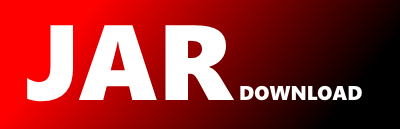
com.alachisoft.ncache.deactivate.cmdline.DeactivateTool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.deactivate.cmdline;
import com.alachisoft.ncache.licensing.config.InfoType;
import com.alachisoft.ncache.ncactivate.cmdline.LicenseTool;
import com.alachisoft.ncache.ncactivate.cmdline.exceptions.CorruptInstallationException;
import com.alachisoft.ncache.ncactivate.crypto.Crypto;
import com.alachisoft.ncache.ncactivate.utils.*;
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
/**
* @author Muneeb_Shahid
*/
public class DeactivateTool extends LicenseTool {
public DeactivateTool() throws CorruptInstallationException {
super();
}
@Override
public void Run(String[] args) {
if (super.intialize(args, new DeactivateArgs())) {
super.pringLogo();
if (!super.printUsage()) {
try {
Thread.sleep(5);
} catch (InterruptedException ex) {
}
this.Run();
}
}
}
@Override
public void Run() {
DeactivateArgs cmdArgs = (DeactivateArgs) super.getCmdArgs();
java.util.HashMap requiredParams = new java.util.HashMap();
requiredParams.put("deactivation code", cmdArgs.getDeactivateKey());
if (checkRequiredParams(requiredParams)) {
//First thing is to update STUB
try {
super.getAppUtil().DeactStub();
} catch (Exception exception) {
System.err.println("ERROR: " + exception.getMessage());
System.exit(1);
}
RegUtil.setKey(InfoType.LICENSE, RegKeys.DeactivateKey, cmdArgs.getDeactivateKey());
RegUtil.StoreRegistry();
super.getAppUtil().getActivationData().Load();
super.getAppUtil().DeactKey(RegUtil.getKey(InfoType.PRODUCT, RegKeys.DeactCode));
if (cmdArgs.getManual()) {
System.out.println("Please copy the following code and email to Alachisoft sales personnel or to [email protected] \n\n" + super.getMachineInfoCode());
} else {
this.onlineDeactivation();
}
}
}
public void onlineDeactivation() {
//int res = AfxMessageBox(_T("Please make sure that you have entered ")
// _T("correct information.\r\nProceed with activation process ?"),
// MB_YESNO|MB_ICONQUESTION|MB_DEFBUTTON2);
//if(res != IDYES) return;
String strHeaders = new String("Content-Type: application/x-www-form-urlencoded\r\n");
String actXml = super.getAppUtil().getActivationData().ToLinearText();
String edition = super.getAppUtil().getActivationData().Product.Edition;
String strFormData = String.format("activationText=%s&Edition=%s&Action=%s", WebUtil.UrlEncode(Crypto.Encode(actXml)), edition, "deactivation");
StringBuilder deactivationConsole = new StringBuilder();
System.out.println("Contacting license server...\r\n");
try {
// Change following host to an available webserver/resource
URL postUrl = new URL(WebUtil.URL);
HttpURLConnection con = (HttpURLConnection) postUrl.openConnection();
con.setDoOutput(true);
con.setRequestMethod("POST");
con.setRequestProperty("Host", "app.alachisoft.com");
con.setRequestProperty("Content-type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(strFormData.length()));
DataOutputStream connOut = new DataOutputStream(con.getOutputStream());
connOut.writeBytes(strFormData);
connOut.flush();
connOut.close();
int rc = con.getResponseCode();
String strMsg = super.getStringTableProps().getProperty(String.valueOf(rc + 1000));
if (rc >= 400 && rc <= 599) {
deactivationConsole.append("Request/Server Error: Status Code = ");
deactivationConsole.
append(String.valueOf(rc)).
append("\r\n").
append("Message: ").
append(strMsg).
append("\r\n");
System.out.println(deactivationConsole.toString());
return;
}
deactivationConsole.
append(String.valueOf(rc)).
append("\r\n").
append("Message: ").
append(strMsg).
append("\r\n\r\n").
append("Requesting validation...\r\n").
append("Waiting for status...\r\n");
System.out.println(deactivationConsole.toString());
DataInputStream connIn = new DataInputStream(con.getInputStream());
//BufferedReader d = new BufferedReader(new InputStreamReader(con.getInputStream()));
BufferedReader d = new BufferedReader(new InputStreamReader(connIn));
//System.err.println("Waiting for response!");
while (!d.ready()) {
// System.out.println(d.ready());
}
//System.err.println("Got the response!");
char tmpChar;
String serverResp = new String("");
char noChar = (char) -1;
do {
tmpChar = (char) d.read();
serverResp += tmpChar;
}
while (tmpChar != noChar);
//AfxMessageBox(serverResp);//just for debugging
//String serverResp = new String("The file is not writable.The file is not writable.dVQ00JemN9dg8EDgpyfGN+dXZ2fn58f3R/fXwha2hpY= Thank you for registering your copy of NCache. ?");
StringRef extractedMessage = new StringRef();
XmlUtil.ExtractFromXML("", serverResp, extractedMessage);
//System.err.println("Stage 2");
if (serverResp.indexOf("") != -1) {
deactivationConsole = new StringBuilder();
deactivationConsole.append("\r\n").append(extractedMessage.strData);
System.out.println(deactivationConsole.toString());
//AfxMessageBox(extractedMessage, MB_OK|MB_ICONERROR);
return;
}
//System.err.println("Stage 3");
StringRef szDeactKey = new StringRef();
//System.err.println("Extract from XML");
XmlUtil.ExtractFromXML("", serverResp, szDeactKey);
//System.err.println("Stage 3:1 Verifying Auth Key");
AppUtil.VerifyResult result = super.getAppUtil().VerifAuthKey(szDeactKey.strData);
if (result != AppUtil.VerifyResult.Success) {
//System.err.println("Stage 3:1 Verifying Auth Verification Failed" + result);
System.out.println("Invalid activation key specified\r\n" + strMsg);
//AfxMessageBox(strMsg, MB_OK|MB_ICONERROR);
return;
}
//System.err.println("Stage 4");
super.getAppUtil().DeactKey(szDeactKey.strData);
System.out.println("\r\n" + extractedMessage.strData);
//System.err.println("Stage 5");
} catch (Exception e) {
System.out.println("Connection failed: " + e.getMessage());
//System.err.println("Stage F");
return;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy