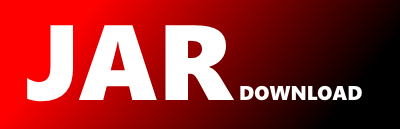
com.alachisoft.ncache.ncactivate.license.NCMisc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nc-activate Show documentation
Show all versions of nc-activate Show documentation
internal package of Alachisoft.
package com.alachisoft.ncache.ncactivate.license;
import com.alachisoft.ncache.licensing.config.InfoType;
import com.alachisoft.ncache.ncactivate.utils.AppUtil;
import com.alachisoft.ncache.ncactivate.utils.RegKeys;
import com.alachisoft.ncache.ncactivate.utils.RegUtil;
import com.alachisoft.ncache.ncactivate.utils.StringRef;
import com.alachisoft.ncache.runtime.exceptions.LicensingException;
import com.alachisoft.ncache.runtime.exceptions.LocalVerificationFailedException;
import com.alachisoft.ncache.runtime.util.RuntimeUtil;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.util.StringTokenizer;
import java.util.Vector;
/*
* NCMisc.java
*
* Created on September 28, 2006, 10:40 AM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*/
/**
* @author Administrator
*/
public class NCMisc {
public static final String winStub = RegUtil.winStub;
public static final String linuxStub = RegUtil.linuxStub;
public static int g_DataOffset = 0x1008;
/**
* Creates a new instance of NCMisc
*/
public NCMisc() {
}
// Fetches the current time and encrypts it together with other params into the data.
// version is the index into the array of version timestamps.
static void TimeStampData(byte[] dataPtr, long dwSize, int extensions, int period, int version) {
// Reach the offset in file where we have some data
int offset = 0;
offset += g_DataOffset;
offset += 16;
offset += (16 * version);
SystemTime pSysTime = new SystemTime();
WriteWordToByteArray(pSysTime.wYear, dataPtr, offset);
offset += 2;
WriteWordToByteArray(pSysTime.wMonth, dataPtr, offset);
offset += 2;
WriteWordToByteArray(pSysTime.wDay, dataPtr, offset);
offset += 2;
WriteWordToByteArray((short) extensions, dataPtr, offset);
offset += 2;
WriteWordToByteArray((short) 0, dataPtr, offset);
offset += 2;
WriteWordToByteArray((short) period, dataPtr, offset);
offset += 2;
WriteWordToByteArray((short) ActivationStatus.Eval.getOrdinal(ActivationStatus.Eval), dataPtr, offset);
offset += 2;
ByteRef dataPtr1 = new ByteRef();
dataPtr1.data = dataPtr;
NCCrypto.EncryptDecryptBytes(dataPtr1, (int) (dwSize - g_DataOffset), g_DataOffset);
}
static void TimeStampData(byte[] dataPtr, long dwSize, int extensions, int period, int version, ActivationStatus activationStatus) throws com.alachisoft.ncache.runtime.exceptions.LicensingException {
// Reach the offset in file where we have some data
int offset = 0;
offset += g_DataOffset;
offset += 16;
offset += (16 * version);
SystemTime pSysTime = new SystemTime();
WriteWordToByteArray(pSysTime.wYear, dataPtr, offset);
offset += 2;
WriteWordToByteArray(pSysTime.wMonth, dataPtr, offset);
offset += 2;
WriteWordToByteArray(pSysTime.wDay, dataPtr, offset);
offset += 2;
WriteWordToByteArray((short) extensions, dataPtr, offset);
offset += 2;
WriteWordToByteArray((short) 0, dataPtr, offset);
offset += 2;
WriteWordToByteArray((short) period, dataPtr, offset);
offset += 2;
if (pSysTime.wSecond != ActivationStatus.Activated.getOrdinal(ActivationStatus.Activated) || pSysTime.wSecond
== ActivationStatus.Deactivated.getOrdinal(ActivationStatus.Deactivated)) {
pSysTime.wSecond = (short) activationStatus.getOrdinal(activationStatus);
WriteWordToByteArray(pSysTime.wSecond, dataPtr, offset);
offset += 2;
} else {
WriteWordToByteArray(pSysTime.wSecond, dataPtr, offset);
offset += 2;
}
ByteRef dataPtr1 = new ByteRef();
dataPtr1.data = dataPtr;
NCCrypto.EncryptDecryptBytes(dataPtr1, (int) (dwSize - g_DataOffset), g_DataOffset);
}
static void UpdateActivationStatus(byte[] dataPtr, long dwSize, int version, ActivationStatus activationStatus) {
//When updating activation status do not change any other data bytes
// Reach the offset in file where we have activation status
int offset = 0;
offset += g_DataOffset;
offset += 16;
offset += (16 * version);
offset += 12;
WriteWordToByteArray((short) activationStatus.getOrdinal(activationStatus), dataPtr, offset);
offset += 2;
ByteRef dataPtr1 = new ByteRef();
dataPtr1.data = dataPtr;
NCCrypto.EncryptDecryptBytes(dataPtr1, (int) (dwSize - g_DataOffset), g_DataOffset);
}
public static void GetInstallTime(byte[] data, long version, SystemTime pSysTime) throws com.alachisoft.ncache.runtime.exceptions.LicensingException {
int offset = 0;
if (data != null) {
offset += g_DataOffset + (16 * version);
pSysTime.wYear = ReadWordFromByteArray(data, offset);
offset += 2;
pSysTime.wMonth = ReadWordFromByteArray(data, offset);
offset += 2;
pSysTime.wMonth -= 1; //Java Calculates Month on zero based index so; Jan = 0,....Dec = 11
pSysTime.wDayOfWeek = ReadWordFromByteArray(data, offset);
offset += 2;
pSysTime.wDay = ReadWordFromByteArray(data, offset);
offset += 2;
pSysTime.wHour = ReadWordFromByteArray(data, offset);
offset += 2;
pSysTime.wMinute = ReadWordFromByteArray(data, offset);
offset += 2;
pSysTime.wSecond = ReadWordFromByteArray(data, offset);
offset += 2;
}
}
public static boolean IsValidVersionMark(byte[] data, int version) throws com.alachisoft.ncache.runtime.exceptions.LicensingException {
int offset = 0;
offset += g_DataOffset;
offset += 16;
offset += (version * 16);
SystemTime pSysTime = new SystemTime();
pSysTime.wYear = ReadWordFromByteArray(data, offset);
offset += 2;
pSysTime.wMonth = ReadWordFromByteArray(data, offset);
offset += 2;
pSysTime.wDay = ReadWordFromByteArray(data, offset);
offset += 2;
pSysTime.wMinute = ReadWordFromByteArray(data, offset);
offset += 2;
if (pSysTime.wYear < 2005 || pSysTime.wYear > 3500) {
return false;
}
if (pSysTime.wMonth > 12 || pSysTime.wDay > 31) {
return false;
}
return true;
}
// Verifies if the version timestamp is valid, i.e., installed!
public static boolean IsValidVersionMark(SystemTime pSysTime) {
if (pSysTime.wYear < 2005 || pSysTime.wYear > 3500) {
return false;
}
if (pSysTime.wMonth > 12 || pSysTime.wDay > 31) {
return false;
}
return true;
}
/**
* Convert the byte array to an int starting from the given offset.
*
* @param b The byte array
* @param offset The array offset
* @return The integer
*/
public static int ReadIntFromByteArray(byte[] b, int offset) {
int value = 0;
for (int i = 0; i < 4; i++) {
int shift = (4 - 1 - i) * 8;
value += (b[i + offset] & 0x000000FF) << shift;
}
return value;
}
public static short ReadWordFromByteArray(byte[] b, int offset) throws com.alachisoft.ncache.runtime.exceptions.LicensingException {
short shortVal = 0;
try {
ByteBuffer bb = ByteBuffer.allocate(2);
bb.order(ByteOrder.LITTLE_ENDIAN);
bb.put(b[offset]);
bb.put(b[offset + 1]);
shortVal = bb.getShort(0);
} catch (Exception ex) {
throw new LicensingException("Unable to find file containing evaluation data");
}
return shortVal;
}
public static byte[] intToByteArray(int value) {
byte[] b = new byte[4];
for (int i = 0; i < 4; i++) {
int offset = (b.length - 1 - i) * 8;
b[i] = (byte) ((value >>> offset) & 0xFF);
}
return b;
}
public static void WriteIntToByteArray(int value, byte[] data, int arrOffset) {
for (int i = 0; i < 4; i++, arrOffset++) {
int offset = (4 - 1 - i) * 8;
data[arrOffset] = (byte) ((value >>> offset) & 0xFF);
}
}
public static void WriteWordToByteArray(short value, byte[] data, int arrOffset) {
for (int i = 0; i < 2; i++, arrOffset++) {
int offset = (2 - 1 - i) * 8;
data[arrOffset] = (byte) ((value >>> offset) & 0xFF);
}
}
public static byte[] ReadEvaluationData() throws LicensingException {
String systemFolder[] = null;
RuntimeUtil.OS currentOS = RuntimeUtil.getCurrentOS();
try {
systemFolder = getStubPath();
} catch (Exception ex) {
throw new LicensingException("Unable to find file containing evaluation data");
}
ByteRef data = null;
for (int i = 0; i < systemFolder.length; i++) {
int dwSize = 0;
boolean fileExists = NCFileUtil.FileExists(systemFolder[i]);
if (fileExists) {
data = NCFileUtil.ReadFile(systemFolder[i], false);
if (data == null) {
throw new LicensingException("Unable to read file containing evaluation data");
}
if (data.data == null) {
throw new LicensingException("Unable to read file containing evaluation data");
}
return data.data;
}
}
return null;
}
public static int ReadEvaluationData(long version, SystemTime pSysTime) throws LicensingException, LocalVerificationFailedException {
String systemFolder[] = getStubPath();
ByteRef data = ReadEvaluationData(systemFolder);
return ReadEvaluationData(data.data, version, pSysTime);
}
public static int ReadEvaluationData(byte[] evalData, long version, SystemTime pSysTime) throws LicensingException {
NCMisc.GetInstallTime(evalData, version, pSysTime);
if (!NCMisc.IsValidVersionMark(pSysTime)) {
throw new LicensingException("Valid evaluation data not found. Version mark missing");
}
try {
RegUtil.LoadRegistry();
AppUtil app = new AppUtil();
pSysTime.wHour = (short) app.getExtensionsUsed();
} catch (java.util.MissingResourceException mre) {
pSysTime.wHour = 0;
}
return 0;
}
public static ByteRef ReadEvaluationData(String[] systemFolder) throws LicensingException, LocalVerificationFailedException {
ByteRef data = null;
for (int i = 0; i < systemFolder.length; i++) {
int dwSize = 0;
boolean fileExists = NCFileUtil.FileExists(systemFolder[i]);
if (fileExists) {
data = NCFileUtil.ReadFile(systemFolder[i]);
if (data == null) {
throw new LicensingException("Invalid Authentication Code: Stage 007");
}
if (data.data == null) {
throw new LicensingException("Invalid Authentication Code: Stage 008");
}
break;
} else {
throw new LocalVerificationFailedException("Failed to load info, contacting local server.");
}
}
return data;
}
public static String[] getStubPath() throws LicensingException {
String systemFolder[] = null;
RuntimeUtil.OS currentOS = RuntimeUtil.getCurrentOS();
try {
if (currentOS == RuntimeUtil.OS.Windows) {
String sys32Folder = System.getenv("WINDIR") + "\\system32";
systemFolder = new String[]{sys32Folder};
for (int i = 0; i < systemFolder.length; i++) {
systemFolder[i] += "\\" + winStub;
}
} else {
systemFolder = new String[]
{
"//usr//lib//" + linuxStub,
"//usr//bin//" + linuxStub,
};
}
} catch (Exception ex) {
throw new LicensingException("Unable to find file containing evaluation data");
}
return systemFolder;
}
public static void ReadActivationCode(StringRef dectext) {
try {
RegUtil.LoadRegistry();
StringRef authCode = new StringRef();
authCode.strData = RegUtil.getKey(InfoType.LICENSE, RegKeys.AuthCode);
String dec = NCCrypto.EDecode(authCode);
dectext.strData = dec;
return;
} catch (java.util.MissingResourceException mre) {
throw mre;
}
}
private static String[] getWinSystemDir(String delim, String prop) {
StringTokenizer tokanizer = new StringTokenizer(prop);
Vector v = new Vector();
String token = "";
while (tokanizer.hasMoreTokens()) {
token = tokanizer.nextToken(";");
if (token.indexOf(delim) != -1) {
if (!v.contains(token)) {
v.add(token);
}
}
}
return (String[]) v.toArray(new String[0]);
}
public boolean allowEvalVerification() {
try {
String paths[] = getStubPath();
} catch (LicensingException ex) {
}
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy