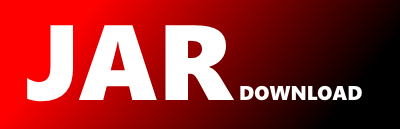
com.alachisoft.ncache.licensing.LicenseInfo Maven / Gradle / Ivy
Show all versions of nc-activate Show documentation
package com.alachisoft.ncache.licensing;
/**
* ***********************************************************************
* Author: Hasan Khan Date created: 06-05-2008
*
* Modified By: Last modified by: Date modified:
* /************************************************************************
*/
//C# TO JAVA CONVERTER TODO TASK: There is no preprocessor in Java:
//#if NCPEX
//#else
//#endif
import com.alachisoft.ncache.ncactivate.appdata.MachineInfo;
import java.util.ArrayList;
import java.util.Collections;
/**
* Encapsulates all the information necessary for license validation
*/
public class LicenseInfo implements java.io.Serializable {
static LicenseInfo licenseInfo;
public ArrayList macsInfo;
private int cores, processors;
private String version, mac1, mac2, mac3, mac4, machineName;
public LicenseInfo() {
version = "";
mac1 = "";
mac2 = "";
mac3 = "";
mac4 = "";
machineName = "";
}
public static LicenseInfo deserialize(String data) {
String[] tokens = data.split("[,]", -1);
LicenseInfo info = new LicenseInfo();
info.setCores(Integer.parseInt(tokens[0]));
info.setProcessors(Integer.parseInt(tokens[1]));
info.setVersion(tokens[2]);
info.setMac1(tokens[3]);
info.setMac2(tokens[4]);
info.setMac3(tokens[5]);
info.setMac4(tokens[6]);
info.setMachineName(tokens[7]);
return info;
}
public static LicenseInfo getLicenseInfo() {
MachineInfo machineInfo = new MachineInfo();
if (licenseInfo == null) {
licenseInfo = new LicenseInfo();
licenseInfo.setMachineName(machineInfo.ComputerName);
licenseInfo.setCores(machineInfo.NumCpus);
//licenseInfo.setProcessors(MiscUtil.getNumProcessors());
//TODO : in Dotnet Version we get from Assembly information
licenseInfo.setVersion("5.1");
ArrayList macs = machineInfo.getMacs();
licenseInfo.setMacsInfo(macs);
if (macs.size() > 0) {
licenseInfo.setMac1(((String) macs.get(0)).toLowerCase());
if (macs.size() > 1) {
licenseInfo.setMac2(((String) macs.get(1)).toLowerCase());
if (macs.size() > 2) {
licenseInfo.setMac3(((String) macs.get(2)).toLowerCase());
if (macs.size() > 3) {
licenseInfo.setMac4(((String) macs.get(3)).toLowerCase());
}
}
}
}
}
return licenseInfo;
}
public ArrayList getMacsInfo() {
return macsInfo;
}
public String getStringMacInfo()
{
ArrayList macList = new ArrayList<>();
macList.add(getMac1());
macList.add(getMac2());
macList.add(getMac3());
macList.add(getMac4());
Collections.sort(macList);
return macList.get(0) + ":" + macList.get(1) + ":" + macList.get(2) + ":" + macList.get(3);
}
public void setMacsInfo(ArrayList macsInfo) {
this.macsInfo = macsInfo;
}
public final int getCores() {
return cores;
}
public final void setCores(int value) {
cores = value;
}
public final int getProcessors() {
return processors;
}
public final void setProcessors(int value) {
processors = value;
}
public final String getVersion() {
return version;
}
public final void setVersion(String value) {
version = value;
}
public final String getMac1() {
return mac1;
}
public final void setMac1(String value) {
mac1 = value;
}
public final String getMac2() {
return mac2;
}
public final void setMac2(String value) {
mac2 = value;
}
public final String getMac3() {
return mac3;
}
public final void setMac3(String value) {
mac3 = value;
}
public final String getMac4() {
return mac4;
}
public final void setMac4(String value) {
mac4 = value;
}
public final String getMachineName() {
return machineName;
}
public final void setMachineName(String value) {
machineName = value;
}
public final String serialize() {
StringBuilder data = new StringBuilder();
data.append(String.format("%1$s,%2$s,%3$s,%4$s,%5$s,%6$s,%7$s,%8$s", getCores(), getProcessors(), getVersion(), getMac1(), getMac2(), getMac3(), getMac4(), getMachineName()));
return data.toString();
}
@Override
public boolean equals(Object obj) {
LicenseInfo info = (LicenseInfo) obj;
if (!tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac1()) && this.getMac1().equals(info.getMac1())
|| !tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac1()) && this.getMac1().equals(info.getMac2())
|| !tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac1()) && this.getMac1().equals(info.getMac3())
|| !tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac1()) && this.getMac1().equals(info.getMac4())
|| !tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac2()) && this.getMac2().equals(info.getMac2())
|| !tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac2()) && this.getMac2().equals(info.getMac3())
|| !tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac2()) && this.getMac2().equals(info.getMac4())
|| !tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac3()) && this.getMac3().equals(info.getMac3())
|| !tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac3()) && this.getMac3().equals(info.getMac4())
|| !tangible.DotNetToJavaStringHelper.isNullOrEmpty(this.getMac4()) && this.getMac4().equals(info.getMac4())) {
return true;
}
if (!this.getMac1().equals("") && this.getMac1() != null && this.getMac1().equals(info.getMac1()) || !this.getMac1().equals("") && this.getMac1() != null
&& this.getMac1().equals(info.getMac2()) || !this.getMac1().equals("") && this.getMac1() != null && this.getMac1().equals(info.getMac3())
|| !this.getMac1().equals("") && this.getMac1() != null && this.getMac1().equals(info.getMac4()) || !this.getMac2().equals("") && this.getMac2() != null
&& this.getMac2().equals(info.getMac2()) || !this.getMac2().equals("") && this.getMac2() != null && this.getMac2().equals(info.getMac3())
|| !this.getMac2().equals("") && this.getMac2() != null && this.getMac2().equals(info.getMac4()) || !this.getMac3().equals("") && this.getMac3() != null
&& this.getMac3().equals(info.getMac3()) || !this.getMac3().equals("") && this.getMac3() != null && this.getMac3().equals(info.getMac4())
|| !this.getMac4().equals("") && this.getMac4() != null && this.getMac4().equals(info.getMac4())) {
return true;
} else {
return false;
}
}
public final boolean Update(LicenseInfo item) {
boolean modified = false;
if (item.getCores() > this.getCores()) {
this.setCores(item.getCores());
modified = true;
}
if (item.getProcessors() > this.getProcessors()) {
this.setProcessors(item.getProcessors());
modified = true;
}
if (!this.getVersion().equals(item.getVersion())) {
this.setVersion(item.getVersion());
modified = true;
}
if (!this.getMachineName().equals(item.getMachineName())) {
this.setMachineName(item.getMachineName());
modified = true;
}
return modified;
}
}