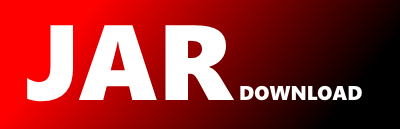
com.alachisoft.ncache.ncactivate.license.NCCpuInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
/*
* NCCpuInfo.java
*
* Created on October 2, 2006, 9:29 AM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*/
package com.alachisoft.ncache.ncactivate.license;
import com.alachisoft.ncache.runtime.exceptions.LicensingException;
import com.alachisoft.ncache.runtime.exceptions.LocalVerificationFailedException;
import com.alachisoft.ncache.runtime.util.RuntimeUtil;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
/**
* @author Administrator
*/
public class NCCpuInfo {
private final static int coresPerLicense = 4;
private static int _licenseCount = -1;
private static int _physCPU = -1;
private static int _logiCPU = -1;
private static int _socketCount = -1;
private static boolean _loaded = false;
private static String _linuxPhysicalCPUCommand = "grep \"cpu cores\" /proc/cpuinfo | uniq | sed -e 's/[^0-9]*//g'";
private static String _linuxLogicalCPUCommand = "grep \"processor\" /proc/cpuinfo | wc -l";
private static String _linuxSocketCountCPUCommand = "grep \"physical id\" /proc/cpuinfo | sort | uniq | wc -l";
private static int _maxCoresPerLicense = 4;
private static int _maxLicense = 16;
/**
* Creates a new instance of NCCpuInfo
*/
public NCCpuInfo() {
}
public static int PresentCores() {
return _physCPU;
}
public static int PresentLicences() {
return _socketCount;
}
public static int calculateLicenseCount(int existingCores) //function to calculate licenses.
{
int physicalCpus = 0;
if (existingCores <= 8)
return 2;
while (true) {
if (existingCores > physicalCpus * _maxCoresPerLicense && existingCores <= (physicalCpus + 1) * _maxCoresPerLicense) {
if (physicalCpus == 0)
physicalCpus = 1;
else
physicalCpus = physicalCpus + 1;
break;
} else
physicalCpus = physicalCpus + 1;
}
return physicalCpus;
}
public static int getLicenseCount() throws LicensingException, LocalVerificationFailedException {
// int licenseCount = 0;
// int physicalCores = getPhysicalCoreCount();
// int logicalCores = getLogicalCoreCount();
//
// int licenseCountForPhysicalCores = physicalCores / coresPerLicense;
// if (physicalCores % coresPerLicense != 0)
// {
// licenseCountForPhysicalCores++;
// }
//
// int licenseCountForLogicalCores = logicalCores / coresPerLicense;
// if (logicalCores % coresPerLicense != 0)
// {
// licenseCountForLogicalCores++;
// }
//
// //Muneeb: 1 License for 8 logical cores afterwards 1 License for 4 logical cores.
// if (licenseCountForLogicalCores > 1)
// {
// licenseCountForLogicalCores--;
// }
//
// licenseCount = licenseCountForLogicalCores + licenseCountForPhysicalCores;
// return licenseCount;
return getSocketCount();
}
public static int getPhysicalCoreCount() throws LicensingException, LocalVerificationFailedException {
if (!_loaded) {
loadInfo();
}
return _physCPU;
}
public static int getLogicalCoreCount() throws LicensingException, LocalVerificationFailedException {
if (!_loaded) {
loadInfo();
}
return _logiCPU;
}
public static int getSocketCount() throws LicensingException, LocalVerificationFailedException {
if (!_loaded) //mashal
{
loadInfo();
if (_physCPU <= 8)
_socketCount = 2;
else
_socketCount = calculateLicenseCount(_physCPU);
}
_socketCount = calculateLicenseCount(_physCPU);
return _socketCount;
}
private static String[][] GetCommands() {
String[][] cmds = null;
if (RuntimeUtil.getCurrentOS().equals(RuntimeUtil.OS.Linux)) {
cmds = new String[][]
{
new String[]
{
"/bin/sh", "-c", _linuxPhysicalCPUCommand
},
new String[]
{
"/bin/sh", "-c", _linuxLogicalCPUCommand
},
new String[]
{
"/bin/sh", "-c", _linuxSocketCountCPUCommand
},
};
} else if (RuntimeUtil.getCurrentOS().equals(RuntimeUtil.OS.Windows)) {
cmds = new String[][]
{
new String[]
{
"wmic", "cpu", "get"
},
};
}
return cmds;
}
private static String GetCommandOutput() throws IOException, LocalVerificationFailedException {
String[][] cmds = GetCommands();
StringBuilder outputText = new StringBuilder();
for (String[] cmd : cmds) {
Process p;
try {
p = Runtime.getRuntime().exec(cmd);
} catch (IOException iOE) {
throw new LocalVerificationFailedException("Failed to execute shell command. Contact local server.");
}
InputStream stdoutStream = new BufferedInputStream(p.getInputStream());
StringBuilder buffer = new StringBuilder();
for (; ; ) {
int c = stdoutStream.read();
if (c == -1) {
break;
}
buffer.append((char) c);
}
if (buffer.toString().equals("")) {
outputText.append(" ");
} else {
outputText.append(buffer.toString());
}
stdoutStream.close();
outputText.append(";");
}
return outputText.toString();
}
public static int GetNumProcessors() throws LicensingException, LocalVerificationFailedException {
if (!_loaded) {
loadInfo();
}
return getLicenseCount();
}
private static void ParseResult(String outString) throws LicensingException {
if (RuntimeUtil.getCurrentOS().equals(RuntimeUtil.OS.Windows)) {
String[] rows = outString.split(";")[0].split("\n");
if (rows.length >= 2) {
int numberOfCores = rows[0].indexOf("NumberOfCores");
int numberOfLogicalProcessors = rows[0].indexOf("NumberOfLogicalProcessors");
int numberOfSockets = rows[0].indexOf("SocketDesignation");
if (numberOfCores >= 0 && numberOfCores < rows[1].length()) {
try {
_physCPU = Character.getNumericValue(rows[1].charAt(numberOfCores));
} catch (Exception e) {
}
}
if (numberOfLogicalProcessors >= 0 && numberOfLogicalProcessors < rows[1].length()) {
try {
_logiCPU = Character.getNumericValue(rows[1].charAt(numberOfLogicalProcessors));
} catch (Exception e) {
}
}
if (numberOfSockets > 0) {
try {
for (int row = 1; row < rows.length - 1; row++) {
if (numberOfSockets < rows[row].length()) {
String socketSubString = rows[row].substring(numberOfSockets);
if (socketSubString.substring(0, 3).toUpperCase().equals("CPU") || socketSubString.substring(0, 3).toUpperCase().equals("PROC")) {
int currentSocketCount = 1;
String[] tokens = socketSubString.split(" ");
try {
currentSocketCount = Integer.parseInt(tokens[1]);
} catch (Exception e) {
}
if (_socketCount < currentSocketCount) {
_socketCount = currentSocketCount;
}
}
}
}
} catch (Exception e) {
//On any exception, considering it a VM
_socketCount = 2;
}
}
if (_socketCount < 1)
_socketCount = 2;
}
} else if (RuntimeUtil.getCurrentOS().equals(RuntimeUtil.OS.Linux)) {
String[] rows = outString.split(";");
int i = 0;
try {
_physCPU = Integer.parseInt(rows[i++].trim());
} catch (Exception e) {
}
try {
_logiCPU = Integer.parseInt(rows[i++].trim());
} catch (Exception e) {
}
try {
_socketCount = Integer.parseInt(rows[i++].trim());
} catch (Exception e) {
}
if (_physCPU == -1) {
if (_logiCPU == -1) {
throw new LicensingException("Invalid Authentication Code: Stage 1000");
}
_physCPU = _logiCPU;
}
if (_socketCount < 1)
_socketCount = 2; //considering it a VM
} else
_socketCount = 2; //If OS not detected, considering it a VM
}
public static void loadInfo() throws LicensingException, LocalVerificationFailedException {
Boolean loaded = false;
if (!_loaded) {
try {
String result;
if (RuntimeUtil.getCurrentOS() == RuntimeUtil.OS.Linux) {
result = GetCommandOutput();
ParseResult(result);
loaded = true;
} else if (RuntimeUtil.getCurrentOS() == RuntimeUtil.OS.Windows) {
result = GetCommandOutput();
ParseResult(result);
_loaded = loaded;
loaded = true;
}
} catch (IOException iOException) {
}
_loaded = loaded;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy