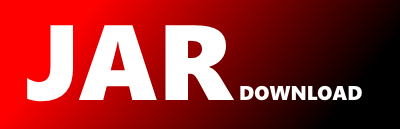
com.alachisoft.ncache.ncactivate.cmdline.LicenseTool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.ncactivate.cmdline;
import com.alachisoft.ncache.common.commandline.ArgumentAttributeAnnotation;
import com.alachisoft.ncache.common.commandline.CommandLineArgs;
import com.alachisoft.ncache.common.commandline.CommandLineArgumentParser;
import com.alachisoft.ncache.ncactivate.cmdline.exceptions.CorruptInstallationException;
import com.alachisoft.ncache.ncactivate.cmdline.exceptions.InvalidKeyException;
import com.alachisoft.ncache.ncactivate.utils.AppUtil;
import java.util.Properties;
/**
* @author Muneeb_Shahid
*/
public abstract class LicenseTool {
private static final String BUNDLE_NAME = "com/alachisoft/ncache/ncactivate/utils/stringtable.properties";
private java.util.Properties stringTableProps;
private AppUtil appUtil;
private String[] args;
private CommandLineArgs cmdArgs;
public LicenseTool() throws CorruptInstallationException {
appUtil = new AppUtil();
stringTableProps = this.appUtil.loadResourceBundle(BUNDLE_NAME);
VerifyInstallation();
}
public Properties getStringTableProps() {
return stringTableProps;
}
public AppUtil getAppUtil() {
return appUtil;
}
public CommandLineArgs getCmdArgs() {
return cmdArgs;
}
public final void VerifyInstallation() throws CorruptInstallationException {
if (appUtil.getActivationData().Load() < 0) {
throw new CorruptInstallationException("The software does not seem to be properly installed.");
}
}
protected boolean intialize(String[] args, CommandLineArgs cmdArgs) {
this.args = args;
this.cmdArgs = cmdArgs;
return this.Parse();
}
protected final boolean ValidateEvalKey(String key) throws InvalidKeyException {
return appUtil.ValidateEvalKey(key);
}
protected boolean checkRequiredParams(java.util.HashMap requiredParams) {
StringBuilder missingArgsBuilder = new StringBuilder();
for (java.util.Map.Entry param : requiredParams.entrySet()) {
if (isNullOrEmpty(param.getValue())) {
missingArgsBuilder.append(param.getKey()).append(", ");
}
}
String missingArgs = missingArgsBuilder.toString().trim();
if (missingArgs.length() > 0) {
System.err.println("Following arguments are required ".concat(missingArgs.substring(0, missingArgs.lastIndexOf(","))).concat("."));
System.err.println("Use -h --help to view help.");
return false;
}
if (!AppUtil.GetReactivate() && getAppUtil().getProductInfo().Reactivation) {
System.out.println("Machine is already activated for enough cores, reactivation not allowed");
System.exit(0);
}
return true;
}
public abstract void Run(String[] args);
protected abstract void Run();
private boolean Parse() {
if (args != null && cmdArgs != null) {
try {
CommandLineArgumentParser.Parse(cmdArgs, args);
return true;
} catch (IllegalArgumentException iLE) {
String defaultValue = null;
try {
defaultValue = cmdArgs.getClass().getMethod("getHelp").getAnnotation(ArgumentAttributeAnnotation.class).fullNotation();
} catch (NoSuchMethodException nE) {
}
System.err.println(iLE.getMessage() + ((defaultValue == null) ? "" : "\nUse \"" + defaultValue + "\" to view help."));
} catch (Exception e) {
System.err.println(e.getMessage());
}
}
return false;
}
protected boolean printUsage() {
if (this.cmdArgs.getUsage()) {
this.cmdArgs.printUsage();
return true;
}
return false;
}
protected boolean pringLogo() {
if (this.cmdArgs.getLogo()) {
this.cmdArgs.printLogo();
return true;
}
return false;
}
public boolean isNullOrEmpty(String param) {
return param == null || param.isEmpty();
}
public String getMachineInfoCode() {
return appUtil.generateMachineInfoCode();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy