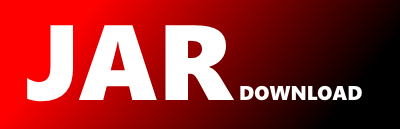
com.alachisoft.ncache.activate.cmdline.ActivateArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.activate.cmdline;
import com.alachisoft.ncache.common.commandline.ArgumentAttributeAnnotation;
import com.alachisoft.ncache.common.commandline.CommandLineArgs;
/**
* @author Muneeb_Shahid
*/
public class ActivateArgs extends CommandLineArgs {
private String licenseKey;
private String fName;
private String lName;
private String email;
private String company;
private String address;
private String city;
private String state;
private String country;
private String zip;
private String phone;
private String authCode;
private Boolean manual = false;
private Boolean reactivae = false;
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-a", fullNotation = "--authcode", appendText = "")
public String getAuthCode() {
return authCode;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-a", fullNotation = "--authcode", appendText = "")
public void setAuthCode(String authCode) {
this.authCode = authCode;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-k", fullNotation = "--licensekey", appendText = "")
public String getLicenseKey() {
return licenseKey;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-k", fullNotation = "--licensekey", appendText = "")
public void setLicenseKey(String licenseKey) {
this.licenseKey = licenseKey;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-f", fullNotation = "--firstname", appendText = "")
public String getfName() {
return fName;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-f", fullNotation = "--firstname", appendText = "")
public void setfName(String fName) {
this.fName = fName;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-l", fullNotation = "--lastname", appendText = "")
public String getlName() {
return lName;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-l", fullNotation = "--lastname", appendText = "")
public void setlName(String lName) {
this.lName = lName;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-e", fullNotation = "--email", appendText = "")
public String getEmail() {
return email;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-e", fullNotation = "--email", appendText = "")
public void setEmail(String email) {
this.email = email;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-c", fullNotation = "--company", appendText = "")
public String getCompany() {
return company;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-c", fullNotation = "--company", appendText = "")
public void setCompany(String company) {
this.company = company;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-r", fullNotation = "--reactivate", appendText = "")
public boolean getReactivate() {
return reactivae;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-r", fullNotation = "--reactivate", appendText = "")
public void setReactivate(boolean reactivae) {
this.reactivae = reactivae;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-t", fullNotation = "--city", appendText = "")
public String getCity() {
return city;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-t", fullNotation = "--city", appendText = "")
public void setCity(String city) {
this.city = city;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-s", fullNotation = "--state", appendText = "")
public String getState() {
return state;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-s", fullNotation = "--state", appendText = "")
public void setState(String state) {
this.state = state;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-y", fullNotation = "--country", appendText = "")
public String getCountry() {
return country;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-y", fullNotation = "--country", appendText = "")
public void setCountry(String country) {
this.country = country;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-z", fullNotation = "--zip", appendText = "")
public String getZip() {
return zip;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-z", fullNotation = "--zip", appendText = "")
public void setZip(String zip) {
this.zip = zip;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-p", fullNotation = "--phone", appendText = "")
public String getPhone() {
return phone;
}
@ArgumentAttributeAnnotation(defaultValue = "", shortNotation = "-p", fullNotation = "--phone", appendText = "")
public void setPhone(String phone) {
this.phone = phone;
}
@ArgumentAttributeAnnotation(defaultValue = "false", shortNotation = "-m", fullNotation = "--manual", appendText = "")
public Boolean getManual() {
return manual;
}
@ArgumentAttributeAnnotation(defaultValue = "false", shortNotation = "-m", fullNotation = "--manual", appendText = "")
public void setManual(Boolean manual) {
this.manual = manual;
}
@Override
public void printUsage() {
StringBuilder usageBuilder = new StringBuilder();
usageBuilder.append("Usage: -k key -f first-name -l last-name -e email [option[...]].").append("\n");
usageBuilder.append("Argument:").append("\n").append("\n");
usageBuilder.append(" ").append("-k --licensekey [licensekey]").append("\n");
usageBuilder.append(" \t").append("Specify the license key recieved from Alachisoft").append("\n").append("\n");
usageBuilder.append(" ").append("-f --firstname [first-name]").append("\n");
usageBuilder.append(" \t").append("Specify the user's first name").append("\n").append("\n");
usageBuilder.append(" ").append("-l --lastname [last-name]").append("\n");
usageBuilder.append(" \t").append("Specfiy the user's last name" + "").append("\n").append("\n");
usageBuilder.append(" ").append("-e --email [email]").append("\n");
usageBuilder.append(" \t").append("Specify the user's email address").append("\n").append("\n");
usageBuilder.append(" ").append("Optional").append("\n");
usageBuilder.append(" ").append("-m --manual [manual]").append("\n");
usageBuilder.append(" \t").append("Enable manual mode.").append("\n").append("\n");
usageBuilder.append(" ").append("-r --reactivate [reactivate]").append("\n");
usageBuilder.append(" \t").append("Enable reactivation mode.").append("\n");
usageBuilder.append(" ").append("-a --authcode [authorization-code]").append("\n");
usageBuilder.append(" \t").append("Specify the authcode, recieved from Alachisoft in order to manually activate NCache.").append("\n").append("\n");
usageBuilder.append(" ").append("-c --company [company]").append("\n");
usageBuilder.append(" \t").append("Specify the user's company name").append("\n").append("\n");
usageBuilder.append(" ").append("-t --city [city]").append("\n");
usageBuilder.append(" \t").append("Specify the name of the city.").append("\n").append("\n");
usageBuilder.append(" ").append("-s --state [state]").append("\n");
usageBuilder.append(" \t").append("Specify the name of the state.").append("\n").append("\n");
usageBuilder.append(" ").append("-y --country [country]").append("\n");
usageBuilder.append(" \t").append("Specify the country").append("\n").append("\n");
usageBuilder.append(" ").append("-z --zip [zip]").append("\n");
usageBuilder.append(" \t").append("Specify postal/zip code").append("\n").append("\n");
usageBuilder.append(" ").append("-p --phone [phone]").append("\n");
usageBuilder.append(" \t").append("Specify the phone number").append("\n").append("\n");
System.out.print(usageBuilder.toString());
super.printUsage();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy