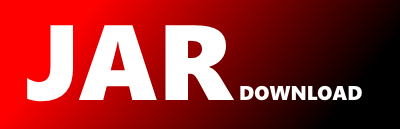
com.alachisoft.ncache.ncactivate.license.CloudDll Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.alachisoft.ncache.ncactivate.license;
import com.alachisoft.ncache.runtime.exceptions.LicensingException;
import com.alachisoft.ncache.runtime.util.RuntimeUtil;
import java.util.MissingResourceException;
import java.util.StringTokenizer;
import java.util.Vector;
/**
* Creates and reads cloud license values
*
* @author moiz_rauf
*/
public class CloudDll {
public static final String winStub = "cld_vc.dll";
public static final String linuxStub = ".cld_vc.so";
public static int InitializeStub(int version, byte[] data) throws LicensingException, Exception {
// ActivationStatus activationStatus = ActivationStatus.Eval;
String[] systemFolder = null;
RuntimeUtil.OS operatingSystem = RuntimeUtil.getCurrentOS();
//
try {
if (operatingSystem == RuntimeUtil.OS.Windows) {
// String home = System.getProperty("user.home");
// String drive = String.valueOf(home.charAt(0));
//
// String path = System.getProperty("java.library.path");
// systemFolder = getWinSystemDir("system32", path);
String sys32Folder = System.getenv("WINDIR") + "\\system32";
systemFolder = new String[]{sys32Folder};
//systemFolder = new String[]{drive + ":\\WINDOWS\\system32\\stubnc.dll"};
for (int i = 0; i < systemFolder.length; i++) {
systemFolder[i] += "\\" + winStub;
}
} else {
systemFolder = new String[]
{
"//usr//lib//" + linuxStub,
"//usr//bin//" + linuxStub
};
}
} catch (Exception ex) {
ex.printStackTrace();
}//
long dwSize = 0;
boolean fileExists = false;
for (int i = 0; i < systemFolder.length && !fileExists; i++) {
fileExists = NCFileUtil.FileExists(systemFolder[i]);
if (fileExists) {
throw new Exception("Already Licensed...");
} else {
//
try {
boolean result = true;
for (i = 0; i < systemFolder.length && result; i++) {
result = NCFileUtil.WriteFile(systemFolder[i], data, data.length);
}
if (result == true) {
return 0;
} else {
return -1;
}
} catch (MissingResourceException mre) {
mre.printStackTrace();
}//
}
if (data == null) {
return -1;
}
}
int ret = -1;
return ret;
}
private static String[] getWinSystemDir(String delim, String prop) {
StringTokenizer tokanizer = new StringTokenizer(prop);
Vector v = new Vector();
String token = "";
while (tokanizer.hasMoreTokens()) {
token = tokanizer.nextToken(";");
if (token.indexOf(delim) != -1) {
if (!v.contains(token)) {
v.add(token);
}
}
}
return (String[]) v.toArray(new String[0]);
}
public static byte[] readStub() throws Exception {
byte[] data = null;
String[] systemFolder = null;
RuntimeUtil.OS operatingSystem = RuntimeUtil.getCurrentOS();
boolean isWindows = false;
//
try {
if (operatingSystem == RuntimeUtil.OS.Windows) {
// String home = System.getProperty("user.home");
// String drive = String.valueOf(home.charAt(0));
//
// String path = System.getProperty("java.library.path");
// systemFolder = getWinSystemDir("system32", path);
String sys32Folder = System.getenv("WINDIR") + "\\system32";
systemFolder = new String[]{sys32Folder};
//systemFolder = new String[]{drive + ":\\WINDOWS\\system32\\stubnc.dll"};
for (int i = 0; i < systemFolder.length; i++) {
systemFolder[i] += "\\" + winStub;
//systemFolder[i] += "\\";
}
isWindows = true;
} else {
systemFolder = new String[]{
// "//usr//lib//" + linuxStub,
// "//usr//bin//" + linuxStub
"//usr//lib//",
"//usr//bin//"
};
}
} catch (Exception ex) {
}//
for (int i = 0; i < systemFolder.length; i++) {
String ncStubFolder = "";
if (isWindows) {
ncStubFolder = systemFolder[i].replace(winStub, "cld_vc.dll");
} else {
ncStubFolder = systemFolder[i].replace(linuxStub, ".cld_vc.so");
}
if (NCFileUtil.FileExists(ncStubFolder)) {
if (isWindows) {
data = NCFileUtil.ReadFile(systemFolder[i]).data;
} else {
data = NCFileUtil.ReadFile(linuxStub).data;
}
} else {
throw new Exception("Not Licensed for cloud...");
}
}
return data;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy