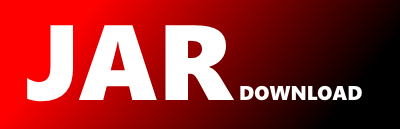
com.alachisoft.ncache.ncactivate.appdata.ProductInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* ProductInfo.java
*
* Created on October 7, 2006, 3:40 PM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*/
package com.alachisoft.ncache.ncactivate.appdata;
import com.alachisoft.ncache.licensing.config.InfoType;
import com.alachisoft.ncache.ncactivate.crypto.Crypto;
import com.alachisoft.ncache.ncactivate.utils.AppUtil;
import com.alachisoft.ncache.ncactivate.utils.EnvironmentUtil;
import com.alachisoft.ncache.ncactivate.utils.RegKeys;
import com.alachisoft.ncache.ncactivate.utils.RegUtil;
import java.util.Locale;
/**
* @author Administrator
*/
public class ProductInfo {
public String ProductName;
public String Edition;
public String Version;
public String LicenseKey;
public String ActId;
public String[] LicSchemes;
public String DeactivateCode;
public int VersionId;
public boolean Reactivation;
/**
* Creates a new instance of MachineInfo
*/
public ProductInfo() {
}
public int Load() {
ProductName = new String("NCache");
String installCode = new String();
installCode = RegUtil.getKey(InfoType.PRODUCT, RegKeys.InstallCode);
if ("".equals(installCode)) {
return -1;
}
installCode = Crypto.Decode(installCode);
String[] tokens = installCode.split(":");
Edition = tokens[0];
String supportedSchemes = tokens[1];//installCode.Tokenize(":"), curPos);
Version = tokens[2];//installCode.Tokenize(":"), curPos);
LicenseKey = RegUtil.getKey(InfoType.LICENSE, RegKeys.LicenseKey);
String authCode = RegUtil.getKey(InfoType.LICENSE, RegKeys.AuthCode);
ActId = AppUtil.getActivationID(authCode);
if ("".equals(ActId))
ActId = EnvironmentUtil.NewGUID();
LicSchemes = supportedSchemes.split(",");
DeactivateCode = RegUtil.getKey(InfoType.LICENSE, RegKeys.DeactCode);
VersionId = AppUtil.getEditionID();
return 0;
}
public void Save() {
RegUtil.setKey(InfoType.LICENSE, RegKeys.LicenseKey, LicenseKey);
}
public String ToXml() {
String xml = new String("");
xml += "";
xml += ProductName;
xml += " ";
xml += "";
xml += Edition;
xml += " ";
xml += "";
xml += Version;
xml += " ";
xml += "";
xml += LicenseKey;
xml += " ";
xml += "";
xml += ActId;
xml += " ";
xml += "";
xml += DeactivateCode;
xml += " ";
xml += "";
xml += Reactivation ? "true" : "false";
xml += "";
xml += " ";
return xml;
}
public String ToLinearText() {
String reactivationString = Reactivation ? "true" : "false";
String text = String.format(Locale.US, "%1$s\t%2$s\t%3$s\t%4$s\t%5$s\t%6$s\t%7$s", ProductName, Edition, Version, LicenseKey, ActId, DeactivateCode, reactivationString);
return text;
}
boolean IsLicSchemeSupported(String licScheme) {
int licCount = LicSchemes.length;
for (int i = 0; i < licCount; i++) {
String scheme = LicSchemes[i];
if (scheme.compareToIgnoreCase(licScheme) == 0) {
return true;
}
}
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy