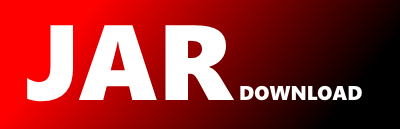
com.alachisoft.ncache.activate.cmdline.ActivateTool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.activate.cmdline;
import com.alachisoft.ncache.ncactivate.cmdline.LicenseTool;
import com.alachisoft.ncache.ncactivate.cmdline.exceptions.CorruptInstallationException;
import com.alachisoft.ncache.ncactivate.crypto.Crypto;
import com.alachisoft.ncache.ncactivate.utils.*;
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
/**
* @author Muneeb_Shahid
*/
public class ActivateTool extends LicenseTool {
public ActivateTool() throws CorruptInstallationException {
super();
}
@Override
public void Run(String[] args) {
if (super.intialize(args, new ActivateArgs())) {
super.pringLogo();
if (!super.printUsage()) {
try {
Thread.sleep(5);
} catch (InterruptedException ex) {
}
this.Run();
}
}
}
@Override
protected void Run() {
RegUtil.LoadRegistry();
ActivateArgs cmdArgs = (ActivateArgs) super.getCmdArgs();
super.getAppUtil().getProductInfo().LicenseKey = getValue(cmdArgs.getLicenseKey());
super.getAppUtil().getUserInfo().FirstName = getValue(cmdArgs.getfName());
super.getAppUtil().getUserInfo().LastName = getValue(cmdArgs.getlName());
super.getAppUtil().getUserInfo().EmailAddr = getValue(cmdArgs.getEmail());
super.getAppUtil().getUserInfo().Company = getValue(cmdArgs.getCompany());
//super.getAppUtil().getUserInfo().Address1 = getValue(cmdArgs.getAddress());
super.getAppUtil().getProductInfo().Reactivation = cmdArgs.getReactivate();
super.getAppUtil().getUserInfo().City = getValue(cmdArgs.getCity());
super.getAppUtil().getUserInfo().State = getValue(cmdArgs.getState());
super.getAppUtil().getUserInfo().Country = getValue(cmdArgs.getCountry());
super.getAppUtil().getUserInfo().Zip = getValue(cmdArgs.getZip());
super.getAppUtil().getUserInfo().Phone = getValue(cmdArgs.getPhone());
if (cmdArgs.getAuthCode() != null) {
try {
AppUtil.VerifyResult result = super.getAppUtil().VerifAuthKey(cmdArgs.getAuthCode());
if (result != AppUtil.VerifyResult.Success) {
System.out.println("Invalid activation key specified");
}
super.getAppUtil().Activate(cmdArgs.getAuthCode());
System.out.println("Product has been activated successfully. Execute verifylicense tool for further verification.");
} catch (Exception exception) {
System.err.println(exception.getMessage());
}
} else {
java.util.HashMap requiredParams = new java.util.HashMap();
requiredParams.put("first name", cmdArgs.getfName());
requiredParams.put("last name", cmdArgs.getlName());
requiredParams.put("license key", cmdArgs.getLicenseKey());
requiredParams.put("email", cmdArgs.getEmail());
if (super.checkRequiredParams(requiredParams)) {
if (cmdArgs.getManual()) {
System.out.println("Please copy the following code and email to Alachisoft sales personnel or to [email protected] \n\n" + super.getMachineInfoCode());
super.getAppUtil().getActivationData().Save();
RegUtil.StoreRegistry();
} else if (this.onlineActivation() > 0) {
super.getAppUtil().getActivationData().User.Save();
}
}
}
}
private String getValue(String arg) {
return arg == null ? "" : arg;
}
private int onlineActivation() {
String strHeaders = new String("Content-Type: application/x-www-form-urlencoded\r\n");
if (!super.getAppUtil().getProductInfo().Reactivation)
super.getAppUtil().getProductInfo().ActId = EnvironmentUtil.NewGUID();
String actXml = super.getAppUtil().getActivationData().ToLinearText();
String edition = super.getAppUtil().getActivationData().Product.Edition;
String strFormData = String.format("activationText=%s&Edition=%s", WebUtil.UrlEncode(Crypto.Encode(actXml)), edition);
System.out.println("Contacting license server...");
try {
// Change following host to an available webserver/resource
URL postUrl = new URL(WebUtil.URL);
HttpURLConnection con = (HttpURLConnection) postUrl.openConnection();
con.setDoOutput(true);
con.setRequestMethod("POST");
//con.setRequestProperty("Host", "20.200.20.10:83");
con.setRequestProperty("Host", "app.alachisoft.com");
con.setRequestProperty("Content-type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(strFormData.length()));
DataOutputStream connOut = new DataOutputStream(con.getOutputStream());
connOut.writeBytes(strFormData);
connOut.flush();
connOut.close();
int rc = con.getResponseCode();
String strMsg = super.getStringTableProps().getProperty(String.valueOf(rc + 1000));
if (rc >= 400 && rc <= 599) {
System.out.println("Request/Server Error: Status Code = " + String.valueOf(rc));
System.out.println("Message: " + strMsg);
return -1;
}
System.out.println("Status Code = " + String.valueOf(rc));
System.out.println("Message: " + strMsg + "\n");
System.out.println("Requesting validation...");
System.out.println("Waiting for status...");
DataInputStream connIn = new DataInputStream(con.getInputStream());
//BufferedReader d = new BufferedReader(new InputStreamReader(con.getInputStream()));
BufferedReader d = new BufferedReader(new InputStreamReader(connIn));
//System.err.println("Waiting for response!");
while (!d.ready()) {
// System.out.println(d.ready());
}
//System.err.println("Got the response!");
char tmpChar;
String serverResp = new String("");
char noChar = (char) -1;
do {
tmpChar = (char) d.read();
serverResp += tmpChar;
}
while (tmpChar != noChar);
//AfxMessageBox(serverResp);//just for debugging
//String serverResp = new String("The file is not writable.The file is not writable.dVQ00JemN9dg8EDgpyfGN+dXZ2fn58f3R/fXwha2hpY= Thank you for registering your copy of NCache. ?");
StringRef extractedMessage = new StringRef();
XmlUtil.ExtractFromXML("", serverResp, extractedMessage);
//System.err.println("Stage 2");
if (serverResp.indexOf("") != -1) {
System.out.println("\n" + extractedMessage.strData);
//AfxMessageBox(extractedMessage, MB_OK|MB_ICONERROR);
return -2;
}
//System.err.println("Stage 3");
StringRef szAuthKey = new StringRef();
//System.err.println("Extract from XML");
XmlUtil.ExtractFromXML("", serverResp, szAuthKey);
//System.err.println("Stage 3:1 Verifying Auth Key");
AppUtil.VerifyResult result = super.getAppUtil().VerifAuthKey(szAuthKey.strData);
if (result != AppUtil.VerifyResult.Success) {
//System.err.println("Stage 3:1 Verifying Auth Verification Failed" + result);
System.out.println("Invalid activation key specified");
//AfxMessageBox(strMsg, MB_OK|MB_ICONERROR);
return -3;
}
//System.err.println("Stage 4");
if (super.getAppUtil().Activate(szAuthKey.strData) == 0) {
System.out.println("\n" + extractedMessage.strData + "\n");
}
} catch (Exception e) {
System.out.println("Connection failed: " + e.getMessage());
return -4;
}
return 1;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy