = false;
try
{
Object item = cache.remove(getKey(key), Object.class);// get(getKey(key));
if(item != null)
result = true;
}
catch (Exception ex)
{
logger.log(Level.SEVERE, ex.getMessage());
}
return result;
}
@Override
public void clear() {
try
{
cache.clear();
}
catch (Exception ex) {
logger.log(Level.SEVERE, ex.getMessage());
}
}
@Override
public boolean invalidate() {
boolean result = false;
try{
if(cache.getCount() > 0)
{
result = true;
cache.clear();
}
}
catch (Exception ex) {
logger.log(Level.SEVERE, ex.getMessage());
}
return result;
}
private String getKey(Object key) {
return cacheName + ":" + key.toString();
}
private CacheItem getCacheItem(Object value) {
CacheItem cItem = new CacheItem(value);
List tagList = new ArrayList();
tagList.add(new Tag(cacheName));
cItem.setTags(tagList);
if (cacheConfig != null) {
if (!cacheConfig.getExpirationType().isEmpty() && !cacheConfig.getExpirationType().equalsIgnoreCase("none")) {
Expiration expiration = new Expiration((cacheConfig.getExpirationType().equalsIgnoreCase("sliding"))? ExpirationType.Sliding : ExpirationType.Absolute, new TimeSpan(0, 0, cacheConfig.getExpirationPeriod()));
cItem.setExpiration(expiration);
}
}
return cItem;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy