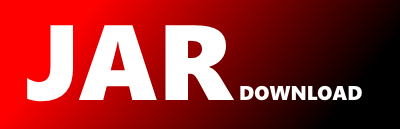
com.alachisoft.ncache.licensing.License Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* License.java
*
* Created on October 13, 2006, 9:18 AM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*/
package com.alachisoft.ncache.licensing;
import com.alachisoft.ncache.ncactivate.license.*;
import com.alachisoft.ncache.ncactivate.utils.AppUtil;
import com.alachisoft.ncache.ncactivate.utils.EnvironmentUtil;
import com.alachisoft.ncache.ncactivate.utils.RegUtil;
import com.alachisoft.ncache.ncactivate.utils.StringRef;
import com.alachisoft.ncache.runtime.exceptions.LicensingException;
import com.alachisoft.ncache.runtime.exceptions.LocalVerificationFailedException;
import com.alachisoft.ncache.runtime.util.RuntimeUtil;
import java.io.IOException;
import java.net.InetAddress;
import java.nio.charset.Charset;
import java.text.ParseException;
import java.util.*;
//import java.util.logging.Level;
//import java.util.logging.Logger;
//import sun.text.normalizer.UTF16;
/**
* @author Administrator
*/
public class License {
private static final String winStub = RegUtil.winStub;
private static final String linuxStub = RegUtil.linuxStub;
static public LicScheme LicenseScheme;
/**
* Creates a new instance of License
*/
public License() {
}
public static String[] getStubPaths() {
String systemFolder[] = null;
if (RuntimeUtil.getCurrentOS().equals(RuntimeUtil.OS.Windows)) {
//String home = System.getProperty("user.home");
//String drive = String.valueOf(home.charAt(0));
//String path = System.getProperty("java.library.path");
//systemFolder = getWinSystemDir("system32", path);
String sys32Folder = System.getenv("WINDIR") + "\\system32";
systemFolder = new String[]{sys32Folder};
//System.out.println(systemFolder[0]);
//systemFolder = new String[]{drive + ":\\WINDOWS\\system32\\stubnc.dll"};
for (int i = 0; i < systemFolder.length; i++) {
systemFolder[i] += "\\" + winStub;
}
} else {
systemFolder = new String[]
{
"//usr//lib//" + linuxStub,
"//usr//bin//" + linuxStub,
};
}
return systemFolder;
}
public static boolean isFree(int edid) {
return /*edid == 37 || edid == 39 || */edid == 19 || edid == 20 || edid == 29; //Clients, Developer and express editions are free, so its always licensed.
}
public static void isActivated(int version) throws LicensingException, LocalVerificationFailedException {
SystemTime pSysTime = new SystemTime();
String systemFolder[] = null;
RuntimeUtil.OS currentOS = RuntimeUtil.getCurrentOS();
try {
systemFolder = NCMisc.getStubPath();
} catch (SecurityException se) {
throw se;
} catch (Exception ex) {
throw new LicensingException("Invalid Authentication Code: Stage 006");
}
ByteRef data = NCMisc.ReadEvaluationData(systemFolder);
NCMisc.GetInstallTime(data.data, version, pSysTime);
if (pSysTime.wSecond == ActivationStatus.Deactivated.getOrdinal(ActivationStatus.Deactivated)) {
throw new LicensingException("Invalid Authentication Code: Stage 009");
}
//if windows is OS and edition is client then we check ncstub.dll as well for deactivation flag.
if (currentOS == RuntimeUtil.OS.Windows
&& (LicenseManager.getEdition().equals(LicenseManager.Edition.enterpriseClient) || LicenseManager.getEdition().equals(LicenseManager.Edition.profClient))) {
for (int i = 0; i < systemFolder.length; i++) {
String ncStubFolder = systemFolder[i].replace(winStub, "ncstub.bin");
if (NCFileUtil.FileExists(ncStubFolder)) {
data = NCFileUtil.ReadFile(systemFolder[i]);
if (data == null) {
throw new LicensingException("Invalid Authentication Code: Stage 007");
}
if (data.data == null) {
throw new LicensingException("Invalid Authentication Code: Stage 008");
}
NCMisc.GetInstallTime(data.data, version, pSysTime);
if (pSysTime.wSecond == ActivationStatus.Deactivated.getOrdinal(ActivationStatus.Deactivated)) {
throw new LicensingException("Invalid Authentication Code: Stage 009");
}
break;
}
}
}
}
public static void isActivated(int version, byte[] data) throws LicensingException {
SystemTime pSysTime = new SystemTime();
NCMisc.GetInstallTime(data, version, pSysTime);
if (pSysTime.wSecond == ActivationStatus.Deactivated.getOrdinal(ActivationStatus.Deactivated)) {
throw new LicensingException("Invalid Authentication Code: Stage 009");
}
}
public static void IsValid(String version) throws LicensingException, LocalVerificationFailedException {
ArrayList macs;
try {
macs = EnvironmentUtil.GetAdaptersInfo();
for (Iterator it = macs.iterator(); it.hasNext(); ) {
String mac = (String) it.next();
}
} catch (SecurityException se) {
throw se;
} catch (IOException ex) {
if (com.alachisoft.ncache.util.Trace.isOn()) {
com.alachisoft.ncache.util.Trace.out.println("License: isValid() " + ex.toString());
}
throw new LicensingException("License not verified due to following reason: " + ex.toString());
}
IsValid(version, NCCpuInfo.GetNumProcessors(), macs, NCCpuInfo.getPhysicalCoreCount(), NCCpuInfo.getLogicalCoreCount(), NCCpuInfo.getSocketCount());
}
public static Integer Decode(byte[] data) {
ByteRef refBytes = new ByteRef();
refBytes.data = data;
NCCrypto.EncryptDecryptBytes(refBytes, data.length, 0);
String cpuCountString = new String(refBytes.data, Charset.forName("utf-8"));
return Integer.decode(cpuCountString);
}
public static String convertToString(byte[] data) {
ByteRef refBytes = new ByteRef();
refBytes.data = data;
NCCrypto.EncryptDecryptBytes(refBytes, data.length, 0);
return new String(refBytes.data, Charset.forName("utf-8"));
}
public static void IsValid(String version, byte[] data1, byte[] data2, byte[] data3, byte[] data4, byte[] data5) throws LicensingException {
ArrayList macs;
int numCPU = 0;
// NCCrypto.EncryptDecryptBytes(refBytes, data1.length, 0);
// String cpuCountString = new String(refBytes.data, Charset.forName("utf-8"));
// numCPU = Integer.decode(cpuCountString);
numCPU = Decode(data1);
ByteRef refBytes = new ByteRef();
refBytes.data = data2;
NCCrypto.EncryptDecryptBytes(refBytes, data2.length, 0);
String macAddressString = new String(refBytes.data, Charset.forName("utf-8"));//new String(refBytes.data, Charset.forName("utf-8"));
if (macAddressString != null) {
String[] parsedmacAddress = macAddressString.split(";");
macs = new ArrayList();
for (int i = 0; i < parsedmacAddress.length; i++) {
macs.add(parsedmacAddress[i]);
}
}
try {
macs = EnvironmentUtil.GetAdaptersInfo();
} catch (SecurityException se) {
throw se;
} catch (IOException ex) {
if (com.alachisoft.ncache.util.Trace.isOn()) {
com.alachisoft.ncache.util.Trace.out.println("License: isValid() " + ex.toString());
}
throw new LicensingException("License not verified due to following reason: " + ex.toString());
}
int physicalCoreCount = Decode(data3);
int logicalCoreCount = Decode(data4);
int socketCount = Decode(data5);
IsValid(version, numCPU, macs, physicalCoreCount, logicalCoreCount, socketCount);
}
private static ExpirationInfo IsValid(String version, int cpuCount, ArrayList macs, int physicalCoreCount, int logicalCoreCount, int socketCount) throws LicensingException {
ExpirationInfo ei = new ExpirationInfo();
// Sample Activation Code
// dVQ0obYXV6dX9zHg1gfGN5ZXZ3eXl4fyx+J352bH9+c=
// cpu-SHOAIB-1-0.9-000102d0c916---
//[lic-type]:[Comp-name]:[logic-cpu]:[phy-cpu]:[ver]:[edtid]:[expiry-date]:[grace-period]:[activation-id]:[mac1]:[mac2]:[mac3]:[mac4]
//Trace.debug("License.IsValid()", "AuthCode: " + authCode);
try {
StringRef ac = new StringRef();
String authCode = "";
NCMisc.ReadActivationCode(ac);
if (!ac.strData.trim().equals("")) {
authCode = ac.strData;
}
String[] authTokens = authCode.split(":");
//
String scheme = authTokens[0].toLowerCase();
if (scheme.compareTo("cpu") == 0) {
LicenseScheme = LicScheme.PerProcessor;
} else if (scheme.compareTo("node") == 0) {
LicenseScheme = LicScheme.PerNode;
} else {
throw new LicensingException(" Invalid Authentication Code: Stage 001 ");
}
String machineName = InetAddress.getLocalHost().getHostName().toLowerCase(); // name
if (machineName.compareTo(authTokens[1].toLowerCase()) != 0) {
throw new LicensingException(" Invalid Authentication Code: Stage 002 ");
}
//TODO: Muneeb logic changed
// // Check the number of processor only for a per processor license
// if (LicScheme != Type.PerNode)
// {
// if (cpuCount > Integer.parseInt(parts[2]))
// {
// throw new LicensingException(" Invalid Authentication Code: Stage 003");
// }
// }
// if (LicenseScheme.equals(LicScheme.PerProcessor))
// {
// if (cpuCount > Integer.parseInt(authTokens[2]))
// {
// throw new LicensingException(" Invalid Authentication Code: Stage 003");
// }
// }
// else if (LicenseScheme.equals(LicScheme.PerCore))
// {
// if (physicalCoreCount > Integer.parseInt(authTokens[2]) || logicalCoreCount > Integer.parseInt(authTokens[3]))
// {
// throw new LicensingException(" Invalid Authentication Code: Stage 003");
// }
// }
// else
// {
// throw new LicensingException(" Invalid Authentication Code: Stage 003");
// }
if (!authTokens[5].contains(version)) {
throw new LicensingException(" Invalid Authentication Code: Stage 004");
}
// if (LicScheme != Type.PerNode)
// {
//
// //[2] Matching Logical cpu's
//// if (licenseInfo.Cores > Convert.ToInt32(parts[2])) //found cores are more then what NC is licensed for
//// throw new LicensingException("Invalid Authentication Code--Stage03");
//
// //[3] Matching Physical processors cpu's
// //Java consider Logical cores as Physical so need to compare them as Physical cores
// if (EnvironmentUtil.GetProcessorsCount() > Integer.parseInt(parts[2])) ////found physical processors are more then what NC is licensed for
// throw new LicensingException("Invalid Authentication Code--Stage04 Processor Count: " + Integer.toString(EnvironmentUtil.GetProcessorsCount()));
// }
//string version =
// Assembly.GetExecutingAssembly().GetName().Version.Major.ToString() + "." +
// Assembly.GetExecutingAssembly().GetName().Version.Minor.ToString();
//Now at three we are getting Physical CPU count. so making it 4. and adding one to rest [Asif Imam]
//if(version.compareTo(parts[4].toLowerCase()) != 0)
//throw new LicensingException("Invalid Authentication Code");
//usama@31dec2014 in new licensing 6,7,8 will be expiration date, grace periods and activation-id respectively
boolean hasMac1 = macs.contains(authTokens[9].toLowerCase());
boolean hasMac2 = macs.contains(authTokens[10].toLowerCase());
boolean hasMac3 = macs.contains(authTokens[11].toLowerCase());
boolean hasMac4 = macs.contains(authTokens[12].toLowerCase());
if (!hasMac1 && !hasMac2 && !hasMac3 && !hasMac4) {
throw new LicensingException("Invalid Authentication Code: Stage 005");
}
if (!authTokens[6].equals("null")) {
try {
Calendar expiration = LicenseHelper.getUTCDate(authTokens[6]);
int gracePeriod = Integer.parseInt(authTokens[7]);
ei.setExpirationDate(expiration);
ei.setGracePeriod(gracePeriod);
if (LicenseHelper.getUTCDate().after(ei.getHardExpirationDate()))
throw new LicensingException("License for NCache has been expired.--Stage11");
// if(LicenseManager.getIsService() && !LicenseMonitor.VerifyLicenseMonitoring())
// throw new LicensingException("Invalid Authentication Code: Stage 012");
} catch (ParseException ex) {
throw new LicensingException("Invalid Authentication Code: Stage 010");
}
} else {
//perpatual license
ei.setIsPerpetual(true);
}
} catch (LicensingException le) {
//le.printStackTrace();
throw new LicensingException("License not verified due to following reason: " + le.toString());
} catch (java.util.MissingResourceException mre) {
throw new LicensingException("License not verified due to following reason: " + mre.toString());
} catch (SecurityException se) {
throw se;
} catch (Exception ex) {
if (com.alachisoft.ncache.util.Trace.isOn()) {
com.alachisoft.ncache.util.Trace.out.println("License: isValid() " + ex.toString());
}
throw new LicensingException("License not verified due to following reason: " + ex.toString());
}
return ei;
}
public static ExpirationInfo GetExpirationInfo() {
ArrayList macs = null;
ExpirationInfo info = new ExpirationInfo(); //Expired by default
try {
macs = EnvironmentUtil.GetAdaptersInfo();
for (Iterator it = macs.iterator(); it.hasNext(); ) {
String mac = (String) it.next();
}
info = IsValid(AppUtil.getVersion(), NCCpuInfo.GetNumProcessors(), macs, NCCpuInfo.getPhysicalCoreCount(), NCCpuInfo.getLogicalCoreCount(), NCCpuInfo.getSocketCount());
} catch (Exception ex) {
}
return info;
}
private static String[] getWinSystemDir(String delim, String prop) {
StringTokenizer tokanizer = new StringTokenizer(prop);
Vector v = new Vector();
String token = "";
while (tokanizer.hasMoreTokens()) {
token = tokanizer.nextToken(";");
if (token.indexOf(delim) != -1) {
if (!v.contains(token)) {
v.add(token);
}
}
}
return (String[]) v.toArray(new String[0]);
}
public static void IsValid() throws LicensingException {
// Sample Activation Code
// dVQ0obYXV6dX9zHg1gfGN5ZXZ3eXl4fyx+J352bH9+c=
// cpu-SHOAIB-1-0.9-000102d0c916---
//[lic-type]:[Comp-name]:[logic-cpu]:[phy-cpu]:[ver]:[edtid][mac1]:[mac2]:[mac3]:[mac4]
//Trace.debug("License.IsValid()", "AuthCode: " + authCode);
try {
StringRef ac = new StringRef();
NCMisc.ReadActivationCode(ac);
String authCode = ac.strData;
//Trace.debug("License.IsValid()", "DecodedAuthCode: " + authCode);
//System.out.println("Auth-code:" + authCode);
String[] parts = ac.strData.split(":");
// for(int i = 0; i < parts.length; i++)
// {
// System.out.println("Index: " + Integer.toString(i) + "Data: " + parts[i]);
// }
String scheme = parts[0].toLowerCase();
if (scheme.compareTo("cpu") == 0) {
LicenseScheme = LicScheme.PerProcessor;
} else {
if (scheme.compareTo("node") == 0) {
LicenseScheme = LicScheme.PerNode;
} else {
throw new LicensingException(" Invalid Authentication Code: Stage 001 ");
}
}
String machineName = InetAddress.getLocalHost().getHostName().toLowerCase(); // name
if (machineName.compareTo(parts[1].toLowerCase()) != 0) {
throw new LicensingException(" Invalid Authentication Code: Stage 002 ");
}
// Check the number of processor only for a per processor license
// if (LicenseScheme != LicScheme.PerNode)
// {
// if (NCCpuInfo.GetNumProcessors() > Integer.parseInt(parts[2]))
// {
// throw new LicensingException(" Invalid Authentication Code: Stage 003");
// }
// }
//
// if (!parts[5].contains(version))
// {
// throw new LicensingException(" Invalid Authentication Code: Stage 004");
// }
// if (LicScheme != Type.PerNode)
// {
//
// //[2] Matching Logical cpu's
//// if (licenseInfo.Cores > Convert.ToInt32(parts[2])) //found cores are more then what NC is licensed for
//// throw new LicensingException("Invalid Authentication Code--Stage03");
//
// //[3] Matching Physical processors cpu's
// //Java consider Logical cores as Physical so need to compare them as Physical cores
// if (EnvironmentUtil.GetProcessorsCount() > Integer.parseInt(parts[2])) ////found physical processors are more then what NC is licensed for
// throw new LicensingException("Invalid Authentication Code--Stage04 Processor Count: " + Integer.toString(EnvironmentUtil.GetProcessorsCount()));
// }
//string version =
// Assembly.GetExecutingAssembly().GetName().Version.Major.ToString() + "." +
// Assembly.GetExecutingAssembly().GetName().Version.Minor.ToString();
//Now at three we are getting Physical CPU count. so making it 4. and adding one to rest [Asif Imam]
//if(version.compareTo(parts[4].toLowerCase()) != 0)
//throw new LicensingException("Invalid Authentication Code");
ArrayList macs = EnvironmentUtil.GetAdaptersInfo();
boolean hasMac1 = macs.contains(parts[9].toLowerCase());
boolean hasMac2 = macs.contains(parts[10].toLowerCase());
boolean hasMac3 = macs.contains(parts[11].toLowerCase());
boolean hasMac4 = macs.contains(parts[12].toLowerCase());
if (!hasMac1 && !hasMac2 && !hasMac3 && !hasMac4) {
throw new LicensingException("Invalid Authentication Code: Stage 005");
}
} catch (LicensingException le) {
//le.printStackTrace();
throw new LicensingException("License not verified due to following reason: " + le.toString());
} catch (java.util.MissingResourceException mre) {
throw new LicensingException("License not verified due to following reason: " + mre.toString());
} catch (Exception ex) {
if (com.alachisoft.ncache.util.Trace.isOn()) {
com.alachisoft.ncache.util.Trace.out.println("License: isValid() " + ex.toString());
}
throw new LicensingException("License not verified due to following reason: " + ex.toString());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy